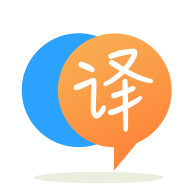
[英]How can add a delay to Progress Dialog - Java - Android - Android Studio
[英]How can I put a delay before code is execute in Android studio Java
我正在創建 Simom Says Game,如何在執行代碼之前延遲。 在圖片 1 中,我需要它等待 1 秒,然后它才會自動按下按鈕。
這是完整的代碼:
public class GameActivity extends AppCompatActivity implements View.OnClickListener {
Random rand = new Random();
Handler handler = new Handler(Looper.getMainLooper());
Button btnGreen, btnRed, btnYellow, btnBlue;
TextView tvScore;
MediaPlayer mpSound1, mpSound2, mpSound3, mpSound4;
Animation animation;
boolean gameOver = false;
boolean buttonClicked = false;
String[] fourColors = {"Green", "Red", "Blue", "Yellow"};
ArrayList<String> allColors = new ArrayList<>();
int count = 0;
int scoreToWin = 8;
int i = 0;
public void ClickGreen(){
btnGreen.startAnimation(animation);
mpSound1.start();
}
public void ClickRed(){
btnRed.startAnimation(animation);
mpSound2.start();
}
public void ClickBlue(){
btnBlue.startAnimation(animation);
mpSound3.start();
}
public void ClickYellow(){
btnYellow.startAnimation(animation);
mpSound4.start();
}
public void game(){
count = 0;
i = 0;
int random;
random = rand.nextInt(4);
allColors.add(fourColors[random]);
handler.postDelayed(new Runnable() {
@Override
public void run() {
for(i = 0; i < allColors.size(); i++) {
switch (allColors.get(i)) {
case "Green":
ClickGreen();
Toast.makeText(GameActivity.this, "Green", Toast.LENGTH_SHORT).show();
break;
case "Red":
ClickRed();
Toast.makeText(GameActivity.this, "Red", Toast.LENGTH_SHORT).show();
break;
case "Blue":
ClickBlue();
Toast.makeText(GameActivity.this, "Blue", Toast.LENGTH_SHORT).show();
break;
case "Yellow":
ClickYellow();
Toast.makeText(GameActivity.this, "Yellow", Toast.LENGTH_SHORT).show();
break;
}
}
}
}, 1000);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game);
animation = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.push_down);
tvScore = findViewById(R.id.tvScore);
btnGreen = findViewById(R.id.btnGreen);//0
btnRed = findViewById(R.id.btnRed);//1
btnBlue = findViewById(R.id.btnBlue);//2
btnYellow = findViewById(R.id.btnYellow);//3
btnGreen.setOnClickListener(this);
btnRed.setOnClickListener(this);
btnBlue.setOnClickListener(this);
btnYellow.setOnClickListener(this);
mpSound1 = MediaPlayer.create(this, R.raw.sound1);
mpSound2 = MediaPlayer.create(this, R.raw.sound2);
mpSound3 = MediaPlayer.create(this, R.raw.sound3);
mpSound4 = MediaPlayer.create(this, R.raw.sound4);
game();
}
@Override
public void onClick(View v) {
if(v.equals(btnGreen)) {
btnGreen.startAnimation(animation);
mpSound1.start();
gameOver = !allColors.get(count).equals("Green");
count++;
buttonClicked = true;
}
else if(v.equals(btnRed)) {
btnRed.startAnimation(animation);
mpSound2.start();
gameOver = !allColors.get(count).equals("Red");
count++;
buttonClicked = true;
}
else if(v.equals(btnBlue)) {
btnBlue.startAnimation(animation);
mpSound3.start();
gameOver = !allColors.get(count).equals("Blue");
count++;
buttonClicked = true;
}
else if(v.equals(btnYellow)) {
btnYellow.startAnimation(animation);
mpSound4.start();
gameOver = !allColors.get(count).equals("Yellow");
count++;
buttonClicked = true;
}
if(buttonClicked){
if (gameOver) {
Intent intent = new Intent(GameActivity.this, GameOverActivity.class);
startActivity(intent);
} else {
if (count >= allColors.size()) {
tvScore.setText(String.valueOf(allColors.size()));
game();
buttonClicked = false;
if(allColors.size() == scoreToWin){
Toast.makeText(GameActivity.this, "WON!", Toast.LENGTH_SHORT).show();
}
}
}
}
}
}
您可以像這樣添加延遲,等待 1 秒: delay(1000)
使用以下代碼在 android 應用程序中添加延遲。
import android.os.Handler;
final Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
// Do something after 5s = 5000ms
// Add your function here which will be executed after 1 second
}
}, 1000);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.