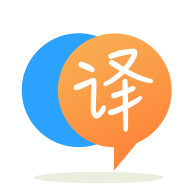
[英]How do I turn the following if statements into a more efficient nested for loops
[英]How am I able to make IF statements more efficient?
我創建了一個小程序,它接受用戶輸入的“飛行規范”,並根據輸入輸出該飛行的詳細信息。 然而,它只是一堆if語句,我想知道是否有任何有用的技術來減少if語句的數量並使程序更高效。
代碼:
import time
def main():
AMS_DESTINATION = "Schiphol, Amsterdam"
GLA_DESTINATION = "Glasgow, Scotland"
AMS_PRICE = 150.00
GLA_PRICE = 80.00
# User input [LLL 0 00 L L]
flightSpecification = str(input("Enter Flight Specification: "))
flightDestination = flightSpecification[0:3]
bagCount = int(flightSpecification[4])
baggageCost = float((bagCount - 1) * 20)
passengerAge = int(flightSpecification[6:8])
standardMeal = 10.00
vegetarianMeal = 12.00
mealType = str(flightSpecification[9])
seatClass = str(flightSpecification[11])
totalFlightCost = 0
if flightDestination.lower() != 'ams' and flightDestination.lower() != 'gla':
print("Please enter a valid flight specification! [LLL 0 00 L L]")
time.sleep(2)
main()
if flightDestination.lower() == 'ams':
print(f"Destination: {AMS_DESTINATION}")
print(f"Flight cost: £{AMS_PRICE}")
totalFlightCost = 150
elif flightDestination.lower() == 'gla':
print(f"Destination: {GLA_DESTINATION}")
print(f"Flight cost: £{GLA_PRICE}")
totalFlightCost = 80
print(f"Number of bags: {bagCount}")
print(f"Baggage Cost: £{baggageCost}")
if passengerAge > 15:
print("Child: False")
elif passengerAge <= 15:
print("Child: True")
totalFlightCost = totalFlightCost / 2
standardMeal = standardMeal - 2.50
vegetarianMeal = vegetarianMeal - 2.50
elif passengerAge < 0:
print("Age cannot be negative")
main()
if mealType == 'S' and seatClass != 'F':
totalFlightCost = totalFlightCost + standardMeal
print("Meal: Standard")
print(f"Meal Cost: £{standardMeal}")
elif mealType == 'V' and seatClass != 'F':
totalFlightCost = totalFlightCost + vegetarianMeal
print("Meal: Vegetarian")
print(f"Meal Cost: £{vegetarianMeal}")
elif mealType == 'N':
print("Meal: None")
print("Meal Cost: £0")
# THIS COULD DEFINITELY BE DONE MORE EFFICIENTLY
if seatClass == 'F':
if mealType == 'S':
totalFlightCost = totalFlightCost + standardMeal
print("Meal: Standard")
print(f"Meal Cost: FREE")
elif mealType == 'V':
totalFlightCost = totalFlightCost + vegetarianMeal
print("Meal: Vegetarian")
print(f"Meal Cost: £{vegetarianMeal}")
print("Seating Class: First")
totalFlightCost = totalFlightCost * 6
elif seatClass == 'E':
print("Seating Class: Economy")
print(totalFlightCost)
main()
謝謝
除了您問題下方的評論外,我在您提到的可以優化的部分更改了一個小東西(我還刪除了用戶輸入,因為它減慢了過程,最后您會明白):
import time
def main2():
AMS_DESTINATION = "Schiphol, Amsterdam"
GLA_DESTINATION = "Glasgow, Scotland"
AMS_PRICE = 150.00
GLA_PRICE = 80.00
# User input [LLL 0 00 L L]
# flightSpecification = str(input("Enter Flight Specification: "))
flightSpecification = 'AMS 1 22 V F'
flightDestination = flightSpecification[0:3]
bagCount = int(flightSpecification[4])
baggageCost = float((bagCount - 1) * 20)
passengerAge = int(flightSpecification[6:8])
standardMeal = 10.00
vegetarianMeal = 12.00
mealType = str(flightSpecification[9])
seatClass = str(flightSpecification[11])
totalFlightCost = 0
