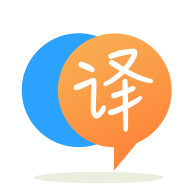
[英]How to create a widget for map function in flutter with buildContext
[英]How to put the result of a function into a Text widget in Flutter?
我是這門語言的新手,我正在開發 BMI(體重指數)應用程序。 如下圖所示:
我接受用戶輸入並計算結果,並在控制台中打印出結果。 例如:
I/flutter ( 4500): 2.25
I/flutter ( 4500): 20.0 // this is the result of BMI
I/flutter ( 4500): you are at your ideal weight. // this is the explanation
我想在文本小部件中顯示這些結果,讓用戶看到它們。 但我不知道該怎么做。 如何從函數中獲取結果的值並將其添加到接口?
這是我的代碼,在代碼中我指出了我卡在哪里。 主功能:
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'calculation.dart';
void main() => runApp(MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
static const String _title = 'BMI';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title),
centerTitle: true,
backgroundColor: Colors.amber[900],
),
body: Center(
child: MyStatefulWidget(),
),
),
);
}
}
enum SingingCharacter { lafayette, jefferson }
/// This is the stateful widget that the main application instantiates.
class MyStatefulWidget extends StatefulWidget {
MyStatefulWidget({Key key}) : super(key: key);
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
SingingCharacter _character = SingingCharacter.lafayette;
double height=1;
double weight=1;
String info1="";
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(10.0),
child:Scrollbar(
child:SingleChildScrollView(
child:Card(
color: Colors.amber[50],
child:Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.fromLTRB(0, 30, 10, 10),
child: Text("Sex:",
style:TextStyle(fontSize: 24, letterSpacing: 1.0)),
),
ListTile(
title: const Text('Female',
style:TextStyle(fontSize: 18, letterSpacing: 1.0)
),
leading: Radio(
activeColor: Colors.orange,
value: SingingCharacter.lafayette,
groupValue: _character,
onChanged: (SingingCharacter value) {
setState(() {
_character = value;
});
},
),
),
ListTile(
title: const Text('Male',
style:TextStyle(fontSize: 18, letterSpacing: 1.0,)
),
leading: Radio(
activeColor: Colors.orange,
value: SingingCharacter.jefferson,
groupValue: _character,
onChanged: (SingingCharacter value) {
setState(() {
_character = value;
});
},
),
),
SizedBox(height: 10.0),
Text("Your height:",
style:TextStyle(fontSize: 24, letterSpacing: 1.0)
),
SizedBox(height: 10),
Padding(
padding: const EdgeInsets.fromLTRB(30, 0, 50, 10),
child: TextField(
decoration: new InputDecoration(labelText: "Your height(cm)"),
keyboardType: TextInputType.number,
inputFormatters: <TextInputFormatter>[
FilteringTextInputFormatter.digitsOnly
], // Only numbers can be entered
onSubmitted: (input1){
if(double.parse(input1)>0){
setState(() => height=double.parse(input1));
print(input1);
}
},
),
),
SizedBox(height: 20),
Text("Your weight:",
style:TextStyle(fontSize: 24, letterSpacing: 1.0)
),
SizedBox(height: 10),
Padding(
padding: const EdgeInsets.fromLTRB(30, 0, 50, 10),
child: new TextField(
decoration: new InputDecoration(labelText: "Your weight(kg)"),
keyboardType: TextInputType.number,
inputFormatters: <TextInputFormatter>[
FilteringTextInputFormatter.digitsOnly
], // Only numbers can be entered
onSubmitted: (input2){
if (double.parse(input2)>0){
// print(weight);
setState(() {
return weight=double.parse(input2);
});
}
},
),),
SizedBox(height: 10,),
RaisedButton(
padding:EdgeInsets.fromLTRB(20, 5, 20, 5),
onPressed: () async{
await Calculation(height, weight);
// return Calculation.info1 ??? //i don't know how to take info1 from calculation function
},
color: Colors.amber[900],
child:Text(
'Calculate',
style:TextStyle(
color: Colors.white,
fontSize: 30,
letterSpacing: 2.0,
),
),
),
SizedBox(height: 20,),
Text('Results: $height,$weight'),
// Text('Calculation.info1'), // i do not know how to show info in a text box.
],
),
),
),
),
);
}
}
計算功能;
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'dart:math';
void Calculation(height,weight) {
double squarevalue = pow(height, 2);
double newsquare = squarevalue / 10000;
String info1="";
print(newsquare);
double value = weight / newsquare;
print(value);
// return value.toString();
if (value < 18.5) {
print("your weight is less than your ideal weight.");
// setState(() => info1="your weight is less than your ideal weight."); //i do not know how to set
// info1 to a new text
// return info1;
}
if (value > 25) {
if (value > 30) {
print("your weight is more than your ideal weight, your health is under risk.");
// info1="your weight is more than your ideal weight, your health is under risk.";
}
else {
print("your weight is more than your ideal weight.");
// info1="your weight is more than your ideal weight.";
}
}
else {
print("you are at your ideal weight.");
// info1="you are at your ideal weight.";
}
}
不是從 Calculation(height,weight) 函數返回 void,而是返回 String 並在 Text Widget 中顯示字符串值。
String Calculation(height,weight) {
....// your code with all conditions
return "you are at your ideal weight."
}
在onpressed函數中,將獲取到的字符串更新為setState內部的狀態變量。
onPressed: () async{
String info = await Calculation(height, weight);
setState(){
infoDisplayedInText = info;
}
},
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.