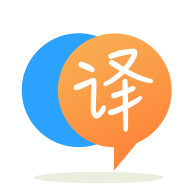
[英]How does parent component re-render a redux state which updated by its child modal?
[英]Redux | child component does NOT re-render parent component
代碼很長,但是思路是這樣的:
我有 3 個 Components Base App 組件,MapComponent,ShipComponent
App組件使用容器調用MapComponent,MapComponent也使用容器調用ShipComponent。 Map 和 Ship 集裝箱都連接到商店。 Map 和 Ship 組件使用相同的多數組數據並傳遞給mapStateToProps
在組件 Ship 中使用 useEffect() 調用一個動作來生成數據並將其放入商店中。
問題:
子組件(Ship 組件)重新呈現得很好,但組件 Map(父組件)甚至沒有,父組件 Map 也使用相同的 shipLocation 數組。 map 和 Ship 組件都是從同一個 redux 商店獲取的。
為什么執行動作后子組件會重新渲染,而父組件不會? 我如何解決它?
作為測試,我沒有將操作通過mapDispatchToProps
傳遞給 ShipComponent,而是將其傳遞給 MapComponent,然后通過 props 將其傳遞給 ShipComponent 以供其執行。 只有這樣它才會更新父 MapComponent。 但是我需要 ShipComponent 來獲取操作並更新 MapComponent。
編輯添加代碼示例:
所以根組件(App)
import React from "react";
import { Provider } from "react-redux";
import Map from "../containers/Map";
import mainStore from "../store";
const store = mainStore();
function AppComponent(props) {
return (
<Provider store={store}>
<Map />
</Provider>
);
}
export default AppComponent;
調用容器 Map 將所有 state 數據從存儲傳遞到組件 MapComponent
import { connect } from "react-redux";
import MapComponent from "../components/MapComponent";
const mapStateToProps = ({ shipR }) => {
return {
shipLocation: [...shipR.shipLocation],
};
};
const mapDispatchToProps = (dispatch) => {
return {
};
};
const Map = connect(mapStateToProps, mapDispatchToProps)(MapComponent);
export default Map;
地圖組件:
import React from "react";
import { Provider } from "react-redux";
import mainStore from "../store";
import Ship from "../containers/Ship";
const store = mainStore();
function MapComponent(props) {
return (
<div id="__Map__">
{
<Provider store={store}>
<Ship />
</Provider>
}
</div>
);
}
export default MapComponent;
這個 MapComponent 調用 ShipComponent 容器
import { connect } from "react-redux";
import ShipComponent from "../components/ShipComponent";
import { generateDefaultShips, shipToggle } from "../actions/shipAction";
const mapStateToProps = ({ shipR }) => {
return {
shipLocation: [...shipR.shipLocation],
};
};
const mapDispatchToProps = (dispatch) => {
return {
genShips() {
dispatch(generateDefaultShips());
},
};
};
const Ship = connect(mapStateToProps, mapDispatchToProps)(ShipComponent);
export default Ship;
ShipComponent 組件如下所示:
import React, { useEffect } from "react";
function ShipComponent(props) {
useEffect(() => {
props.genShips();
}, []);
return (
<div className="ship"></div>
);
}
export default ShipComponent;
genShips()
是一個動作:
import { SHIP_GENERATION_RESULT } from "../constants";
export function generateDefaultShips() {
let arr = [];
for (let x = 0; x < 7; x++) {
arr[x] = [];
for (let y = 0; y < 11; y++) arr[x][y] = null;
}
return { type: SHIP_GENERATION_RESULT, ships: arr };
}
MapComponent 減速器:
const initiateState = {
};
function mapReducer(state = initiateState, action) {
return state;
}
export default mapReducer;
和 ShipComponent 減速器:
import {
SHIP_GENERATION_RESULT,
} from "../constants";
const initiateState = {
shipLocation: [],
};
function shipReducer(state = initiateState, action) {
switch (action.type) {
case SHIP_GENERATION_RESULT: {
return {
...state,
shipLocation: action.ships,
};
}
default:
return state;
}
}
export default shipReducer;
和常量 index.js 包含: export const SHIP_GENERATION_RESULT = "SHIP_GENERATION_RESULT";
所有這些容器、常量、組件和操作都在不同的文件中
ps 子組件 ShipComponent 可以很好地獲取 shipLocation 多數組數據,但是由於 MapComponent(父級)不會重新渲染,所以他不會。
O 和 reduxers index.js:
import { combineReducers } from "redux";
import mapReducer from "./mapReducer";
import shipReducer from "./shipReducer";
const allReducers = combineReducers({
mapR: mapReducer,
shipR: shipReducer,
});
export default allReducers;
存儲 index.js:
import { createStore, applyMiddleware } from "redux";
import thunkMiddleware from "redux-thunk";
import allReducers from "../reducers";
import { composeWithDevTools } from "redux-devtools-extension";
export default function mainStore(prevState) {
return createStore(
allReducers,
prevState,
composeWithDevTools(applyMiddleware(thunkMiddleware))
);
}
您正在為 App 和 Map 組件使用不同的商店,刪除商店及其在 MapComponent 中的提供者應該有所幫助。 所有應用程序中應該只存儲 1 個 redux 以使所有數據保持一致。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.