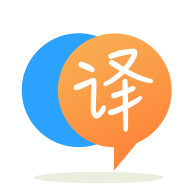
[英]How to only return true when all conditions in for loop are true?
[英]How do you make a conditional statement return true when only one condition is true?
我需要做一個 if 語句,它的值取決於 N 個其他條件。 特別是,只有當N個條件之一為真時才需要返回真(如果多個條件為真,則必須返回假)。 更正式地說, if (1, 2, 3... N)
當且僅當只有一個語句評估為 true 時才評估 true ,否則評估為 false 。
我實現了這個方法,
boolean trueOnce(boolean[] conditions) {
boolean trueOnce = false;
for (boolean condition : conditions) {
if (condition) {
if (!trueOnce) {
trueOnce = true;
} else {
trueOnce = false;
break;
}
}
}
return trueOnce;
}
但我要求更實用的東西。 我正在使用 Java,但我認為這個問題對於每種語言都是通用的。 謝謝。
編輯:這種方法做得很好,但我的問題是,Java(或任何其他語言)是否有更實用的方法(也就是說,甚至沒有實現整個方法)?
使用條件計數true
存在的簡單循環是 go 的簡單方法 return 語句是返回boolean
的比較,是否true
計數正好等於 1:
long count = 0;
for (boolean condition: conditions) {
if (condition) {
count++;
}
}
return count == 1;
這總是迭代並非總是必要的所有數組。 您可以優化迭代以在找到兩個true
值時停止,因此繼續迭代沒有意義。
long count = 0;
for (boolean condition: conditions) {
if (condition && ++count > 1) {
break;
}
}
return count == 1;
你可以使用這個解決方案
public boolean trueOnce(boolean[] conditions) {
boolean m = false;
for(boolean condition : conditions) {
if(m && condition)
return false;
m |= condition;
}
return m;
}
這是一個非常小的解決方案,只需幾行就可以完全滿足您的需求。
使用 Java Stream API:
boolean trueOnce(boolean[] conditions) {
return IntStream.range(0, conditions.length)
.filter(x -> conditions[x]) // leave only `true` values
.limit(2) // no need to get more than two
.count() == 1; // check if there is only one `true` value
}
使用 Stream API,您可以將其重寫為(盡管作為普通循環,它看起來不太“實用”):
import java.util.stream.*;
static boolean trueOnce(boolean ... conditions) {
// find index of first true, if not available get -1
int firstTrue = IntStream.range(0, conditions.length)
.filter(i -> conditions[i])
.findFirst().orElse(-1);
// if first true is found, check if none in the remainder of array is true
return firstTrue > -1 && IntStream.range(firstTrue + 1, conditions.length)
.noneMatch(i -> conditions[i]);
}
使用@VLAZ 建議的更改恢復初始版本:
import java.util.stream.*;
boolean trueOnce(boolean[] conditions) {
return IntStream.range(0, conditions.length)
.filter(i -> conditions[i])
.limit(2) // !
.count() == 1;
}
可以使用 while 運算符執行迭代。 定義一個變量來控制正值的增量,另一個變量來評估數組的每個元素。 這兩個變量將是我們的停止條件,該方法將返回解決方案,當存在單個正值時為 true,在所有其他情況下為 false。
boolean trueOnce(boolean[] conditions)
{
int i = 0, count = 0;
while(count <= 1 && i < conditions.length){
if(conditions[i++]){
count++;
}
}
return count == 1;
}
或按照建議,我們可以使用:
private static boolean trueOnce(boolean[] conditions)
{
int count = 0;
for(int i = 0; i < conditions.length && count <= 1; i++){
if(conditions[i]){
count++;
}
}
return count == 1;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.