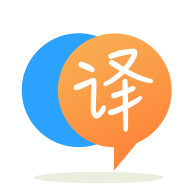
[英]I have a problem with the implementing a try and catch block on Java, could I have some advice on how to go about it?
[英]I have a problem with splitting up my Java program into separate methods within the same class, could I have some advice on how to go about it?
我的任務是拆分我的程序,該程序允許用戶輸入一個數字數組,然后在 1 到 10 之間的奇數之后檢查奇數是否是數組中 5 個數字中的每一個的因子。 我不斷嘗試不同的方法,但似乎都沒有奏效。 有人可以幫助我或發送我應該如何排序的樣本嗎? 這是程序:
import java.util.Scanner;
public class CheckboxExample{
public static void main(String args[]) {
CheckBox c = new CheckBox();
new CheckboxExample(); // links to checkbox class
Scanner s = new Scanner(System.in);
int array[] = new int[10];
System.out.println ("Please enter 10 random numbers"); // prompts the user to enter 10 numbers
int num; // declares variable num
try{
for (int i = 0; i < 10; i++) {
array[i] = s.nextInt(); // array declaration
}
}catch (Exception e){
System.out.println ("You have an error");
}
System.out.println ("Please enter an odd number between 1 and 10");
try{
num = s.nextInt ();
if (num % 2 == 0){
do{
System.out.println ("\nYour number is even, enter an odd one");
num = s.nextInt ();
}while (num % 2 == 0);
}
if (num < 0 | num > 10){
do{
System.out.println ("Your number is outside of the range, try again");
num = s.nextInt ();
}while (num < 0 | num > 10);
}
for (int i = 0; i < 5 ; i++){
if (array[i] % num == 0) {
System.out.println("Your number is a factor of " + array[i] );
}
}
}catch (Exception e){
System.out.println ("error");
}
}
}
理想情況下,一種方法應該負責一項任務。 在您的情況下,您應該考慮您的代碼嘗試做的不同事情並在某種意義上組織它們,即您調用的每個方法都在您嘗試做的事情列表中做一件事情。 例如:據我了解,您的代碼執行以下操作:
現在一種可能的方法是將你的代碼分成 5 種方法來完成這些事情。
首先,您調用讀取 10 個數字的方法。 然后調用該方法讀取奇數。 3. 和 4. 實際上是讀取數字的一部分,因為您需要重試無效輸入,因此您可以編寫輸入奇數的方法,使其使用驗證輸入的方法。 最后,當您擁有所有有效輸入時,您調用產生結果的方法(即,如果數字是列表中數字的一個因素)。
您的代碼的一般異常值可能如下所示:
public class CheckboxExample {
public static void main(String args[]) {
CheckBox c = new CheckBox();
new CheckboxExample(); // links to checkbox class
Scanner s = new Scanner(System.in);
int array[] = readInputArray();
int number = readOddValue();
calculateFactors(array, number);
}
private int[] readInputArray() {...}
private int readOddValue() {...}
private void calculateFactors(int[] array, int number) {...}
//additional methods used by readOddValue which verify if the value is actually odd
}
請注意,這只是將代碼拆分為方法的一種方法,並且有多種方法可以設計和實現這些方法。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.