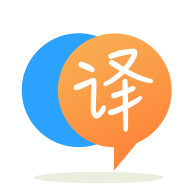
[英]close the opened accordion menu when second accordion menu is clicked, bootstrap
[英]How to.close accordion when clicked for the second time?
import React, { useState } from 'react';
const Accordion = ({ items }) => {
const [activeIndex, setActiveIndex] = useState(null);
const onTitleClick = (index) => {
setActiveIndex(index);
};
const renderedItems = items.map((item, index) => {
const active = index === activeIndex ? 'active' : '';
return (
<React.Fragment key={item.title}>
<div className={`title ${active}`} onClick={() => onTitleClick(index)}>
<i className='dropdown icon'></i>
{item.title}
</div>
<div className={`content ${active}`}>
<p>{item.content}</p>
</div>
</React.Fragment>
);
});
return <div className='ui styled accordion'>{renderedItems}</div>;
};
export default Accordion;
我使用語義 UI 庫創建了一個手風琴。 我能夠將下拉菜單的類別設置為“活動”,因此我單擊的任何內容都會展開。
我正在嘗試對以下代碼實現“第二次點擊關閉”功能,因此我嘗試通過在 onTitleClick 函數下添加以下代碼來實現:
const onTitleClick = (index) => {
if (index === activeIndex) {
setActiveIndex(null); // in case 'clicked index' is the same as what's active, then configure it to "null" and rerender
}
setActiveIndex(index);
};
我的理解是每當狀態更新時,react 將再次運行其腳本,因此在這種特殊情況下,如果單擊同一索引,變量“active”應該返回空字符串。
出乎我的意料,當我第二次點擊它時什么也沒發生。
我嘗試在 if 語句中使用 console.log,它會顯示我第二次單擊了該項目。
請指教。
問題在這里:
const onTitleClick = (index) => {
if (index === activeIndex) {
setActiveIndex(null); // in case 'clicked index' is the same as what's active, then configure it to "null" and rerender
}
setActiveIndex(index); <-- here
}
這里發生的情況是, if
條件匹配,則它設置為setActiveIndex
null 但代碼將再次運行,因此它設置為setActiveIndex(index)
。 這就是為什么點擊不起作用。 你需要這樣做:
const onTitleClick = (index) => {
if (activeIndex === index) {
setActiveIndex(null);
} else {
setActiveIndex(index);
}
};
這是演示: https : //codesandbox.io/s/musing-poitras-bkiwh?file=/ src/App.js: 270-418
試試下面的方法,
const onTitleClick = (index) => {
setActiveIndex(activeIndex !== index ? index : null);
};
我已經嘗試過使用 React 類組件,所以成功了。
import React, { Component } from 'react';
class Accordion extends Component {
state = {
activeIndex: null
}
onTitleClick = (index) => event => {
const { activeIndex } = this.state;
this.setState({ activeIndex: activeIndex == index ? null : index })
};
render() {
const { activeIndex } = this.state;
const{items}=this.props;
return <div className='ui styled accordion'>
{
items.map((item, index) => {
const active = index === activeIndex ? 'active' : '';
return <React.Fragment key={item.title}>
<div className={`title ${active}`} onClick={this.onTitleClick(index)}>
<i className='dropdown icon'></i>
{item.title}
</div>
<div className={`content ${active}`}>
<p>{item.content}</p>
</div>
</React.Fragment>
})
}
</div>
}
}
export default Accordion;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.