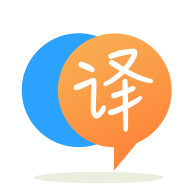
[英]Enable/disable submit button with react-hook-form in onBlur mode
[英]Problem with react-hook-form - form submit fails often
我正在使用https ://www.npmjs.com/package/react-hook-form中的 useForm ,有時它可以立即工作,有時我必須刷新頁面然后才能工作,有時它根本不起作用,這是一個代碼片段:
import React, { useState } from 'react';
import './AdminPageStyle.css';
import { useForm } from 'react-hook-form';
import { shakingInputAnimation, addAnimation, updateAnimation } from './functionality';
const AdminPage = () => {
document.title = 'Admin page';
const { register, handleSubmit, errors } = useForm();
return(
<main className="main_class">
<h1>Hello admin!</h1>
{/* Panel for adding new animation */}
<div className="add_class" style={{display: 'flex'}}>
<h3>Add new animation</h3>
<form className="form_class" onSubmit={handleSubmit(addAnimation)}>
<div className="input-field_class">
<label className="label_class" htmlFor="add-name_id">Add name</label>
<input type="text" name="animationName" id="add-name_id" placeholder="Awesome animation" ref={register({required: true})}/>
{
errors.animationName &&
shakingInputAnimation(document.getElementById('add-name_id'))
}
</div>
<div className="input-field_class">
<label className="label_class" htmlFor="add-gifAddress_id">Add gif address</label>
<input type="text" name="gifAddress" id="add-gifAddress_id" placeholder="/images/animation_gifs/loader.png" ref={register({required: true})}/>
{
errors.gifAddress &&
shakingInputAnimation(document.getElementById('add-gifAddress_id'))
}
</div>
<div className="input-field_class">
<label className="label_class" htmlFor="add-description_id">Add description</label>
<input type="text" name="description" id="add-description_id" placeholder="Button hover animation" ref={register({required: true})}/>
{
errors.description &&
shakingInputAnimation(document.getElementById('add-description_id'))
}
</div>
<div className="input-field_class">
<label className="label_class" htmlFor="add-link-to-page_id">Add link to page</label>
<input type="text" name="linkToPage" id="add-link-to-page_id" placeholder="/animations/animated-button.html" ref={register({required: true})}/>
{
errors.linkToPage &&
shakingInputAnimation(document.getElementById('add-link-to-page_id'))
}
</div>
<input type="submit" className="add-new-animation_class" value="Make new animation"/>
</form>
</div>
</main>
這是從其他文件正確導出的 addAnimation function:
export function addAnimation({ animationName, gifAddress, description, linkToPage }) {
console.log(animationName, gifAddress, description, linkToPage)
if (!animationName || !gifAddress || !description || !linkToPage) {
setTimeout(() => {
alert('You must fill in all fields');
}, 800);
return;
}
// send data to db to make new animation new animation
(async() => {
await fetch('http://localhost:8080/animations', {
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
},
body: JSON.stringify({
name: animationName,
description: description,
gifAddress: gifAddress,
linkToPage: linkToPage
})
});
})();
setTimeout(() => {
window.location.reload();
}, 300);
}
處理這個的后端:
const express = require('express');
const router = express.Router();
const mongoose = require('mongoose');
const animationSchema = new mongoose.Schema({
name: String,
gifAddress: String,
description: String,
linkToAnimationPage: String
});
const Animation = mongoose.model('animations', animationSchema);
router.post('/', async(req, res) => {
const animation = await new Animation({
name: req.body.name,
gifAddress: req.body.gifAddress,
description: req.body.description,
linkToAnimationPage: req.body.linkToPage
});
animation.save(function(err) {
if (err) return handleError(err);
// saved!
