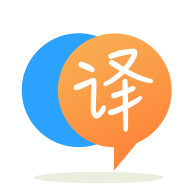
[英]Showing custom dialog made with ComposeView inside Xml based activity - Jetpack Compose - Android
[英]Android: Jetpack Compose and XML in Activity
如何在同一活動中添加 Jetpack Compose 和 xml? 一個例子將是完美的。
如果要在 XML 文件中使用 Compose,可以將其添加到布局文件中:
<androidx.compose.ui.platform.ComposeView
android:id="@+id/my_composable"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
然后,設置內容:
findViewById<ComposeView>(R.id.my_composable).setContent {
MaterialTheme {
Surface {
Text(text = "Hello!")
}
}
}
如果你想要相反,即在你的撰寫中使用 XML 文件,你可以使用這個:
AndroidView(
viewBlock = { context: Context ->
val view = LayoutInflater.from(context)
.inflate(R.layout.my_layout, null, false)
val textView = view.findViewById<TextView>(R.id.text)
// do whatever you want...
view // return the view
},
update = { view ->
// Update the view
}
)
https://developer.android.com/jetpack/compose/interop?hl=en
要嵌入 XML 布局,請使用由
androidx.compose.ui:ui-viewbinding
庫提供的AndroidViewBinding API。 為此,您的項目必須啟用視圖綁定。 AndroidView 與許多其他內置可組合項一樣,采用可用於例如在父可組合項中設置其 position 的 Modifier 參數。
@Composable
fun AndroidViewBindingExample() {
AndroidViewBinding(ExampleLayoutBinding::inflate) {
exampleView.setBackgroundColor(Color.GRAY)
}
}
如果您想像常規View一樣提供可組合組件(能夠在 XML 中指定其屬性),請從AbstractComposeView子類化。
@Composable fun MyComposable(title: String) {
Text(title)
}
// Do not forget these two imports for the delegation (by) to work
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
class MyCustomView @JvmOverloads constructor(
context: Context,
attrs: AttributeSet? = null,
defStyle: Int = 0
) : AbstractComposeView(context, attrs, defStyle) {
var myProperty by mutableStateOf("A string")
init {
// See the footnote
context.withStyledAttributes(attrs, R.styleable.MyStyleable) {
myProperty = getString(R.styleable.MyStyleable_myAttribute)
}
}
// The important part
@Composable override fun Content() {
MyComposable(title = myProperty)
}
}
這就是你將如何使用它就像一個普通的視圖:
<my.package.name.MyCustomView
android:id="@+id/myView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:myAttribute="Helloooooooooo!" />
感謝 ProAndroidDev 提供這篇文章。
要為您的視圖定義您自己的自定義屬性,請參閱這篇文章。
此外,確保使用AndroidX Core庫的-ktx版本,以便能夠訪問有用的 Kotlin 擴展函數,例如Context::withStyledAttributes
:
implementation("androidx.core:core-ktx:1.6.0")
您可以查看此博客以獲取有關互操作的更多詳細信息 API https://blog.yudiz.com/adopting-jetpack-compose-with-interop-api/
更新:當您想在撰寫 function 中使用 XML 文件時
AndroidView(
factory = { context: Context ->
val view = LayoutInflater.from(context)
.inflate(R.layout.test_layout, null, false)
val edittext= view.findViewById<EditText>(R.id.edittext)
view
},
update = { }
)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.