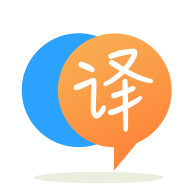
[英]How do I convert to binary in javascript? How do I have a recursive function return data from each call all at once?
[英]I have written a recursive function but it only returns one child, how can I get this to return all children?
我有一組表示 HTML 頁面標記結構的對象。 我編寫了一個遞歸函數,該函數部分工作,但無法弄清楚如何從具有多個子項的對象返回多個子項。
這是對象數組:
const arrayOfObjects = [
{"type": "Root", "id":0, "children" : [
{"type" : "Title", "id": "foo"},
{"type" : "Image", "id": "bar"},
{"type" : "Container", "id": "blo", "children":[
{"type" : "Container", "id": "goo", "children":[
{"type" : "Image", "id": "tar"},
{"type" : "Video", "id": "yar"}
]},
{"type" : "Container", "id": "sha", "children":[
{"type" : "Title", "id": "koo"},
]},
]},
{"type" : "Container", "id": "boo", "children":[
{"type" : "Container", "id": "bos", "children":[
{"type" : "Container", "id": "ooo", "children":[
{"type" : "Container", "id": "loo", "children":[
{"type" : "Title", "id": "bar"},
{"type" : "Image", "id": "rab"}
]}
]}
]}
]}
]}
]
這是我的代碼:
const findChildren = (el) => {
let x = []
if(el.type === "Container"){
x += '[' + el.type + ']'
+ findChildren(el.children[0])
+ '[/' + el.type + ']'
return x
}
else{
return x += '[' + el.type + '/]'
}
}
genStructure = (data) => {
let output = []
data.forEach(function(el) {
output += findChildren(el)
});
return output
}
genStructure(arrayOfObjects[0].children) // here I ignore root as it will always be the same
這是我運行它時得到的。
[Title/][Image/][Container][Container][Image/][/Container][/Container][Container][Container][Container][Container][Title/][/Container][/Container][/Container][/Container]
但這正是我運行它時所需要的。 如您所見,缺少一個帶有標題的容器(容器的sha
id 和標題的koo
),並且缺少標題( rab
id )
[Title/][Image/][Container][Container][Image/][Video/][/Container][Container][Title/][/Container][/Container][Container][Container][Container][Container][Title/][Image/][/Container][/Container][/Container][/Container]
我覺得我可能很接近,但我無法弄清楚我做錯了什么。 我怎樣才能得到缺失的數據?
這是它的 jsfiddle https://jsfiddle.net/gwxhymq4/5/
const findChildren = (el) => {
let x = '';
if(el.type === "Container"){
el.children.forEach(function(child) {
x += findChildren(child)
});
return '[' + el.type + ']' + x + '[/' + el.type + ']';
}
else{
return '[' + el.type + '/]'
}
}
而不是+ findChildren(el.children[0])
,您應該使用.map
來遍歷所有孩子並獲取結果字符串(不僅僅是第一個孩子):
+ el.children.map(findChildren).join('')
或者再次調用genStructure
,它使用相同的邏輯。
此外,將x
初始化為空字符串,或者完全保留它並只return
if
/ else
的表達式會更合適:
const arrayOfObjects = [ {"type": "Root", "id":0, "children" : [ {"type" : "Title", "id": "foo"}, {"type" : "Image", "id": "bar"}, {"type" : "Container", "id": "blo", "children":[ {"type" : "Container", "id": "goo", "children":[ {"type" : "Image", "id": "tar"}, {"type" : "Video", "id": "yar"} ]}, {"type" : "Container", "id": "sha", "children":[ {"type" : "Title", "id": "koo"}, ]}, ]}, {"type" : "Container", "id": "boo", "children":[ {"type" : "Container", "id": "bos", "children":[ {"type" : "Container", "id": "ooo", "children":[ {"type" : "Container", "id": "loo", "children":[ {"type" : "Title", "id": "bar"}, {"type" : "Image", "id": "rab"} ]} ]} ]} ]} ]} ] const findChildren = (el) => { if(el.type === "Container"){ return ( '[Container]' + mapChildren(el) + '[/Container]' ); } else{ return '[' + el.type + '/]' } }; const mapChildren = (element) => { return element.children.map(findChildren).join(''); } console.log(mapChildren(arrayOfObjects[0]));
這是 HTML 代碼。
<div id='meh'>
</div>
這是 JavaScript 代碼。
const arrayOfObjects = [
{"type": "Root", "id":0, "children" : [
{"type" : "Title", "id": "foo"},
{"type" : "Image", "id": "bar"},
{"type" : "Container", "id": "blo", "children":[
{"type" : "Container", "id": "goo", "children":[
{"type" : "Image", "id": "tar"},
{"type" : "Video", "id": "yar"}
]},
{"type" : "Container", "id": "sha", "children":[
{"type" : "Title", "id": "koo"},
]},
]},
{"type" : "Container", "id": "boo", "children":[
{"type" : "Container", "id": "bos", "children":[
{"type" : "Container", "id": "ooo", "children":[
{"type" : "Container", "id": "loo", "children":[
{"type" : "Title", "id": "bar"},
{"type" : "Image", "id": "rab"}
]}
]}
]}
] }
]}
]
const findChildren = (el) => {
let x = []
if(el.type === "Container"){
x += '[' + el.type + ']';
el.children.forEach(e => {
x += findChildren(e)
});
x += '[/' + el.type + ']';
return x
}
else{
return x += '[' + el.type + '/]'
}
}
genStructure = (data) => {
let output = []
data.forEach(function(el) {
output += findChildren(el)
});
return output
}
const el = document.getElementById("meh")
el.innerHTML = genStructure(arrayOfObjects[0].children)
這就是結果。
[Title/][Image/][Container][Container][Image/][Video/][/Container][Container][Title/][/Container][/Container][Container][Container][Container][Container][Title/][Image/][/Container][/Container][/Container][/Container]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.