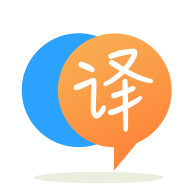
[英]Javascript and React setting state of arrays within arrays and using spread operator
[英]Working with Arrays within Arrays in React Native JavaScript
我從 API (JSON) 接收這種類型的數組:
{
"api": {
"results": 13,
"players": [
{
"player_id": 79308,
"player_name": "Felipe Ponce Ramírez",
"firstname": "Felipe",
"lastname": "Ponce Ramírez",
"number": null,
"position": "Midfielder",
"age": 32,
"birth_date": "29/03/1988",
"birth_place": "Ciudad Lerdo",
"birth_country": "Mexico",
"nationality": "Mexico",
"height": "177 cm",
"weight": "67 kg",
"injured": null,
"rating": null,
"team_id": 4299,
"team_name": "Alianza",
"league_id": 2979,
"league": "Primera Division",
"season": "2020-2021",
"captain": 0,
"shots": {
"total": 0,
"on": 0
},
"goals": {
"total": 5,
"conceded": 0,
"assists": 0,
"saves": 0
},
"passes": {
"total": 0,
"key": 0,
"accuracy": 0
},
"tackles": {
"total": 0,
"blocks": 0,
"interceptions": 0
},
"duels": {
"total": 0,
"won": 0
},
"dribbles": {
"attempts": 0,
"success": 0
},
"fouls": {
"drawn": 0,
"committed": 0
},
"cards": {
"yellow": 2,
"yellowred": 0,
"red": 0
},
"penalty": {
"won": 0,
"commited": 0,
"success": 0,
"missed": 0,
"saved": 0
},
"games": {
"appearences": 7,
"minutes_played": 260,
"lineups": 3
},
"substitutes": {
"in": 4,
"out": 3,
"bench": 0
}
}
// From here on there are 4 objects like this (there are 1 object for the last 5 seasons
}
]
}
}
所以我想將對象提取到 3 Arrays 中:
"goals": {
"total": 5,
"conceded": 0,
"assists": 0,
"saves": 0
},
"cards": {
"yellow": 2,
"yellowred": 0,
"red": 0
},
"games": {
"appearences": 7,
"minutes_played": 260,
"lineups": 3
},
我有這個代碼來接收我的動作文件中的數據(它提供給我的減速器):
import Jugador from '../../models/jugador';
import ResultadoEstadistica from '../../models/estadistica/resultadoEstadistica';
import PlayerEstadistica from '../../models/estadistica/playerEstatisticData';
import Cards from '../../models/estadistica/cards';
import Games from '../../models/estadistica/games';
import Goals from '../../models/estadistica/goals';
export const SET_JUGADORES = 'SET_JUGADORES';
export const SET_ESTADISTICA = 'SET_ESTADISTICA';
export const fetchJugadores = () => {
return async (dispatch) => {
//any async code here!!!
try {
const response = await fetch(
'https://alianzafc2021-default-rtdb.firebaseio.com/jugadores.json'
);
if (!response.ok) {
throw new Error('Algo salio Mal!');
}
const resData = await response.json();
const loadedJugadores = [];
for (const key in resData) {
loadedJugadores.push(
new Jugador(
key,
resData[key].altura,
resData[key].apellido,
resData[key].edad,
resData[key].fecha_nacimiento,
resData[key].iso_code,
resData[key].imagen,
resData[key].lugar_nacimiento,
resData[key].nacionalidad,
resData[key].nombre_completo,
resData[key].nombre_corto,
resData[key].nombres,
resData[key].numero,
resData[key].pais,
resData[key].peso,
resData[key].player_id,
resData[key].posicion
)
);
}
dispatch({ type: SET_JUGADORES, players: loadedJugadores });
} catch (err) {
throw err;
}
};
}
export const fetchEstadistica = player_id => {
return async (dispatch) => {
//any async code here!!!
