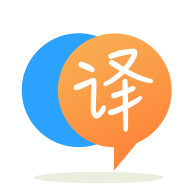
[英]How can I write tests that have setup and teardown operations that are asynchronous?
[英]Trying to learn how to write tests, how do I write when I have a promise?
I created a function that connects to an API and returns a promise.. I wanted to test that function so created a mock function in Jest to handle it, however I am not really sure I am doing this right and I am finding it very hard找到有關如何編寫良好單元測試的 go 的良好資源..
export const sbConnect = (userId) => {
return new Promise((resolve, reject) => {
const sb = new SendBird({appId: APP_ID});
sb.connect(userId, API_Token, (user, error) => {
if (error) {
reject(error);
} else {
resolve(user);
}
});
});
};
這是我正在嘗試測試的 function。 到目前為止,我已經創建了一個測試並嘗試了這個..
import {sbConnect} from '../helpers/sendBirdSetupActions';
const SendBird = jest.fn();
describe('Connects to SendBird API', () => {
it('Creates a new SB instance', () => {
let userId = 'testUser';
let APP_ID = 'Testing_App_ID_001';
sbConnect(userId);
expect(SendBird).toBeCalled();
});
});
您可以使用jest.mock(moduleName, factory, options)手動模擬sendbird
模塊。
模擬SendBird
構造函數、實例及其方法。
例如
index.ts
:
import SendBird from 'sendbird';
const API_Token = 'test api token';
const APP_ID = 'test api id';
export const sbConnect = (userId) => {
return new Promise((resolve, reject) => {
const sb = new SendBird({ appId: APP_ID });
sb.connect(userId, API_Token, (user, error) => {
if (error) {
reject(error);
} else {
resolve(user);
}
});
});
};
index.test.ts
:
import { sbConnect } from './';
import SendBird from 'sendbird';
const mSendBirdInstance = {
connect: jest.fn(),
};
jest.mock('sendbird', () => {
return jest.fn(() => mSendBirdInstance);
});
describe('65220363', () => {
afterAll(() => {
jest.resetAllMocks();
});
it('should get user', async () => {
const mUser = { name: 'teresa teng' };
mSendBirdInstance.connect.mockImplementationOnce((userId, API_Token, callback) => {
callback(mUser, null);
});
const actual = await sbConnect('1');
expect(actual).toEqual(mUser);
expect(SendBird).toBeCalledWith({ appId: 'test api id' });
expect(mSendBirdInstance.connect).toBeCalledWith('1', 'test api token', expect.any(Function));
});
it('should handle error', async () => {
const mError = new Error('network');
mSendBirdInstance.connect.mockImplementationOnce((userId, API_Token, callback) => {
callback(null, mError);
});
await expect(sbConnect('1')).rejects.toThrow(mError);
expect(SendBird).toBeCalledWith({ appId: 'test api id' });
expect(mSendBirdInstance.connect).toBeCalledWith('1', 'test api token', expect.any(Function));
});
});
單元測試結果:
PASS src/stackoverflow/65220363/index.test.ts (11.115s)
65220363
✓ should get user (6ms)
✓ should get user (3ms)
----------|----------|----------|----------|----------|-------------------|
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s |
----------|----------|----------|----------|----------|-------------------|
All files | 100 | 100 | 100 | 100 | |
index.ts | 100 | 100 | 100 | 100 | |
----------|----------|----------|----------|----------|-------------------|
Test Suites: 1 passed, 1 total
Tests: 2 passed, 2 total
Snapshots: 0 total
Time: 12.736s
源代碼: https://github.com/mrdulin/jest-codelab/tree/master/src/stackoverflow/65220363
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.