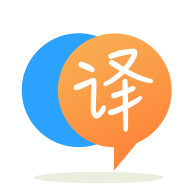
[英]Single digit displaying after entering double digit as input in java Program
[英]Take 6 digit input from user and store each digit in a single array in Java
我想使用掃描儀讀取 6 位數字,例如 123456,並將每個數字存儲在數字數組中。
System.out.println("Please enter a 6 digit code");
int code = keyboard.nextInt();
然后以某種方式將其放入:
int [] array = {1,2,3,4,5,6};
我需要數組為 int 類型,因為當我將序列中的奇數 (1+3+5) 相加並添加偶數 (2+4+6)(根據程序)然后我有一些條件告訴我要連接到最后的數字。
我可以通過預先定義一組數字來實現這一點,但我希望這個數字來自用戶。
除非有另一種不使用數組的方法? 我很感激任何指點。
這是我預定義數組及其值時的代碼。
int code[] = {1,2,3,4,5,6};
//add the numbers in odd positions together
int sum_odd_position = 0;
for (int i=0; i<6; i+=2)
{
sum_odd_position += code[i];
}
//convert the initial code into strings
String string_code = "";
String seven_digit_code = "";
//add numbers in even positions together
int sum_even_position=0;
for (int i=1; i<6; i+=2)
{
sum_even_position += code [i];
}
//add them both together
int sum_odd_plus_even = sum_odd_position + sum_even_position;
//calculate remainder by doing our answer mod 10
int remainder = sum_odd_plus_even % 10;
System.out.println("remainder = "+remainder);
//append digit onto result
if (remainder==0) {
//add a 0 onto the end
for (int i=0; i<6; i+=2)
{
string_code += code [i];
}
}
else {
//subtract remainder from 10 to derive the check digit
int check_digit = (10 - remainder);
//concatenate digits of array
for (int i=0; i<6; i++)
{
string_code += code[i];
}
//append check digit to string code
}
seven_digit_code = string_code + remainder;
System.out.println(seven_digit_code);
}
}
首先從用戶讀入一個字符串。 創建一個數組來放入數字。 逐個字符地遍歷字符串。 將每個字符轉換為適當的 int 值並將其放入數組中。
String line = keyboard.nextLine();
int[] digits = new int[line.length()];
for (int i = 0; i < digits.length; ++i) {
char ch = line.charAt(i);
if (ch < '0' || ch > '9') {
throw new IllegalArgumentException("You were supposed to input decimal digits.");
}
digits[i] = ch - '0';
}
(另請注意,如果ch-'0'
不適合您的目的,則有多種方法可以將 char 解析為 int。)
向用戶發送消息:
System.out.println("Hello, please enter a 6 digit number: ");
要要求輸入,請制作一次掃描儀並設置分隔符:
// do this once for the entire app
Scanner s = new Scanner(System.in);
s.useDelimiter("\r?\n");
然后索取東西:
int nextInt = s.nextInt();
String nextString = s.next();
選擇您想要的任何數據類型,並nextX()
調用正確的nextX()
方法。
這聽起來你應該要求一個字符串而不是一個數字(因為大概000000
是有效的輸入,但這不是一個真正的整數),所以你會使用next()
。
要檢查它是否為 6 位數字,您可以使用多種方法。 據推測,無論如何您都需要將其轉換為數組,那么為什么不循環遍歷每個字符:
String in = s.next();
if (in.length() != 6) throw new IllegalArgumentException("6 digits required");
int[] digits = new int[6];
for (int i = 0; i < 6; i++) {
char c = in.charAt(i); // get character at position i
int digit = Character.digit(c, 10); // turn into digit
if (digit == -1) throw new IllegalArgumentException("digit required at pos " + i);
digits[i] = digit;
}
如果你想不那么以英語/西方為中心,或者你想添加對十六進制數字的支持,你可以使用 Character 中的一些實用方法:
if (digit == -1) throw new IllegalArgumentException("digit required");
首先,為數字分配一個掃描儀和一個數組
Scanner input = new Scanner(System.in);
int[] digits = new int[6];
Math.log10
檢查正確的位數for (;;) {
System.out.print("Please enter six digit integer: ");
int val = input.nextInt();
// a little math. Get the exponent of the number
// and assign to an int. This will determine the number of digits.
if ((int) Math.log10(val) != 5) {
System.out
.println(val + " is not six digits in size.");
continue;
}
for (int i = 5; i >= 0; i--) {
digits[i] = val % 10;
val /= 10;
}
break;
}
System.out.println(Arrays.toString(digits));
用於輸入123456
照片
[1, 2, 3, 4, 5, 6]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.