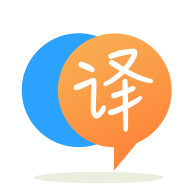
[英]TypeError: super(type, obj): obj must be an instance or subtype of type. Error when calling super after metaclass creation
[英]TypeError: super(type, obj): obj must be an instance or subtype of type ONLY when my metaclass is imported
我創建了一個元類,通過父 class 管理__init__
function 參數的 inheritance 參數。 讓我告訴你我的意思:
class A(metaclass= MagicMeta):
def __init__(self, a, taunt = None):
print(locals())
self.a = a
self.taunt = taunt
class B(A, metaclass=MagicMeta):
def __init__(self, b):
self.b = b
class Foo(B,metaclass=MagicMeta):
def __init__(self,yolo, name ='empty', surname = None):
print(yolo,a,b)
self.name = name
self.surname= surname
o =Foo(1,2,3,taunt='taunted')
o.taunt
>>> 'taunted'
o.b
>>> 2
我的元類在寫入與我的 class 相同的文件時運行良好但是當我導入它時,我收到此錯誤: TypeError: super(type, obj): obj must be an instance or subtype of type when my metaclass is imported
import
我的元類:
import re
from inspect import Parameter
def get_args(f):
args = list()
kwargs = dict()
for param in inspect.signature(f).parameters.values():
if (param.kind == param.POSITIONAL_OR_KEYWORD):
if param.default ==Parameter.empty:
args.append(param.name)
else:
kwargs[param.name]= param.default
return args, kwargs
def compileKwargs(dct):
string =""
poke = False
for k, o in dct.items():
if type(o) == str:
string+= k+"='"+o+"', "
else:
string+= k+"="+str(o)+", "
return string
def compileKwargs2(dct):
string =""
poke = False
for k, o in dct.items():
if type(o) == str:
string+= k+"='"+k+"', "
else:
string+= k+"="+k+", "
return string
def stringArgs(liste):
return " ".join([e+"," for e in liste])
def compileArgs(liste1,liste2):
liste1.extend([e for e in liste2 if e not in liste1])
return liste1
def editFuncName(actual: str, replace:str):
#print('EDITFUNCNAME')
#print(actual)
string = re.sub('(?<=def ).*?(?=\()',replace, actual)
#print('string', string)
return string
import inspect
from textwrap import dedent, indent
def processCode(code : list):
string=""
#print('processcode')
for i,e in enumerate(code):
#print('row', e)
#print('dedent', e)
if i != 0:
string+=indent(dedent(e),'\t')
else :
string+=dedent(e)
return string
import types
class MagicMeta(type):
def __init__(cls, name, bases, dct):
#print(bases,dct)
setattr(cls,'_CODE_', dict())
#GET THE __init__ code function and its arg and kwargs
# for the class and the inherited class
func = cls.__init__
cls._CODE_[func.__name__]= inspect.getsourcelines(func)
args2 =get_args(cls.__bases__[0].__init__)
setattr(cls,'_ARGS_', dict())
cls._ARGS_[func.__name__]=[get_args(func), args2]
lines = cls._CODE_['__init__']
string= lines[0][0]
arg, kwarg = cls._ARGS_['__init__'][0]
arg2, kwarg2 = cls._ARGS_['__init__'][1]
comparg = stringArgs(compileArgs(arg, arg2))
#------------------------------------------------------
#PROCESS arg and kwargs to manage it as string
dct = {**kwarg ,**kwarg2}
#print(dct)
newargs = comparg + compileKwargs(dct)
string = re.sub('(?<=\().*?(?=\))',newargs, string)
print(type(arg2))
print(arg2)
superarg =stringArgs([a for a in arg2 if a != 'self']) + compileKwargs2(kwarg2)
arg =stringArgs([a for a in arg2 if a != 'self'])
printt = "print({})\n".format(arg)
printtt = "print(locals())\n"
print(superarg)
#--------------------------------------------------------
#ADD the super().__init__ in the __init__ function
superx = "super({},self).{}({})\n".format(cls.__name__, func.__name__, superarg)
#superx = "super().{}({})\n".format( func.__name__, superarg)
print(superx)
code = lines[0]
#print('LINE DEF', code[0])
#--------------------------------------------------------
#BUILD the code of the new __init__ function
code[0]= editFuncName(string, 'tempo')
code.insert(1, printt)
code.insert(2, "print(self, type(self))\n")
if len(bases)>0:
code.insert(3, superx)
print('code:',code)
codestr = processCode(code)
#print('précompile', codestr)
#--------------------------------------------------------
#REPLACE the __init__ function code
comp = compile(codestr, '<string>','exec')
#print(comp)
exec(comp)
cls.__init__ = types.MethodType(eval('tempo'), cls)
#print(eval('tempo.__code__'))
#--------------------------------------------------------
我會避免在每次需要時設置元類的代碼
此外,我認為,為了深入了解 python,這是一個很好的機會來了解為什么 import 會改變 class 錯誤行為,當它的內部代碼被動態修改時
所以我認為通過一些調整,你可以在不使用元類的情況下解決這個問題。 看起來您想使用 inheritance 讓您的 Foo 實例包含 b、a 和嘲諷。 但是您正在使用有問題的位置參數傳遞它們。 一種解決方案是攝取 *args 和 **kwargs,並在超級調用中將它們傳遞給祖先類。 然后我們可以訪問並刪除 args[0] 來設置 b 和 a。 這是令人擔憂的,因為如果 inheritance 訂單發生變化,那么 b 和 a 是什么變化。 例如:
class A:
def __init__(self, *args):
args = list(args)
a = args.pop(0)
self.a = a
try:
super().__init__(*args)
except TypeError:
pass
class B:
def __init__(self, *args):
args = list(args)
b = args.pop(0)
self.b = b
try:
super().__init__(*args)
except TypeError:
pass
class C(B,A):
pass
class D(A, B):
pass
c = C('a', 'b')
d = D('a', 'b')
>>> c.__dict__
{'b': 'a', 'a': 'b'}
>>> d.__dict__
{'a': 'a', 'b': 'b'}
我們不能依靠這種方法來可靠地設置 a 和 b 是什么。 因此,我們應該使用關鍵字 args。 此代碼使用關鍵字 arguments 來設置這些祖先參數:
class NewA:
def __init__(self, *, a, taunt = None):
self.a = a
self.taunt = taunt
class NewB(NewA):
def __init__(self, *, b, **kwargs):
super().__init__(**kwargs)
self.b = b
class NewFoo(NewB):
def __init__(self, yolo, name ='empty', surname = None, **kwargs):
super().__init__(**kwargs)
self.name = name
self.surname= surname
f = NewFoo(1, b=2, a=3,taunt='taunted')
>>> print(f.__dict__)
{'a': 3, 'taunt': 'taunted', 'b': 2, 'name': 'empty', 'surname': None}
在其中,我們使用 python3 中的一項新功能來要求命名為 arguments。 當我們嘗試省略 a 時,我們得到:
>>> NewFoo(1, b=2, taunt='taunted')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 3, in __init__
File "<stdin>", line 3, in __init__
TypeError: __init__() missing 1 required keyword-only argument: 'a'
我們可以通過以下方式要求這些命名為 arguments:
Parameters after “*” or “*identifier” are keyword-only parameters and may only be passed used keyword arguments.
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.