if flightDestination.lower() != 'ams' and flightDestination.lower() != 'gla':
print("Please enter a valid flight specification! [LLL 0 00 L L]")
time.sleep(2)
main()
if flightDestination.lower() == 'ams':
print(f"Destination: {AMS_DESTINATION}")
print(f"Flight cost: £{AMS_PRICE}")
totalFlightCost = 150
elif flightDestination.lower() == 'gla':
print(f"Destination: {GLA_DESTINATION}")
print(f"Flight cost: £{GLA_PRICE}")
totalFlightCost = 80
print(f"Number of bags: {bagCount}")
print(f"Baggage Cost: £{baggageCost}")
if passengerAge > 15:
print("Child: False")
elif passengerAge <= 15:
print("Child: True")
totalFlightCost = totalFlightCost / 2
standardMeal = standardMeal - 2.50
vegetarianMeal = vegetarianMeal - 2.50
elif passengerAge < 0:
print("Age cannot be negative")
main()
if mealType == 'S' and seatClass != 'F':
totalFlightCost = totalFlightCost + standardMeal
print("Meal: Standard")
print(f"Meal Cost: £{standardMeal}")
elif mealType == 'V' and seatClass != 'F':
totalFlightCost = totalFlightCost + vegetarianMeal
print("Meal: Vegetarian")
print(f"Meal Cost: £{vegetarianMeal}")
elif mealType == 'N':
print("Meal: None")
print("Meal Cost: £0")
# THIS COULD DEFINITELY BE DONE MORE EFFICIENTLY
if seatClass == 'F' and mealType is 'S':
totalFlightCost = totalFlightCost + standardMeal
print("Meal: Standard")
print(f"Meal Cost: FREE")
print("Seating Class: First")
totalFlightCost = totalFlightCost * 6
elif seatClass is 'F' and mealType == 'V':
totalFlightCost = totalFlightCost + vegetarianMeal
print("Meal: Vegetarian")
print(f"Meal Cost: £{vegetarianMeal}")
print("Seating Class: First")
totalFlightCost = totalFlightCost * 6
elif seatClass == 'E':
print("Seating Class: Economy")
print(totalFlightCost)
更改 main 中的輸入,您將在下面的測試中認識到沒有真正的性能問題:
start = time.time()
main()
print('main() takes: ')
print(time.time() - start)
start = time.time()
main2()
print('main2() takes:')
print(time.time() - start)
main() 需要:7.319450378417969e-05 秒
main2() 需要:4.935264587402344e-05 秒
在您設置的遞歸路徑中(在評論中提到),您還使用 time.sleep(2)。 插入硬編碼睡眠總是會影響你的表現。
對於這樣一個簡單的程序,它根本不值得考慮效率。 然而,展望未來,如果您要驗證具有數十萬或更多項目的一批數據,那么效率就會成為一個問題。
以下是一些需要考慮的要點:
# flightSpecification = str(input("Enter Flight Specification: "))
flightSpecification = input("Enter Flight Specification: ")
注意input()
總是返回一個str
,所以它周圍的str()
是多余的。
# mealType = str(flightSpecification[9])
mealType = flightSpecification[9]
類似地,由於flightSpecification
已經是str
,因此str()
是多余的。
#if flightDestination.lower() == 'ams':
flightDestinationLower = flightDestination.lower()
if flightDestinationLower == 'ams':
由於您進行了類似的比較,因此執行lower()
一次可能比多次執行更有效。
flightDestinationLower = flightDestination.lower()
if flightDestinationLower == 'ams':
print(f"Destination: {AMS_DESTINATION}")
print(f"Flight cost: £{AMS_PRICE}")
totalFlightCost = 150
elif flightDestinationLower == 'gla':
print(f"Destination: {GLA_DESTINATION}")
print(f"Flight cost: £{GLA_PRICE}")
totalFlightCost = 80
else:
print("Please enter a valid flight specification! [LLL 0 00 L L]")
time.sleep(2)
#main()
continue
重新排列if
的順序,以便else:
不需要進行比較。 也不要在您打算使用迭代的地方使用遞歸。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.