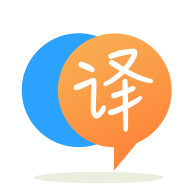
[英]Pass data from child to parent and to another child component React Js
[英]how to pass an event from a child component to parent component back down to another child in React
例如,我在 React 中有三個組件,一個應用程序(父組件),一個按鈕組件和一個用於顯示某些內容的組件,可以是任何東西都無關緊要。 讓我們說在按鈕組件被激活時,我如何將信息(即事件實際發生)傳遞給 App 父組件回傳給另一個子組件,以讓它知道發生了特定事件來顯示一些消息?
這就是我將如何使用鈎子解決這個問題:
const Parent=(props)=>{
[eventHappend,setEventHappend]=useState(false)
return (
<div>
<Child1 setEventHappend={setEventHappend} />
<Child2 eventHappend={eventHappend} />
</div>
)
}
const Child =({setEventHappend})=>{
return (
<div>
<button onClick={e=>setEventHappend(true)} > click me 1 </button>
</div>
)
}
const Child2 =({eventHappend})=>{
return (
<div>
<button onClick={e=>{/* some code*/ }} > {eventHappend?'event happend':'event didnt happen yet '} </button>
</div>
)
}
有多種方法可以實現此傳遞狀態作為子元素的道具(必須在其他方法之前知道)、上下文或使用具有存儲的 redux。
通常來說,一般來說。 React 有一種單向的數據流方式。 因為在父元素中將保持狀態並將傳遞給子元素。
這里 App 持有狀態 buttonClick ,其中包含有關事件的信息。
const App = () => {
const [ buttonClick, setButtonClick] = React.useState(false);
const messageToBeDispalyed = "The button has been clicked"
return (
<div>
<CustomButton setEventHappened={setButtonClick} />
<DisplayText value = {buttonClick ? messageToBeDispalyed : ""} />
</div>
)
}
const CustomButton = (props) =>{
return <button onClick={(e)=>props.setEventHappened(true)} > Click Me </button>
}
const DisplayText = (props) => {
return <h1> {props.value} </h1>
}
與其他答案類似,但您會將方法從父級傳遞給子級以更新狀態。 但請注意,這樣做會導致對所有父母的孩子進行重新渲染。
const Parent = () => {
const [state, setState] = React.useState(false);
const handleClick = value => {
setState(value);
};
return (
<Child state={state} handleClick={handleClick} />
<OtherChild isTrue={state} /> // this component needs data changed by <Child />
)
};
const Child = props => {
const {state, handleClick} = props;
return (
<button onClick={() => handleClick(!state)} >click me</button>
);
};
通過這種方式,父級單獨處理狀態更改並將該方法提供給子級。
正如@Loveen Dyall 和@Shruti B 提到的,您可以將 RXJS 用於更模塊化的方法,雖然 RxJS 通常被認為用於 Angular 項目,但它是一個完全獨立的庫,可以與其他 JavaScript 框架(如 React 和 Vue)一起使用。
在 React 中使用 RxJS 時,組件之間通信的方式是使用一個 Observable 和一個 Subject(這是一種 observable),我不會過多地介紹 observables 如何工作的細節,因為它是一個很大的主題,但簡而言之,我們對兩種方法感興趣:Observable.subscribe() 和 Subject.next()。 了解有關 RXJS 和 Observables 的更多信息: https ://blog.logrocket.com/understanding-rxjs-observables/
Observable.subscribe() React 組件使用 observable 訂閱方法來訂閱發送到 observable 的消息。
Subject.next() 主題 next 方法用於將消息發送到 observable,然后將消息發送到所有 React 組件,這些組件是該 observable 的訂閱者(也稱為觀察者)。
import { eventsService} from '../services';
const Child1 =()=>{
const [child2EventFired,setChild2EventFired]=useState(false)
useEffect(()=>{
let subscription = eventsService.getEventNotification().subscribe(eventTitle =>
{
if (eventTitle=="CHILD2_BUTTON_CLICK" ) {
setChild2EventFired(true)
}else{
setChild2EventFired(false)
}
});
return ()=>{
subscription.unsubscribe();
}
},[])
return <div>
<button> {child2EventFired? 'child2 button event fired':'event not fired yet'} </button>
</div>
}
在您的 Child 1 組件中,如果您使用類組件,您將訂閱 useEffect 或 compoentDidMount 中的 observable:
import { eventsService} from '../services';
const Child2 =()=>{
Child2Click=(e)=>{
//some code,
//then send messages to the observable observable
eventsService.sendEvent('CHILD2_BUTTON_CLICK');
}
return <div>
<button onClick={Child2Click} >click me</button>
</div>
}
在您的 Child 2 組件中
import { eventsService} from '../services'; const Child2 =()=>{ Child2Click=(e)=>{ //some code, //then send messages to the observable observable eventsService.sendEvent('CHILD2_BUTTON_CLICK'); } return <div> <button onClick={Child2Click} >click me</button> </div> }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.