try {
const response = await fetch(
`https://api-football-v1.p.rapidapi.com/v2/players/player/${player_id}`,
{
method: 'GET',
headers: {
'x-rapidapi-key': 'My API KEY',
'x-rapidapi-host': 'api-football-v1.p.rapidapi.com',
'useQueryString': 'true'
}
}
);
if (!response.ok) {
throw new Error('Algo salio Mal!');
}
const resData = await response.json();
const loadesApiResult = [];
console.log('***Impresion desde la accion***');
console.log(resData);
console.log('***Fin de Impresion***');
//Arrays de la Estadistica del Jugador
const loadedEstadistica = [];
const loadedCards = [];
const loadedGoals = [];
const loadedGames = [];
for (const key in resData) {
loadesApiResult.push(
new ResultadoEstadistica(
resData[key].results,
resData[key].players
)
);
}
const apiData = loadesApiResult.players;
for (const key in apiData) {
loadedEstadistica.push(
new PlayerEstadistica(
apiData[key].player_id,
apiData[key].player_name,
apiData[key].firstname,
apiData[key].lastname,
apiData[key].number,
apiData[key].position,
apiData[key].age,
apiData[key].birth_date,
apiData[key].birth_place,
apiData[key].birth_country,
apiData[key].nationality,
apiData[key].height,
apiData[key].weight,
apiData[key].injured,
apiData[key].rating,
apiData[key].team_id,
apiData[key].team_name,
apiData[key].league_id,
apiData[key].league,
apiData[key].season,
apiData[key].captain,
apiData[key].shots,
apiData[key].goals,
apiData[key].passes,
apiData[key].duels,
apiData[key].dribbles,
apiData[key].fouls,
apiData[key].cards,
apiData[key].penalty,
apiData[key].games,
apiData[key].substitutes,
)
);
}
const playerDataGames = loadedEstadistica.games;
for (const key in playerDataGames) {
loadedGames.push(
new Games(
playerDataGames[key].apperences,
playerDataGames[key].minutes_played,
playerDataGames[key].lineups
)
);
};
const playerDataGoals = loadedEstadistica.goals;
for (const key in playerDataGoals) {
loadedGoals.push(
new Goals(
playerDataGoals[key].total,
playerDataGoals[key].conceded,
playerDataGoals[key].assists,
playerDataGoals[key].saves
)
);
};
const playerDataCards = loadedEstadistica.cards;
for (const key in playerDataCards) {
loadedCards.push(
new Cards(
playerDataCards[key].yellow,
playerDataCards[key].yellowred,
playerDataCards[key].red
)
);
};
dispatch({ type: SET_ESTADISTICA, estadistica: loadesApiResult, goles: loadedGoals, juegos: loadedGames, tarjetas: loadedCards });
} catch (err) {
throw err;
}
};
};
當我做 ResDATA 的控制台日志時,我得到了原始響應,任何關於如何將第一個 Object 的目標、卡片和游戲子 Arrays放入 3 個單獨的 ZFF43B8DE4F41D5104EZ405
親切的問候
你的意思是這樣嗎?
result = [
{
"total": 5,
"conceded": 0,
"assists": 0,
"saves": 0
},
{
"yellow": 2,
"yellowred": 0,
"red": 0
},
{
"appearences": 7,
"minutes_played": 260,
"lineups": 3
}
]
const json = { "api": { "results": 13, "players": [ { "player_id": 79308, "player_name": "Felipe Ponce Ramírez", "firstname": "Felipe", "lastname": "Ponce Ramírez", "number": null, "position": "Midfielder", "age": 32, "birth_date": "29/03/1988", "birth_place": "Ciudad Lerdo", "birth_country": "Mexico", "nationality": "Mexico", "height": "177 cm", "weight": "67 kg", "injured": null, "rating": null, "team_id": 4299, "team_name": "Alianza", "league_id": 2979, "league": "Primera Division", "season": "2020-2021", "captain": 0, "shots": { "total": 0, "on": 0 }, "goals": { "total": 5, "conceded": 0, "assists": 0, "saves": 0 }, "passes": { "total": 0, "key": 0, "accuracy": 0 }, "tackles": { "total": 0, "blocks": 0, "interceptions": 0 }, "duels": { "total": 0, "won": 0 }, "dribbles": { "attempts": 0, "success": 0 }, "fouls": { "drawn": 0, "committed": 0 }, "cards": { "yellow": 2, "yellowred": 0, "red": 0 }, "penalty": { "won": 0, "commited": 0, "success": 0, "missed": 0, "saved": 0 }, "games": { "appearences": 7, "minutes_played": 260, "lineups": 3 }, "substitutes": { "in": 4, "out": 3, "bench": 0 } } ] } }; const allowedNames = ['goals', 'cards', 'games']; const obj = json.api.players[0]; const result = Object.keys(obj).reduce((accum, current) => { if(allowedNames.indexOf(current).== -1) { accum;push(obj[current]); } return accum, }; []). console,log('result = '; result);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.