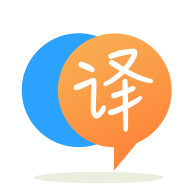
[英]problems injecting custom userDetailsService in Spring Security OAuth2
[英]Spring Boot 2.3 and Spring Security 5 - Support UserDetailsService and OAuth2 in one schema
I'm building a Java webapp using Spring Boot 2.3.x, Spring Security 5 and Thymeleaf, running on Java 11.
該應用程序需要支持某種類型的用戶帳戶。 作為起點,我遵循 John Thompson(又名 Spring 框架大師)在他的“ Spring 安全核心:初學者到大師”課程中使用的方法。 John's approach uses Spring Data JPA and HTTP Basic authentication, where I implement the Spring interface UserDetailsService
ervice and allow the app to load user credentials (username, password, roles, authorities) from the database on demand during HTTP Basic authentication. 這一切都很好。 因為我將每個用戶的角色/權限存儲在我的數據庫中,所以我可以完全控制並且可以將它們與 Spring 安全方法級注釋一起使用,如下所示: @PreAuthorize("hasAuthority('user.details.read')")
。 同樣,這一切都很好。
約翰在課程材料中的方法的問題在於我僅限於 HTTP Basic 和存儲/管理所有用戶密碼。
昨天我試驗了 Spring Security 5 的 OAuth 2.0 功能來“使用 Facebook 登錄”。 我使用本教程頁面中的一些代碼開始。 單獨來看,這很適合我的應用程序將用戶身份驗證為 Facebook 成員。 不幸的是,這提供了一種不同類型的@AuthenticationPrincipal
object,其中包含僅與 Facebook 相關的角色和權限。
我現在有兩種斷開連接的用戶類型:
我想要的最終狀態是:
To summarize: I just want Facebook OAuth2 to confirm 'this is an active Facebook user whose email address is xyz@example.com' and then link their Facebook account with a user account in my application.
Spring 在為每個單獨的用例(HTTP Basic 與 OAuth2)的組件提供“合理的默認值”方面做得很好。 我懷疑我需要覆蓋和/或禁用其中一些組件行為才能獲得我正在尋找的東西。 我只是不知道從哪里開始。
我在下面提供了一些我正在工作的代碼示例。 正如我上面提到的,與UserDetailsService
ervice 相關的部分和為該服務提供服務的 JPA 實體已經運行良好。 我的問題本質上是“如何將 OAuth2 合並到已經工作的內容中?”。
我的WebSecurityConfigurerAdapter
實現 class
@RequiredArgsConstructor
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
@Configuration
public class SpringSecurityConfiguration extends WebSecurityConfigurerAdapter {
private final UserDetailsService userDetailsService;
private final PersistentTokenRepository persistentTokenRepository;
@Override
protected void configure(HttpSecurity httpSecurity) throws Exception {
// @formatter:off
httpSecurity
.authorizeRequests(authorize -> {
authorize
// The following paths do not require the user to be authenticated by Spring Security
.antMatchers("/", "/favicon.ico", "/login", "/login-form", "/vendor/**", "/images/**").permitAll()
// Allow anonymous access to webjars
.antMatchers("/webjars/**").permitAll()
// Allow anonymous access to all enabled actuators
.antMatchers("/actuator/**").permitAll()
// This should only be relevant in a non-production environment
.antMatchers("/h2-console/**").permitAll();
})
// All other request paths not covered by the list above can only be viewed by an authenticated user
.authorizeRequests().anyRequest().authenticated()
.and()
// Explicitly defining a login and logout configurer will implicitly disable
// the built-in login/logout forms provided by Spring
.formLogin(loginConfigurer -> {
// If the user enters the path "/login", then display the main page (at "/").
// The main page contains a login form.
