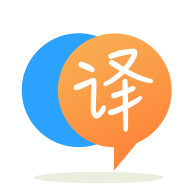
[英]How to add an indicator of certain value on x-axis in plotly candlestick chart
[英]Plotly: How to add volume to a candlestick chart
代碼:
from plotly.offline import init_notebook_mode, iplot, iplot_mpl
def plot_train_test(train, test, date_split):
data = [Candlestick(x=train.index, open=train['open'], high=train['high'], low=train['low'], close=train['close'],name='train'),
Candlestick(x=test.index, open=test['open'], high=test['high'], low=test['low'], close=test['close'],name='test')
]
layout = {
'shapes': [
{'x0': date_split, 'x1': date_split, 'y0': 0, 'y1': 1, 'xref': 'x', 'yref': 'paper',
'line': {'color': 'rgb(0,0,0)', 'width': 1}}],
'annotations': [{'x': date_split, 'y': 1.0, 'xref': 'x', 'yref': 'paper', 'showarrow': False, 'xanchor': 'left','text': ' test data'},
{'x': date_split, 'y': 1.0, 'xref': 'x', 'yref': 'paper', 'showarrow': False, 'xanchor': 'right', 'text': 'train data '}] }
figure = Figure(data=data, layout=layout)
iplot(figure)
上面的代碼沒問題。但現在我想在此燭台圖表中“交易量”
代碼:
from plotly.offline import init_notebook_mode, iplot, iplot_mpl
def plot_train_test(train, test, date_split):
data = [Candlestick(x=train.index, open=train['open'], high=train['high'], low=train['low'], close=train['close'],volume=train['volume'],name='train'),
Candlestick(x=test.index, open=test['open'], high=test['high'], low=test['low'],close=test['close'],volume=test['volume'],name='test')]
layout = {
'shapes': [
{'x0': date_split, 'x1': date_split, 'y0': 0, 'y1': 1, 'xref': 'x', 'yref': 'paper',
'line': {'color': 'rgb(0,0,0)', 'width': 1}}
],
'annotations': [
{'x': date_split, 'y': 1.0, 'xref': 'x', 'yref': 'paper', 'showarrow': False, 'xanchor': 'left',
'text': ' test data'},
{'x': date_split, 'y': 1.0, 'xref': 'x', 'yref': 'paper', 'showarrow': False, 'xanchor': 'right',
'text': 'train data '}
]
}
figure = Figure(data=data, layout=layout)
iplot(figure)
錯誤:
ValueError:為 plotly.graph_objs.Candlestick 類型的 object 指定的無效屬性:'volume'
您還沒有提供一個完整的代碼段與數據樣本,所以我不得不認為,建立在一個示例的解決方案在這里。
在任何情況下,您都會收到該錯誤消息,因為go.Candlestick
沒有Volume
屬性。 乍一看似乎並非如此,但您可以輕松地將go.Candlestick
設置為單獨的跟蹤,然后使用以下方法為 Volumes 包含單獨的go.Bar()
跟蹤:
fig = make_subplots(specs=[[{"secondary_y": True}]])
fig.add_traces(go.Candlestick(...), secondary_y=True)
fig.add_traces(go.Bar(...), secondary_y=False)
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import pandas as pd
# data
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/finance-charts-apple.csv')
# Create figure with secondary y-axis
fig = make_subplots(specs=[[{"secondary_y": True}]])
# include candlestick with rangeselector
fig.add_trace(go.Candlestick(x=df['Date'],
open=df['AAPL.Open'], high=df['AAPL.High'],
low=df['AAPL.Low'], close=df['AAPL.Close']),
secondary_y=True)
# include a go.Bar trace for volumes
fig.add_trace(go.Bar(x=df['Date'], y=df['AAPL.Volume']),
secondary_y=False)
fig.layout.yaxis2.showgrid=False
fig.show()
如果您想在 OHLC 圖表下方添加較小的體積子圖,您可以使用:
rows
和cols
指定次要情節電網。shared_xaxes=True
用於相同的縮放和過濾row_width=[0.2, 0.7]
改變圖表的高度比例。 IE。 體積圖比 OHLC 小import pandas as pd
import plotly.graph_objects as go
from plotly.subplots import make_subplots
# data
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/finance-charts-apple.csv')
# Create subplots and mention plot grid size
fig = make_subplots(rows=2, cols=1, shared_xaxes=True,
vertical_spacing=0.03, subplot_titles=('OHLC', 'Volume'),
row_width=[0.2, 0.7])
# Plot OHLC on 1st row
fig.add_trace(go.Candlestick(x=df["Date"], open=df["AAPL.Open"], high=df["AAPL.High"],
low=df["AAPL.Low"], close=df["AAPL.Close"], name="OHLC"),
row=1, col=1
)
# Bar trace for volumes on 2nd row without legend
fig.add_trace(go.Bar(x=df['Date'], y=df['AAPL.Volume'], showlegend=False), row=2, col=1)
# Do not show OHLC's rangeslider plot
fig.update(layout_xaxis_rangeslider_visible=False)
fig.show()
這是我根據 Vestland 之前的回答進行的改進實施,其中包含一些標簽和着色改進。
import plotly.graph_objects as go
from plotly.subplots import make_subplots
