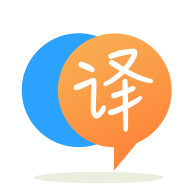
[英]How do i change a button color when it is pressed and to original when other button pressed. Buttons are created using class in python
[英]How do i make the tiles in a grid change color When pressed?
所以我能夠使用 Tkinter 在 python 中創建一個網格,但我不知道如何在按下代碼時使每個單元格改變顏色:
import tkinter as tk
def create_grid(event=None):
w = c.winfo_width() # Get current width of canvas
h = c.winfo_height() # Get current height of canvas
c.delete('grid_line') # Will only remove the grid_line
# Creates all vertical lines at intevals of 100
for i in range(0, w, 100):
c.create_line([(i, 0), (i, h)], tag='grid_line')
# Creates all horizontal lines at intevals of 100
for i in range(0, h, 100):
c.create_line([(0, i), (w, i)], tag='grid_line')
root = tk.Tk()
c = tk.Canvas(root, height=1000, width=1000, bg='white')
c.pack(fill=tk.BOTH, expand=True)
c.bind('<Configure>', create_grid)
root.mainloop()
我不完全知道你所說的“按下時”是什么意思,所以我假設你的意思是“當鼠標按下瓷磚時”。
第一步是以更容易復制的格式放置行:
lineNumber = 10 # <-- CHANGED
def create_grid(event=None):
w = c.winfo_width() # Get current width of canvas
h = c.winfo_height() # Get current height of canvas
c.delete('grid_line') # Will only remove the grid_line
for i in range(0, w, w//lineNumber): # <-- CHANGED
c.create_line([(i, 0), (i, h)], tag='grid_line')
for i in range(0, h, h//lineNumber): # <-- CHANGED
c.create_line([(0, i), (w, i)], tag='grid_line')
下一步是查找鼠標單擊事件並從 x 和 y 計算目標立方體:
def onClick(event):
if event.num == 1: # LEFT CLICK
cLenX = c.winfo_width()//lineNumber
cLenY = c.winfo_height()//lineNumber
x, y = event.x, event.y
cubeX = (x-(x % cLenX)) / cLenX
cubeY = (y-(y % cLenY)) / cLenY
print(cubeX, cubeY)
# canvas.create_polygon(x0, y0, x1, y1,...xn, yn, options)
c.create_polygon(cLenX*cubeX, cLenY*cubeY, cLenX*(cubeX+1), cLenY*cubeY, cLenX*(cubeX+1), cLenY*(cubeY+1), cLenX*cubeX, cLenY*(cubeY+1), fill="red")
root.bind("<Button>", onClick)
將它添加到您的代碼中,您就可以開始了。 如果你懶惰復制粘貼這個:
import tkinter as tk
lineNumber = 10
def create_grid(event=None):
w = c.winfo_width() # Get current width of canvas
h = c.winfo_height() # Get current height of canvas
c.delete('grid_line') # Will only remove the grid_line
# Creates all vertical lines at intevals of 100
for i in range(0, w, int(w/lineNumber)):
c.create_line([(i, 0), (i, h)], tag='grid_line')
# Creates all horizontal lines at intevals of 100
for i in range(0, h, int(h/lineNumber)):
c.create_line([(0, i), (w, i)], tag='grid_line')
root = tk.Tk()
c = tk.Canvas(root, height=500, width=500, bg='white')
c.pack(fill=tk.BOTH, expand=True)
c.bind('<Configure>', create_grid)
def onClick(event):
if event.num == 1: # LEFT CLICK
cLenX = int(c.winfo_width()/lineNumber)
cLenY = int(c.winfo_height()/lineNumber)
x, y = event.x, event.y
cubeX = (x-(x % cLenX)) / cLenX
cubeY = (y-(y % cLenY)) / cLenY
print(cubeX, cubeY)
# canvas.create_polygon(x0, y0, x1, y1,...xn, yn, options)
c.create_polygon(cLenX*cubeX, cLenY*cubeY, cLenX*(cubeX+1), cLenY*cubeY, cLenX*(cubeX+1), cLenY*(cubeY+1), cLenX*cubeX, cLenY*(cubeY+1), fill="red")
root.bind("<Button>", onClick)
root.mainloop()
實際上,該代碼仍然不完美:
我希望你自己弄清楚如何解決這些問題!
在您的代碼中,請在create_grid函數中添加此 for 循環
for i in range (0, w, 100):
for j in range (0, h, 100):
x=c.create_rectangle(i, j,i+100,j+100,fill='white')
c.tag_bind(x, '<ButtonRelease-1>', onObjectClick)
然后在create_grid函數之前添加以下函數
def onObjectClick(event):
x=event.widget.find_closest(event.x, event.y)[0]
c.itemconfig(x,fill="blue")
所以你的完整代碼是
import tkinter as tk
###################Add this function also###################
def onObjectClick(event):
x=event.widget.find_closest(event.x, event.y)[0]
c.itemconfig(x,fill="blue")
############################################################
def create_grid(event=None):
global areas
w = c.winfo_width() # Get current width of canvas
h = c.winfo_height() # Get current height of canvas
c.delete('grid_line') # Will only remove the grid_line
# Creates all vertical lines at intevals of 100
for i in range(0, w, 100):
c.create_line([(i, 0), (i, h)], tag='grid_line')
# Creates all horizontal lines at intevals of 100
for i in range(0, h, 100):
c.create_line([(0, i), (w, i)], tag='grid_line')
#####Add this loop#############################################
for i in range (0, w, 100):
for j in range (0, h, 100):
x=c.create_rectangle(i, j,i+100,j+100,fill='white')
c.tag_bind(x, '<ButtonRelease-1>', onObjectClick)
#####Add this for loop##########################################
root = tk.Tk()
c = tk.Canvas(root, height=1000, width=1000, bg='white')
c.pack(fill=tk.BOTH, expand=True)
c.bind('<Configure>', create_grid)
root.mainloop()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.