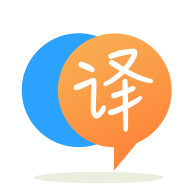
[英]Most efficient way to copy elements from multidimensional array to flat array?
[英]Efficient and faster way to find coprimes elements from an array
我正在做一個小項目。 我的任務是在數組中查找與所有其他值互質的元素。 我想出了一個使用雙 for 循環的方法。 但是,我相信時間復雜度不會很好,尤其是在元素數組很大的情況下。
這些是我所擁有的:
ArrayList<Integer> getAllCoPrimes(int[] inputs) {
ArrayList<Integer> coprimes = new ArrayList<>();
int[] elementsCount = new int[inputs.length];
for (int i = 0; i < inputs.length; i++) {
int x = inputs[i];
for (int j = 0; j < inputs.length; j++) {
int y = inputs[j];
if (i != j) {
if (gcd(x, y) == 1) {
elementsCount[i]++;
if (elementsCount[i] == inputs.length) //x is a coprime with all the elements in the array
coprimes.add(x);
}
}
}
}
return coprimes;
}
static int gcd(int a, int b) {
// Everything divides 0
if (a == 0 || b == 0)
return 0;
// base case
if (a == b)
return a;
// a is greater
if (a > b)
return gcd(a - b, b);
return gcd(a, b - a);
}
我想問一下有什么方法可以改進我的方法以更有效地運行嗎? 先感謝您 :)
您的算法目前是 O(n^2*log(S))。 n = 項目數,S = 數字的大小
我相信它可以在 O(n*n) 中實現(這不一定是很大的收益)。
注意:您可能對原始代碼沒問題...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.