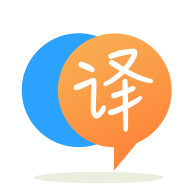
[英]JAVA: How to convert String ArrayList to Integer Arraylist?
[英]How to convert a String ArrayList to an Integer ArrayList?
我正在嘗試將字符串數組列表轉換為整數數組列表,但它使我的應用程序崩潰並給出錯誤為NumberFormatException
,請提供任何幫助。
List<String> sample = new ArrayList<String>(set2);
List<Integer> sample2 = new ArrayList<Integer>(sample.size());
for (String fav : sample) {
sample2.add(Integer.parseInt(fav));
}
每次添加到列表時都嘗試此方法,以確保添加的只是數字字符串(用 '0'-'9' 中的字符表示的字符串。)
public static boolean isNumeric(String strNum) {
if (strNum == null) {
return false;
}
try {
double d = Double.parseDouble(strNum);
} catch (NumberFormatException nfe) {
return false;
}
return true;
}
所以你的代碼看起來像:
List<String> sample = new ArrayList<String>(set2);
List<Integer> sample2 = new ArrayList<Integer>(sample.size());
for (String fav : sample) {
if (isNumeric(fav))
sample2.add(Integer.parseInt(fav));
}
這應該會讓你無一例外地離開。
在這段代碼中,我添加了這段代碼fav.chars().allMatch( Character::isDigit )
來檢查字符串文本的所有字符是否都是非數字,如果為真,則將其添加到sample2
數組中:
List<String> sample = new ArrayList<String>(set2);
List<Integer> sample2 = new ArrayList<Integer>(sample.size());
for (String fav : sample) {
if(fav.chars().allMatch( Character::isDigit )) {
sample2.add(Integer.parseInt(fav));
}
}
您可以過濾掉那些包含非數字字符的字符串以避免此錯誤:
List<String> sample = List.of("123", "235", "abc!", " ");
List<Integer> sample2 = sample.stream()
.filter(str -> str.codePoints()
// all characters are digits
.allMatch(Character::isDigit))
.map(Integer::parseInt)
.collect(Collectors.toList());
System.out.println(sample2); // [123, 235]
根據您的代碼:
第一件事是如何確定樣本數組中的所有字符串都是整數。 如果您確定它們是整數,那么您的代碼就可以了。 因為它是一個應用程序,所以你不需要它崩潰,所以在我看來正確吞下異常是好的(除非你正確記錄發生了什么)。
因此,如果可能,最好的方法是使用List<Integer>
列表(首先限制非整數值)。 但是如果需要字符串,那么您可以檢查它是否可以先被解析,然后將其添加到列表中,即如果只需要避免解析異常。 希望這是有道理的。
方法 1(如果列表初始列表大小無關緊要,則建議使用):
public static void main(String[] args){
List<String> sample=new ArrayList<>(Arrays.asList("1","2","non-integer")); //here the non-integer throws a number format exception but the try catch block catches it and returns 0;
List<Integer> sample2=new ArrayList<>(sample.size());
for (String fav:sample){
if ( isIntegerParseable(fav) ) {
sample2.add(Integer.parseInt(fav));
}
}
// sample2 output is : [1, 2]
}
private static boolean isIntegerParseable(String fav) {
try{
if(fav == null) return false;
Integer.parseInt(fav);
return true;
}catch (NumberFormatException nfe){
//logg this error ( the following value is not a number);
}
return false;
}
方法 2(不推薦):
public static void main(String[] args){
List<String> sample=new ArrayList<>(Arrays.asList("1","2","non-integer")); //here the non-integer throws a number format exception but the try catch block catches it and returns 0;
List<Integer> sample2=new ArrayList<>(sample.size());
for (String fav:sample){
sample2.add(toIntOrDefault(fav));
}
// "If you are returning a null then [1, 2, null] but returning null is even risky as it may result NPE"
// sample2 output is : [1, 2, 0]
}
private static int toIntOrDefault(String value){
try{
return Integer.parseInt(value);
}catch (NumberFormatException nfe){
//logg this error ( the following value is not a number);
// may throw your custom exceptions if any or default value in this case
}
return 0; //you can even return null and change return type to Integer as you are using List<Integer> which is an object type list.
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.