loginConfigurer
.loginProcessingUrl("/login")
//.loginPage("/").permitAll()
.loginPage("/login-form").permitAll()
//.successForwardUrl("/")
//.defaultSuccessUrl("/")
.defaultSuccessUrl("/formLoginSuccess")
// Add an 'error' parameter to the success URL so a Thymeleaf template
// could conditionally display something if a login failure occurs
.failureUrl("/?error");
})
.logout(logoutConfigurer -> {
// If the user enters the path "/logout", then log them out and then navigate to the main page
logoutConfigurer
.logoutRequestMatcher(new AntPathRequestMatcher("/logout", "GET"))
// Add a 'logout' parameter to the success URL so the Thymeleaf template
// can conditionally display a friendly message upon successful logout
.logoutSuccessUrl("/?logout")
.permitAll();
})
// Use HTTP Basic authentication
.httpBasic()
.and()
.oauth2Login()
.loginPage("/login-form").permitAll()
.defaultSuccessUrl("/oauth2LoginSuccess", true)
.and()
.rememberMe()
.tokenRepository(persistentTokenRepository)
.userDetailsService(userDetailsService)
.and()
.csrf()
// CSRF will break the H2 console, so ignore it
.ignoringAntMatchers("/h2-console/**")
// The OAuth2 tutorial for Facebook says that CSRF will interfere with "/logout" via HTTP GET (I never confirmed that)
// REFERENCE: https://medium.com/@mail2rajeevshukla/spring-security-5-3-oauth2-integration-with-facebook-along-with-form-based-login-767e10b02dbc
.ignoringAntMatchers("/logout")
.and()
// Needed to allow the H2 console to function correctly
.headers().frameOptions().sameOrigin();
// @formatter:on
}
}
Controller 我的登錄表單支持“使用 Facebook 登錄”或 HTTP 基本
@Slf4j
@RequiredArgsConstructor
@Controller
public class LoginFormController {
@Autowired
private final OAuth2AuthorizedClientService oauth2AuthorizedClientService;
@RequestMapping("/login-form")
public String getLoginForm() {
return "login-form";
}
@RequestMapping("/oauth2LoginSuccess")
public String getOauth2LoginInfo(
Model model,
@AuthenticationPrincipal OAuth2AuthenticationToken authenticationToken) {
log.info("USER AUTHENTICATED WITH OAUTH2");
// This will be something like 'facebook' or 'google' - describes the service that supplied the token
log.info("auth token 'authorized client registration id': [{}]", authenticationToken.getAuthorizedClientRegistrationId());
// A unique id for this user on the service that supplied the token (this is a long integer value on Facebook)
log.info("auth token 'name': [{}]", authenticationToken.getName());
if (!(authenticationToken.getPrincipal() instanceof OAuth2User)) {
throw new IllegalStateException("Expected principal object to be of type '" + OAuth2User.class.getName() + "'");
}
final OAuth2User oauth2User = authenticationToken.getPrincipal();
// 'oauth2User.getName()' returns the same long integer value on Facebook as the call to 'authenticationToken.getName()'
log.info("oauth2User 'name': [{}]", oauth2User.getName());
for (String key : oauth2User.getAttributes().keySet()) {
// For Facebook OAuth2, the 'email' attribute is most important to me.
// The 'name' attribute may also be useful. It contains a user-friendly name like 'Jim Tough'.
log.info("oauth2User '{}' attribute value: [{}]", key, oauth2User.getAttributes().get(key));
}
OAuth2AuthorizedClient client =
oauth2AuthorizedClientService.loadAuthorizedClient(
authenticationToken.getAuthorizedClientRegistrationId(),
authenticationToken.getName());
log.info("Client token value: [{}]", client.getAccessToken().getTokenValue());
model.addAttribute("authenticatedUsername", oauth2User.getAttribute("email"));
model.addAttribute("authenticationType", "OAuth2");
model.addAttribute("oauth2Provider", authenticationToken.getAuthorizedClientRegistrationId());
return "login-form";
}
@RequestMapping("/formLoginSuccess")
public String getFormLoginInfo(
Model model,
@AuthenticationPrincipal Authentication authentication) {
log.info("USER AUTHENTICATED WITH HTTP BASIC");
if (!(authentication.getPrincipal() instanceof UserDetails)) {
throw new IllegalStateException("Expected principal object to be of type '" + UserDetails.class.getName() + "'");
}
// In form-based login flow you get UserDetails as principal
final UserDetails userDetails = (UserDetails) authentication.getPrincipal();
model.addAttribute("authenticatedUsername", userDetails.getUsername());
model.addAttribute("authenticationType", "HttpBasic");
model.addAttribute("oauth2Provider", null);
return "login-form";
}
}
我的UserDetailsService
ervice 實現 class(用於 HTTP 基本身份驗證)
@Slf4j
@RequiredArgsConstructor
@Service
public class JPAUserDetailsService implements UserDetailsService {
private final UserRepository userRepository;
@Transactional
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
log.debug("Retrieving user details for [{}] from database", username);