candlesticks = go.Candlestick(
x=candles.index,
open=candles['open'],
high=candles['high'],
low=candles['low'],
close=candles['close'],
showlegend=False
)
volume_bars = go.Bar(
x=candles.index,
y=candles['volume'],
showlegend=False,
marker={
"color": "rgba(128,128,128,0.5)",
}
)
fig = go.Figure(candlesticks)
fig = make_subplots(specs=[[{"secondary_y": True}]])
fig.add_trace(candlesticks, secondary_y=True)
fig.add_trace(volume_bars, secondary_y=False)
fig.update_layout(title="ETH/USDC pool after Uniswap v3 deployment", height=800)
fig.update_yaxes(title="Price $", secondary_y=True, showgrid=True)
fig.update_yaxes(title="Volume $", secondary_y=False, showgrid=False)
fig.show()
如果您想為買/賣添加不同的顏色,例如“綠色”/“紅色”,您可以使用一些庫(例如 mplfinance)自動執行這些操作,但是這些圖是非交互式的。 要獲得具有不同顏色的買/賣顏色的交互式繪圖,需要為每個數據點添加跟蹤。 這是代碼:
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import pandas as pd
# Create subplots and mention plot grid size
title=df.symbol.unique()[0]
fig = make_subplots(rows=2, cols=1, shared_xaxes=True,
vertical_spacing=0.02,
row_width=[0.25, 0.75])
# Plot OHLC on 1st row
fig.add_trace(go.Candlestick(x=df.index,
open=df['open'], high=df['high'],
low=df['low'], close=df['close'],showlegend=False),row=1, col=1,)
# Bar trace for volumes on 2nd row without legend
# fig.add_trace(go.Bar(x=df.index, y=df['volume'], showlegend=False), row=2, col=1)
df['color']=''
df['color']=['red' if (x>y) else t for x,y,t in zip(df['open'],df['close'],df['color'])]
df['color']=['green' if (x<y) else t for x,y,t in zip(df['open'],df['close'],df['color'])]
colors=df.color.tolist()
df['prev_color']=[colors[0]]+colors[:(len(colors)-1)]
df.loc[((df.open==df.close) & (df.color=='')),'color']=[z for x,y,z,t in zip(df['open'],df['close'],df['prev_color'],df['color']) if (x==y and t=='')]
colors=df.color.tolist()
df['prev_color']=[colors[0]]+colors[:(len(colors)-1)]
df.loc[((df.open==df.close) & (df.color=='')),'color']=[z for x,y,z,t in zip(df['open'],df['close'],df['prev_color'],df['color']) if (x==y and t=='')]
markers=['green','red']
for t in markers:
df_tmp=df.loc[~(df.color==t)] ## somehow the color it takes is opposite so take negation to
fig.add_trace(go.Bar(x=df_tmp.index, y=df_tmp['volume'], showlegend=False), row=2, col=1)
# Do not show OHLC's rangeslider plot
fig.update(layout_xaxis_rangeslider_visible=False)
fig.layout.yaxis2.showgrid=False
fig.update_layout(title_text=title,title_x=0.45)
fig.show()
我在 colors 的不同子圖中的 Plotting Volume 上的兩分錢,它只是使 @user6397960 響應更短而無需黑客獲得正確的顏色,只需使用marker_color
。 想一想,是什么讓蠟燭變綠? 收盤價高於開盤價的事實,紅色蠟燭呢? 好吧,收盤價低於開盤價,所以有了這個基礎知識:
import plotly.graph_objects as go
from plotly.subplots import make_subplots
# Create a Figure with 2 subplots, one will contain the candles
# the other will contain the Volume bars
figure = make_subplots(rows=2, cols=1, shared_xaxes=True, row_heights=[0.7, 0.3])
# Plot the candles in the first subplot
figure.add_trace(go.Candlestick(x=df.index, open=df.open, high=df.high, low=df.low, close=df.close, name='price',
increasing_line_color='#26a69a', decreasing_line_color='#ef5350'),
row=1, col=1)
# From our Dataframe take only the rows where the Close > Open
# save it in different Dataframe, these should be green
green_volume_df = df[df['close'] > df['open']]
# Same for Close < Open, these are red candles/bars
red_volume_df = df[df['close'] < df['open']]
# Plot the red bars and green bars in the second subplot
figure.add_trace(go.Bar(x=red_volume_df.index, y=red_volume_df.volume, showlegend=False, marker_color='#ef5350'), row=2,
col=1)
figure.add_trace(go.Bar(x=green_volume_df.index, y=green_volume_df.volume, showlegend=False, marker_color='#26a69a'),
row=2, col=1)
# Hide the Range Slider
figure.update(layout_xaxis_rangeslider_visible=False)
figure.update_layout(title=f'BTC/USDT', yaxis_title=f'Price')
figure.update_yaxes(title_text=f'Volume', row=2, col=1)
figure.update_xaxes(title_text='Date', row=2)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.