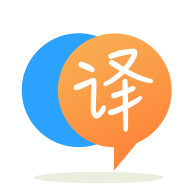
[英]How can I convert hamming number code in a while or for loop python
[英]How can I transform my for loop code into the while loop in Python?
我正在嘗試編寫一個名為 has_no_e 的 function,它將字符串作為參數,如果字符串包含字母 e,則返回 False,如果字符串不包含字母 e,則返回 True。
我已經使用 for 循環編寫了 function,但我沒有編寫類似的 function 使用 while 循環執行相同的操作。
這是我的 function 與 for 循環:
def has_no_e(word):
for letters in word:
if letters == "e":
return False
return True
而我寫的function是:
def has_no_e2(word):
index = 0
while index < len(word):
if word[index] != "e":
index += 1
return False
return True
你能告訴我我的 function 和 while 循環有什么問題嗎? 它每次都返回 False,而不取決於是否有“e”。
有兩種方法可以為此實現相同的修復。 與您現有代碼更接近的一個是
def has_no_e2(word):
index = 0
while index < len(word):
if word[index] != "e":
index += 1
continue # skip the rest of the loop and go back to the top
return False
return True
更好的是
def has_no_e2(word):
index = 0
while index < len(word):
if word[index] == "e":
return False
index += 1
return True
請注意,以下兩段代碼大致等價:
for elem in iterable:
# code
_index = 0
while _index < len(iterable):
elem = iterable[_index]
# code
_index += 1
它比這更復雜,因為for
循環經常使用迭代器而不是索引,但你明白了。
嘗試:
index = 0
while index < len(word):
if word[index] != "e":
index += 1
else:
return False
return True
目前,您的代碼在第一個循環結束時返回 false
您可以使用in運算符:
def has_no_e(word):
return 'e' not in word
如果你真的需要一個while循環:
def while_has_no_e(word):
characters = list(word)
while characters:
if characters.pop() == 'e':
return False
return True
print(has_no_e('foo'))
print(has_no_e('element'))
print(while_has_no_e('foo'))
print(while_has_no_e('element'))
出去:
True
False
True
False
def has_no_e1(_string):
return 'e' not in _string
def has_no_e2(_string):
return _string.find('e') == -1
def has_no_e3(_string):
while _string:
if _string[0] == 'e':
return False
_string = _string[1:]
return True
if __name__ == "__main__":
word = 'With the letter `e`'
print(
has_no_e1(word),
has_no_e2(word),
has_no_e3(word),
)
word = 'Without it'
print(
has_no_e1(word),
has_no_e2(word),
has_no_e3(word),
)
一些變化,你對 go 很好,你只需要將你在 for 循環中寫的任何條件寫到 while 中,就是這樣:
def has_no_e2(word):
index = 0
while index < len(word):
if word[index] == "e":
return False
index+=1
return True
現在讓我給你一個襯里:
>>> string = 'hello'
>>> False if string.count('e') > 0 else True
False
#changing the contents
>>> string = 'hlo'
>>> False if string.count('e') > 0 else True
True
您不需要創建 function,它已經存在並且它被稱為 find()
has_e = word.find("e")
function 要么返回字母的確切 position,要么返回 -1(如果不存在)。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.