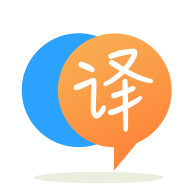
[英]Create a nested array of objects from flat object array with childIds
[英]Create a nested array of objects (up to 5 levels) from flat array of objects
我需要從最多 5 個級別的平面對象數組創建一個嵌套的對象數組。 原始 JSON 如下所示:
[{
"code": "01",
"name": "Some name 1",
"level": "1"
}, {
"code": "01.1",
"name": "Some name 2",
"level": "2"
}, {
"code": "01.11",
"name": "Some name 3",
"level": "3"
}, {
"code": "01.11.1",
"name": "Some name 4",
"level": "4"
}, {
"code": "01.11.11",
"name": "Some name 5",
"level": "5"
}, {
"code": "01.11.12",
"name": "Some name 6",
"level": "5"
}]
新數組將用於 Ant Design Tree組件,因此它應該具有以下結構:
[
{
key: '01',
title: 'Some name 1',
children: [
key: '01.1'
title: 'Some name 2',
children: [
{
key: '01.11'
title: 'Some name 3',
children: [
{
key: '01.11.1'
title: 'Some name 4',
children: [
{
key: '01.11.11'
title: 'Some name 5'
},
{
key: '01.11.12'
title: 'Some name 6'
}
]
}
]
}
]
]
}
]
我創建了一個用於嵌套數組的函數,但組件變得非常慢。 我想有更好的方法來做到這一點。 這是我的代碼:
const __getCodes = () => {
let array = codeData;
let result = [];
for(let i = 0; i < array.length; i++) {
let obj = array[i];
if (obj.level === "1") {
result.push({key: obj.code, title: obj.code + ' ' + obj.name, checkable: false});
}
if (obj.level === "2") {
const currentGroup = result.find(group => group.key === obj.code.substring(0,2))
if (!currentGroup.children) {
currentGroup.children = []
}
currentGroup.children.push({key: obj.code, title: obj.code + ' ' + obj.name, checkable: false})
}
if (obj.level === "3") {
const parentGroup = result.find(group => group.key === obj.code.substring(0,2))
const currentGroup = parentGroup.children.find(group => group.key === obj.code.substring(0,4))
if (!currentGroup.children) {
currentGroup.children = []
}
currentGroup.children.push({key: obj.code, title: obj.code + ' ' + obj.name})
}
if (obj.level === "4") {
const subParentGroup = result.find(group => group.key === obj.code.substring(0,2))
const parentGroup = subParentGroup.children.find(group => group.key === obj.code.substring(0,4))
const currentGroup = parentGroup.children.find(group => group.key === obj.code.substring(0,5))
if (!currentGroup.children) {
currentGroup.children = []
}
currentGroup.children.push({key: obj.code, title: obj.code + ' ' + obj.name})
}
if (obj.level === "5") {
const subSubParentGroup = result.find(group => group.key === obj.code.substring(0,2))
const subParentGroup = subSubParentGroup.children.find(group => group.key === obj.code.substring(0,4))
const parentGroup = subParentGroup.children.find(group => group.key === obj.code.substring(0,5))
const currentGroup = parentGroup.children.find(group => group.key === obj.code.substring(0,7))
if (!currentGroup.children) {
currentGroup.children = []
}
currentGroup.children.push({key: obj.code, title: obj.code + ' ' + obj.name})
}
}
return result;
}
const treeData = __getCodes();
如何優化此功能以獲得所需的結果?
您可以創建一個解決方案,該解決方案將使用level
屬性將當前對象推送到嵌套結構中的某個級別,並將其與引用和reduce
方法相結合。
const data = [{"code":"01","name":"Some name 1","level":"1"},{"code":"01.1","name":"Some name 2","level":"2"},{"code":"01.11","name":"Some name 3","level":"3"},{"code":"01.11.1","name":"Some name 4","level":"4"},{"code":"01.11.11","name":"Some name 5","level":"5"},{"code":"01.11.12","name":"Some name 6","level":"5"}] const result = data.reduce((r, { level, ...rest }) => { const value = { ...rest, children: [] } r[level] = value.children; r[level - 1].push(value) return r; }, [[]]).shift() console.log(result)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.