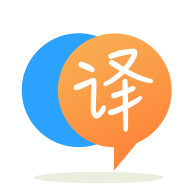
[英]Jest Unit Testing function that calls a second one that returns a promise
[英]Jest unit testing a function that calls a function returning a Promise
我有一個 function,它調用一個 function,它返回一個 Promise。 這是它的代碼:
export const func1 = ({
contentRef,
onShareFile,
t,
trackOnShareFile,
}) => e => {
trackOnShareFile()
try {
func2(contentRef).then(url => {
onShareFile({
title: t('shareFileTitle'),
type: 'application/pdf',
url,
})
}).catch(e => {
if (process.env.NODE_ENV === 'development') {
console.error(e)
}
})
e.preventDefault()
} catch (e) {
if (process.env.NODE_ENV === 'development') {
console.error(e)
}
}
}
在func2
中調用的func1
是這樣的:
const func2 = element => {
return import('html2pdf.js').then(html2pdf => {
return html2pdf.default().set({ margin: 12 }).from(element).toPdf().output('datauristring').then(pdfAsString => {
return pdfAsString.split(',')[1]
}).then(base64String => {
return `data:application/pdf;base64,${base64String}`
})
})
}
現在我正在嘗試為func1
編寫一些單元測試,但遇到了一些問題。 到目前為止我所做的是:
describe('#func1', () => {
it('calls `trackOnShareFile`', () => {
// given
const props = {
trackOnShareFile: jest.fn(),
onShareFile: jest.fn(),
shareFileTitle: 'foo',
contentRef: { innerHTML: '<div>hello world</div>' },
}
const eventMock = {
preventDefault: () => {},
}
// when
func1(props)(eventMock)
// then
expect(props.trackOnShareFile).toBeCalledTimes(1)
})
it('calls `onShareFile` prop', () => {
// given
const props = {
trackOnShareFile: jest.fn(),
onShareFile: jest.fn(),
shareFileTitle: 'foo',
contentRef: { innerHTML: '<div>hello world</div>' },
}
const eventMock = {
preventDefault: () => {},
}
// when
func1(props)(eventMock)
// then
expect(props.onShareFile).toBeCalledTimes(1)
})
})
現在第一次測試通過,但第二次測試我得到Expected mock function to have been called one time, but it was called zero times.
. 我不確定如何正確測試它。 任何形式的幫助都是可觀的。
好的,我已經開始工作了。
首先,我們需要模擬出data-url-generator
(這是我們導入func2
的地方)。 html2pdf
庫在測試環境中不起作用,因為它使用的假 DOM 沒有完全實現 canvas 圖形。
jest.mock('./data-url-generator', () => jest.fn())
那么測試本身可以這樣寫:
it('invokes the `onShareFile` prop', done => {
// given
const t = key => `[${key}]`
const urlMock = 'data:application/pdf;base64,PGh0bWw+PGJvZHk+PGRpdj5oZWxsbyB3b3JsZDwvZGl2PjwvYm9keT48L2h0bWw+'
const shareFileTitle = 'bar'
const contentRef = document.createElement('div')
contentRef.textContent = 'hello world'
const trackOnShareFile = () => { }
const eventMock = {
preventDefault: () => { },
}
func2.mockResolvedValue(urlMock)
const onShareFileMock = ({ title, type, url }) => {
// then
expect(func2).toHaveBeenCalledTimes(1)
expect(func2).toHaveBeenCalledWith(contentRef)
expect(title).toBe('[shareFileTitle]')
expect(type).toBe('application/pdf')
expect(url).toBe(urlMock)
done()
}
// when
func1({
contentRef,
onShareFile: onShareFileMock,
shareFileTitle,
t,
trackOnShareFile,
})(eventMock)
})
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.