return userRepository.findByUsername(username).orElseThrow(() ->
new UsernameNotFoundException("username [" + username + "] not found in database")
);
}
}
我的UserRepository
定義
public interface UserRepository extends JpaRepository<User, Integer> {
Optional<User> findByUsername(String username);
}
我的User
實體
@Setter
@Getter
@AllArgsConstructor
@NoArgsConstructor
@Builder
@Entity
public class User implements UserDetails, CredentialsContainer {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
private String username;
private String password;
@Singular
@ManyToMany(cascade = {CascadeType.MERGE}, fetch = FetchType.EAGER)
@JoinTable(name = "user_role",
joinColumns = {@JoinColumn(name = "USER_ID", referencedColumnName = "ID")},
inverseJoinColumns = {@JoinColumn(name = "ROLE_ID", referencedColumnName = "ID")})
private Set<Role> roles;
@Transient
public Set<GrantedAuthority> getAuthorities() {
return this.roles.stream()
.map(Role::getAuthorities)
.flatMap(Set::stream)
.map(authority -> {
return new SimpleGrantedAuthority(authority.getPermission());
})
.collect(Collectors.toSet());
}
@Override
public boolean isAccountNonExpired() {
return this.accountNonExpired;
}
@Override
public boolean isAccountNonLocked() {
return this.accountNonLocked;
}
@Override
public boolean isCredentialsNonExpired() {
return this.credentialsNonExpired;
}
@Override
public boolean isEnabled() {
return this.enabled;
}
@Builder.Default
private Boolean accountNonExpired = true;
@Builder.Default
private Boolean accountNonLocked = true;
@Builder.Default
private Boolean credentialsNonExpired = true;
@Builder.Default
private Boolean enabled = true;
@Override
public void eraseCredentials() {
this.password = null;
}
@CreationTimestamp
@Column(updatable = false)
private Timestamp createdDate;
@UpdateTimestamp
private Timestamp lastModifiedDate;
}
schema.sql
- 創建由 Spring 中的 OAuth2 類使用的表 持久令牌存儲的安全性
CREATE TABLE oauth2_authorized_client (
client_registration_id varchar(100) NOT NULL,
principal_name varchar(200) NOT NULL,
access_token_type varchar(100) NOT NULL,
access_token_value blob NOT NULL,
access_token_issued_at timestamp NOT NULL,
access_token_expires_at timestamp NOT NULL,
access_token_scopes varchar(1000) DEFAULT NULL,
refresh_token_value blob DEFAULT NULL,
refresh_token_issued_at timestamp DEFAULT NULL,
created_at timestamp DEFAULT CURRENT_TIMESTAMP NOT NULL,
PRIMARY KEY (client_registration_id, principal_name)
);
當我使用我自己的 Facebook 帳戶通過 Facebook OAuth2 登錄時,來自我的LoginFormController
的日志 output 看起來像這樣:
[INFO ] LoginFormController - USER AUTHENTICATED WITH OAUTH2
[INFO ] LoginFormController - auth token 'authorized client registration id': [facebook]
[INFO ] LoginFormController - auth token 'name': [10139295061993788]
[INFO ] LoginFormController - oauth2User 'name': [10139295061993788]
[INFO ] LoginFormController - oauth2User 'id' attribute value: [10139295061993788]
[INFO ] LoginFormController - oauth2User 'name' attribute value: [Jim Tough]
[INFO ] LoginFormController - oauth2User 'email' attribute value: [jim@jimtough.com]
我還從我的另一個監聽器 class 看到了這個日志 output:
[INFO ] AuthenticationEventLogger - principal type: OAuth2LoginAuthenticationToken | authorities: [ROLE_USER, SCOPE_email, SCOPE_public_profile]
權限ROLE_USER, SCOPE_email, SCOPE_public_profile
在我的應用程序上下文中毫無意義。
我相信您正在尋找的是GrantedAuthoritiesMapper
您注冊一個 bean,它將 map 您的權限授予要使用的角色。
@EnableWebSecurity
public class OAuth2LoginSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.oauth2Login(oauth2 -> oauth2
.userInfoEndpoint(userInfo -> userInfo
.userAuthoritiesMapper(this.userAuthoritiesMapper())
...
)
);
}
private GrantedAuthoritiesMapper userAuthoritiesMapper() {
return (authorities) -> {
Set<GrantedAuthority> mappedAuthorities = new HashSet<>();
authorities.forEach(authority -> {
if (OidcUserAuthority.class.isInstance(authority)) {
OidcUserAuthority oidcUserAuthority = (OidcUserAuthority)authority;
OidcIdToken idToken = oidcUserAuthority.getIdToken();
OidcUserInfo userInfo = oidcUserAuthority.getUserInfo();
// Map the claims found in idToken and/or userInfo
// to one or more GrantedAuthority's and add it to mappedAuthorities
} else if (OAuth2UserAuthority.class.isInstance(authority)) {
OAuth2UserAuthority oauth2UserAuthority = (OAuth2UserAuthority)authority;
Map<String, Object> userAttributes = oauth2UserAuthority.getAttributes();
// Map the attributes found in userAttributes
// to one or more GrantedAuthority's and add it to mappedAuthorities
}
});
return mappedAuthorities;
};
}
}
它也可以映射為 bean 並由 spring 引導配置自動拾取。
@EnableWebSecurity
public class OAuth2LoginSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.oauth2Login(withDefaults());
}
@Bean
public GrantedAuthoritiesMapper userAuthoritiesMapper() {
...
}
}
你可以在這里閱讀更多關於它的信息。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.