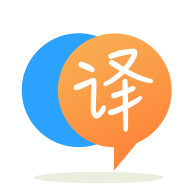
[英]How to code a custom Comparator to sort a Treeset with SimpleEntry?
[英]How to sort and avoid duplicates in TreeSet with my comparator?
我想創建TreeSet()
,它將使用我預定義的比較器對我的元素進行排序。 但問題是,當我將比較器作為參數提供給TreeSet(MyComparator)
的構造函數時,TreeSet 並沒有避免重復。 我可以實現元素的排序並避免重復嗎?
比較器看起來像:
public static Comparator<Participant> byNameAndAge = (L, R) -> {
//check if they have the same code
if (L.code.equalsIgnoreCase(R.code))
return 0;
int res = L.name.compareToIgnoreCase(R.name);
if (res == 0)
res = Integer.compare(L.age, R.age);
return res;
};
你誤解了一些事情。 TreeSet 確實消除了重復項,“重復項”定義為“比較方法返回 0 的任何兩個元素”。 沒有 2 個這樣的元素可以同時存在於樹集中。 如果您這么說,我確定您的代碼不起作用,但是您粘貼的代碼不是問題,TreeSet 的代碼也不是。
一個簡單的例子:
Comparator<String> byLength = (a, b) -> a.length() - b.length();
Set<String> set = new TreeSet<String>(byLength);
set.add("Hello");
set.add("World");
set.add("X");
set.add("VeryLong");
System.out.println(set);
> [X, Hello, VeryLong]
請注意“世界”是如何消失的,因為比較器說它等於 Hello(它們都是 5 長度,a.length() - b.length() 返回 0,而 0 是“相等,因此,消除重復”根據樹集)。 換句話說,您粘貼的代碼將消除重復,問題出在其他地方。
此代碼與您的代碼幾乎相同。
public static void main(String[] args) {
// custom comparator
Comparator<Participant> byNameAndAge = Comparator
// first sorting by name ignoring case
.comparing(Participant::getName, String::compareToIgnoreCase)
// second sorting by age
.thenComparingInt(Participant::getAge);
// constructor with a comparator as a parameter
TreeSet<Participant> treeSet = new TreeSet<>(byNameAndAge);
treeSet.addAll(Set.of( // test data
new Participant("John", 25),
new Participant("Junior", 2),
new Participant("james", 31),
new Participant("john", 22)));
// output
treeSet.forEach(System.out::println);
//name=james, age=31
//name=john, age=22
//name=John, age=25
//name=Junior, age=2
}
static class Participant {
String name;
int age;
public Participant(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() { return name; }
public int getAge() { return age; }
@Override
public String toString() {
return "name=" + name + ", age=" + age;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.