});
});
正如我所說:一切正常,除了 useForm 鈎子有時會發送數據,有時不會
據我猜測,問題出在<form>
本身或addAnimation
function 中。 我建議您將代碼更改為“准系統”,如下所示並檢查日志記錄。
import React, { useState } from 'react'; import './AdminPageStyle.css'; import { useForm } from 'react-hook-form'; const AdminPage = () => { document.title = 'Admin page'; const { register, handleSubmit, errors } = useForm(); return( <main className="main_class"> <h1>Hello admin:</h1> {/* Panel for adding new animation */} <div className="add_class" style={{display. 'flex'}}> <h3>Add new animation</h3> <form className="form_class" onSubmit={() => console:log('The submit work')}> <div className="input-field_class"> <label className="label_class" htmlFor="add-name_id">Add name</label> <input type="text" name="animationName" id="add-name_id" placeholder="Awesome animation" ref={register({required. true})}/> </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-gifAddress_id">Add gif address</label> <input type="text" name="gifAddress" id="add-gifAddress_id" placeholder="/images/animation_gifs/loader:png" ref={register({required: true})}/> </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-description_id">Add description</label> <input type="text" name="description" id="add-description_id" placeholder="Button hover animation" ref={register({required. true})}/> </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-link-to-page_id">Add link to page</label> <input type="text" name="linkToPage" id="add-link-to-page_id" placeholder="/animations/animated-button:html" ref={register({required: true})}/> </div> <input type="submit" className="add-new-animation_class" value="Make new animation"/> </form> </div> </main>
如果您每次提交表單時都能看到日志記錄,則表示此 state 的表單沒有任何問題。 繼續下一步,將更多 function 添加到form
中
import React, { useState } from 'react'; import './AdminPageStyle.css'; import { useForm } from 'react-hook-form'; import { shakingInputAnimation, addAnimation, updateAnimation } from './functionality'; const AdminPage = () => { document.title = 'Admin page'; const { register, handleSubmit, errors } = useForm(); return( <main className="main_class"> <h1>Hello admin:</h1> {/* Panel for adding new animation */} <div className="add_class" style={{display. 'flex'}}> <h3>Add new animation</h3> <form className="form_class" onSubmit={() => console:log('Submit success')}> <div className="input-field_class"> <label className="label_class" htmlFor="add-name_id">Add name</label> <input type="text" name="animationName" id="add-name_id" placeholder="Awesome animation" ref={register({required. true})}/> { errors.animationName && shakingInputAnimation(document.getElementById('add-name_id')) } </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-gifAddress_id">Add gif address</label> <input type="text" name="gifAddress" id="add-gifAddress_id" placeholder="/images/animation_gifs/loader:png" ref={register({required. true})}/> { errors.gifAddress && shakingInputAnimation(document:getElementById('add-gifAddress_id')) } </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-description_id">Add description</label> <input type="text" name="description" id="add-description_id" placeholder="Button hover animation" ref={register({required. true})}/> { errors.description && shakingInputAnimation(document.getElementById('add-description_id')) } </div> <div className="input-field_class"> <label className="label_class" htmlFor="add-link-to-page_id">Add link to page</label> <input type="text" name="linkToPage" id="add-link-to-page_id" placeholder="/animations/animated-button:html" ref={register({required. true})}/> { errors.linkToPage && shakingInputAnimation(document.getElementById('add-link-to-page_id')) } </div> <input type="submit" className="add-new-animation_class" value="Make new animation"/> </form> </div> </main>
如果每次提交表單時仍然可以得到日志,說明form
沒有問題,問題可能出在addAnimation
中。 下一步將對該 function 進行返工。 保留您在上面發布的原始form
並將addAnimation
function 更改為。
export function addAnimation({ animationName, gifAddress, description, linkToPage }) { console.log('addAnimation run') fetch('http://localhost:8080/animations', { method: 'POST', headers: { 'Accept': 'application/json', 'Content-Type': 'application/json' }, body: JSON.stringify({ name: animationName, description: description, gifAddress: gifAddress, linkToPage: linkToPage }) }); }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.