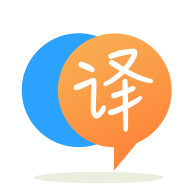
[英]Is it possible, using jquery, to target all elements of a specific class within the parent div of the button that calls the event?
[英]Select elements within target div (row) on event jQuery
我有一個表,我需要 select 使用jQuery
在同一行中完成事件(在本例中是keyup
事件)之后的所有行的值。 該表可能有很多行,但選擇必須在行事件的項目上。
這是表的 HTML:
<table class="table" id="form-table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Description</th>
<th scope="col">Qty</th>
<th scope="col">Price</th>
<th scope="col">Discount</th>
<th scope="col">Amount</th>
<th scope="col">Hidden</th>
</tr>
</thead>
<tbody>
<tr id="row-1">
<th scope="row">1</th>
<td class="description-column">
<div class="form-group">
<select class="form-control" id="companySelect">
{% for product in product_options %}
<option>{{ product }}</option>
{% endfor %}
</select>
</div>
</td>
<td class="qty-column">
<div class="form-group">
<input type="number" class="form-control" id="qty" placeholder="Qty" min="0" step="1">
</div>
</td>
<td class="price-column">
<div class="form-group">
<input type="number" class="form-control" id="price" min="0.01" placeholder="Price">
</div>
</td>
<td class="discount-column">
<div class="form-group">
<input type="number" class="form-control" id="discount" placeholder="0" min="0.01" max="99.99">
</div>
<div>%</div>
</td>
<td class="amount-column"></td>
<td class="hidden-column">
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="hiddenCheck">
</div>
</td>
</tr>
</tbody>
</table>
在網站上看起來像這樣:
有關更多上下文:我正在制作一個報價應用程序,我將在其中輸入每行中每個項目的價格和數量,並且我需要在每個keyup
之后更新“金額”列。 所以我需要知道如何 select 正確的“數量”、“價格”、“折扣”和“金額”ID 的輸入行來做到這一點。
首先, id
屬性是唯一的,所以你應該像這樣用class
替換它
<input type="number" class="form-control qty" placeholder="Qty" min="0" step="1">
其次,您可以像這樣使用.closest()
來獲得tr
$(this).closest("tr")
最后,您可以通過創建另一個名為updateAmount_per_row
的方法來計算單個行,如下所示:
function updateAmount_per_row(tr){
var qTy = getValue_by_className(tr, '.qty-column .qty');
var price = getValue_by_className(tr, '.price-column .price');
var amount = qTy * price;
tr.find('.amount-column').html(amount);
}
$(".qty-column, .price-column").on("keyup", function(){ var tr = $(this).closest("tr"); updateAmount_per_row(tr); }); function updateAmount_per_row(tr){ var qTy = getValue_by_className(tr, '.qty-column.qty'); var price = getValue_by_className(tr, '.price-column.price'); var amount = qTy * price; tr.find('.amount-column').html(amount); } function getValue_by_className(tr, className){ var value = parseInt(tr.find(className).val(), 10); return value >= 0? value: 1; }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <table class="table" id="form-table"> <thead> <tr> <th scope="col">#</th> <th scope="col">Description</th> <th scope="col">Qty</th> <th scope="col">Price</th> <th scope="col">Discount</th> <th scope="col">Amount</th> <th scope="col">Hidden</th> </tr> </thead> <tbody> <tr id="row-1"> <th scope="row">1</th> <td class="description-column"> <div class="form-group"> <select class="form-control" class="companySelect"> <option>Product A</option> <option>Product B</option> </select> </div> </td> <td class="qty-column"> <div class="form-group"> <input type="number" class="form-control qty" placeholder="Qty" min="0" step="1"> </div> </td> <td class="price-column"> <div class="form-group"> <input type="number" class="form-control price" min="0.01" placeholder="Price"> </div> </td> <td class="discount-column"> <div class="form-group"> <input type="number" class="form-control" class="discount" placeholder="0" min="0.01" max="99.99"> </div> <div>%</div> </td> <td class="amount-column"></td> <td class="hidden-column"> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="hiddenCheck"> </div> </td> </tr> <tr id="row-2"> <th scope="row">2</th> <td class="description-column"> <div class="form-group"> <select class="form-control" class="companySelect"> <option>Product A</option> <option>Product B</option> </select> </div> </td> <td class="qty-column"> <div class="form-group"> <input type="number" class="form-control qty" placeholder="Qty" min="0" step="1"> </div> </td> <td class="price-column"> <div class="form-group"> <input type="number" class="form-control price" min="0.01" placeholder="Price"> </div> </td> <td class="discount-column"> <div class="form-group"> <input type="number" class="form-control" class="discount" placeholder="0" min="0.01" max="99.99"> </div> <div>%</div> </td> <td class="amount-column"></td> <td class="hidden-column"> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="hiddenCheck"> </div> </td> </tr> </tbody> </table>
使用Mai Phuong答案作為測試,我設法通過使用target
屬性而不是this
來使其工作,因為出於某種原因, this
引用了整個文檔( #document
)。
這是keyup
事件監聽器:
$('.price, .qty, .discount').keyup((event) => {
let tr = event.target.closest('tr');
updateAmount(tr);
});
這是最終的updateAmount
function:
function updateAmount(tr) {
let qty = $(tr).find('.qty').val();
let price = $(tr).find('.price').val();
let discount = $(tr).find('.discount').val();
let amount = (qty * price * (100 - discount)) / 100;
$(tr).find('.amount').html(amount);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.