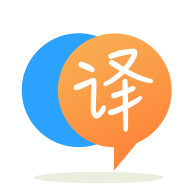
[英]how to find the prime factors of a number?spent hours trying to figure out why both the if and else are executed simultaneously
[英]Trying to figure out how to find the final distance on my turtle with random number
這是我的代碼,我試圖弄清楚如何獲得我的烏龜走過的整個長度的總距離,但我不知道如何在不將其從我無法做到的循環中取出的情況下計算它因為 numsteps 是一個輸入。 順便說一下,這是給學校的
for a in range(numsteps):
s = randint(-100,100)
angle = random() * 2 * pi
x = s * cos(angle)
y = s * sin(angle)
walking.goto(x,y)
distance = sqrt(x ** 2 + y ** 2)
finald.goto(x,y)
print("The final distance is {:,.0f}".format(distance))
print("Your total distance traveled is {}")
您必須保存之前的位置以計算從當前位置到它的距離。
然后每次計算距離后(接近循環末尾),當前位置變為前一個:
from math import sin, cos, pi, sqrt
from random import random, randint
start_x, start_y = 0, 0 # The starting point coordinates
previous_x, previous_y = start_x, start_y
total_distance = 0 # We will progressively increase it
numsteps = int(input("Number of steps: "))
for __ in range(numsteps):
s = randint(-100, 100)
angle = random() * 2 * pi
x = s * cos(angle)
y = s * sin(angle)
# walking.goto(x, y) # I commented it out for testing
distance = sqrt((x - previous_x) ** 2 + (y - previous_y) ** 2)
total_distance += distance
prev_x, prev_y = x, y
final_distance = sqrt((x - start_x) ** 2 + (y - start_y) ** 2)
print("The final distance is {:,.0f}".format(final_distance))
print("Your total distance traveled is {:,.0f}".format(total_distance))
請注意__
而不是您的a
(或其他常規名稱)——這個特殊名稱(一或兩個下划線字符)表明它的值不符合我們的興趣。
(因為我們只使用range(numsteps)
作為計數器。)
我會根據一些觀察進行簡化:
Turtle 已經知道距離函數了,不需要重新發明
當我們可以向任何方向前進時,向后移動(負距離)是多余的——這與向任何方向向前移動沒有什么不同。
我們可以更容易地計算出我們移動了多遠,而不是衡量我們將移動多遠。
這導致代碼更像:
from math import pi
from random import random
from turtle import Screen, Turtle
numsteps = int(input("Number of steps: "))
screen = Screen()
walking = Turtle()
walking.radians()
total_distance = 0 # sum of all distances traveled
for _ in range(numsteps):
previous = walking.position()
angle = random() * 2 * pi
distance = random() * 100
walking.setheading(angle)
walking.forward(distance)
total_distance += walking.distance(previous)
final_distance = walking.distance(0, 0) # distance from where we started
print("Your final distance traveled is {:,.0f} pixels".format(final_distance))
print("Your total distance traveled is {:,.0f} pixels".format(total_distance))
screen.exitonclick()
輸出
> python3 test.py
Number of steps: 100
Your final distance traveled is 356 pixels
Your total distance traveled is 4,630 pixels
>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.