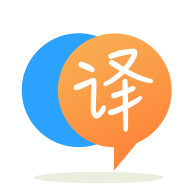
[英]How to assign task for each thread without forcefully using c I.e each thread need to take some work after completion of first work ?
[英]How to forcefully make one thread to be executed first in c
我在 c 中創建了兩個線程。 我想由每個線程執行兩個單獨的函數。 如何使一個特定的線程首先被執行。
#include <stdio.h>
#include <pthread.h>
void* function_1(void* p)
{
// statements
}
void* function_2(void* p)
{
// statements
}
int main(void)
{
pthread_t id1;
pthread_t id2;
pthread_create(&id1, NULL, function_1, NULL);
pthread_create(&id2, NULL, function_2, NULL);
pthread_exit(NULL);
}
程序啟動時如何使function_1在function_2之前執行?
將您的主要功能更改為如下所示:
int main(void) {
function_1(NULL);
function_2(NULL);
}
不是開玩笑! 如果function_2()
在function_1()
完成之前不啟動很重要,那么這就是這樣做的方法。 任何時候你需要一個程序以某種嚴格的順序做某些事情; 實現這一目標的最佳方法是在同一個線程中完成所有事情。
線程確實需要不時地彼此“同步”(例如,在生產者將某些東西放入隊列以供取用之前,消費者從隊列中取出某些東西沒有任何意義),但是如果您的線程大部分時間都沒有相互獨立工作,那么您可能不會從使用多個線程中獲得任何好處。
@SolomonSlow 已經說明了顯而易見的問題。 如果要順序執行,為什么要使用多個線程?
胸圍只是為了完整性:
pthread_create(&id1, NULL, function_1, NULL);
pthread_join(id1, NULL);
pthread_create(&id2, NULL, function_2, NULL);
如其他答案之一所述,您的問題可能是XY 問題。 在這種情況下最好不要使用多線程,而是使用單線程。
如果您確實想使用線程,那么正如其他答案之一中所述,使用pthread_join
可能是您在問題中發布的代碼的最佳解決方案。
但是,如果您只想確保function_2
中的代碼在另一個線程完成執行function_1
之前不被執行,並且不想等待執行function_1
的線程終止(例如,因為線程應該在之后執行其他操作)調用function_1
),然后我建議您使用 pthreads 條件變量和 pthreads mutex同步線程。
以下示例將代碼添加到function_1
的末尾,以通知另一個線程它現在可以繼續執行function_2
。 它還在function_2
的開頭添加了代碼,等待這個信號被發送。
這是示例:
#include <stdio.h>
#include <pthread.h>
struct sync_data
{
pthread_mutex_t mutex;
volatile int predicate;
pthread_cond_t cond;
};
void* function_1(void* p)
{
// the function's main code goes here
//signal to other thread that it may now proceed
struct sync_data *psd = p;
pthread_mutex_lock( &psd->mutex );
psd->predicate = 1;
pthread_mutex_unlock( &psd->mutex );
pthread_cond_signal( &psd->cond );
}
void* function_2(void* p)
{
//wait for signal from other thread
struct sync_data *psd = p;
pthread_mutex_lock( &psd->mutex );
while ( !psd->predicate )
pthread_cond_wait( &psd->cond, &psd->mutex );
pthread_mutex_unlock( &psd->mutex );
// the function's main code goes here
}
int main(void)
{
struct sync_data sd;
pthread_t id1;
pthread_t id2;
//init sync_data struct members
pthread_mutex_init( &sd.mutex, NULL );
sd.predicate = 0;
pthread_cond_init( &sd.cond, NULL );
//create and start both threads
pthread_create( &id1, NULL, function_1, &sd );
pthread_create( &id2, NULL, function_2, &sd );
//wait for all threads to finish
pthread_join(id1, NULL);
pthread_join(id2, NULL);
//cleanup
pthread_mutex_destroy( &sd.mutex );
pthread_cond_destroy( &sd.cond );
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.