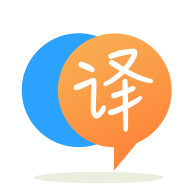
[英]Cannot make unique javascript object. What's wrong with this code?
[英]How to Link a CSS Object to a JavaScript Object. (In code, not in files.)
基本上,我試圖在我的 CSS 中獲得一個 object 來模仿我的 object 在我的 Z9E13B69D1D2DA9327102 代碼中的坐標。 I have the javascript object being the piece everyone will see, while when I need an animation to play I make the JavaScript Object invisible, and put an identical CSS Object in its place with an animation attached to it. Then, When the animation is done, I need the CSS Object to disappear and the JavaScript Object to Reappear. 如果我聽起來像個徹頭徹尾的白痴,我很抱歉。 如果您有更好的方法來交換它們甚至處理代碼,請告訴我。 我還想讓所有代碼相關,而不是將外部源/附加組件帶入程序。 如果您需要使用 HTML,那也可以。
TL;DR 基本上我希望 CSS 對象的坐標來模仿 JavaScript 坐標。
注意:代碼只是基本的東西,而不是來自我的實際游戲。 請與一粒鹽一起服用。 隨心所欲地塑造它。
Box = {
x: 20,
y: 30,
width: 100,
height: 200
}
#Box {
left: 4px;
top: 2px;
@keyframes Box {
0% {transform: rotate(22.5deg);}
12.5% {transform: rotate(33.75deg);}
25% {transform: rotate(45deg);}
37.5% {transform: rotate(56.25deg);}
50% {transform: rotate(67.5eg);}
62.5% {transform: rotate(78.75deg);}
75% {transform: rotate(90deg);}
87.5% {transform: rotate(101.25deg);}
100% {transform: rotate(135deg);}
}
不確定是否完全理解,但是:
如果您有此 html 元素:
<div id="box"></div>
然后是它的 CSS:
#Box {
left: 4px;
top: 2px;
@keyframes Box {
0% {transform: rotate(22.5deg);}
12.5% {transform: rotate(33.75deg);}
25% {transform: rotate(45deg);}
37.5% {transform: rotate(56.25deg);}
50% {transform: rotate(67.5eg);}
62.5% {transform: rotate(78.75deg);}
75% {transform: rotate(90deg);}
87.5% {transform: rotate(101.25deg);}
100% {transform: rotate(135deg);}
}
最后在 JS
Box = {
x: 20,
y: 30,
width: 100,
height: 200
};
document.getElementById('box').style.marginLeft = Box.x;
...
document.getElementById('box').addEventListener("webkitAnimationEnd",function(){
document.getElementById('box').style.marginLeft = 'initial';
});
如果您正在談論兩個出現並背靠背消失的框,則可以通過 CSS 完成。 將您的 animation 問題想象為在關鍵幀中以不同的不透明度(透明度)移動的 2 個框。
.BOX_1{ position:absolute; z-index:0; animation-name: Box1; animation-duration: 2s; animation-delay:100ms; animation-iteration-count: infinite; }.BOX_2{ position:absolute; z-index:0; animation-name: Box2; animation-duration: 2s; animation-delay:100ms; animation-iteration-count: infinite; } @keyframes Box1 { 0% {transform: rotate(22.5deg);opacity:0;} 25% {transform: rotate(45deg);opacity:0.25;} 50% {transform: rotate(67.5eg);opacity:0.5;} 75% {transform: rotate(90deg);opacity:0.75;} 100% {transform: rotate(135deg);opacity:1;} } @keyframes Box2 { 0% {transform: rotate(22.5deg);opacity:1;} 25% {transform: rotate(45deg);opacity:0.75;} 50% {transform: rotate(67.5eg);opacity:0.5;} 75% {transform: rotate(90deg);opacity:0.25;} 100% {transform: rotate(135deg);opacity:0;} }
在此處查看有關 css 動畫的這篇文章,並根據您的要求調整不透明度等屬性您可以將 JS 庫用於非常復雜的動畫
聽起來您想使用 JS 技巧來更改界面(HTML 和 CSS)的 state。 幸運的是,您有幾個選擇。
生成“盒子”的 JS 將創建 HTML。 將 class styles 應用於 HTML 實體,並在需要時添加/刪除它們:
CSS:
.hide {
display: hidden;
}
.show {
display: initial;
}
HTML:
<box id='mybox' class='show' />
還有一些基本的 JS 來隱藏它:
$('#mybox')[0].classList.replace('show', 'hide')
但是您始終可以只更改目標節點styles
:
var el = document.getElementById(id);
el.style.color = "red";
el.style.fontSize = "15px";
el.style.backgroundColor = "#FFFFFF";
或者,您可以發明一個新的樣式表:
var sheet = document.createElement('style')
sheet.innerHTML = "div {border: 2px solid black; background-color: blue;}";
document.body.appendChild(sheet);
並更改現有樣式表:
stylesheet.insertRule(".have-border { border: 1px solid black;}", 0);
它可以更深入地 go,用 JS 操作 CSS 的聲明。
var styleObj = document.styleSheets[0].cssRules[0].style;
console.log(styleObj.cssText);
for (var i = styleObj.length; i--;) {
var nameString = styleObj[i];
styleObj.removeProperty(nameString);
}
console.log(styleObj.cssText);
它允許您將文字元素寫入單個規則表:
const rules = document.createElement('span').style;
rules.backgroundColor = 'red';
rules.cursor = 'pointer';
rules.color = 'white';
document.getElementById('style_string').innerHTML = rules.cssText;
document.getElementById('obj').style = rules.cssText;
或者把它和一些美味的東西混合起來:
const box = document.querySelector("#mybox")
const rules = {
color: '#369',
width: '100px',
}
Object.assign(box.style, rules)
利用現代 CSS 語法,您可以在工作表中寫入“--vars”,然后使用 JS 操作這些變量:
:root {
--text-color: black;
--background-color: white;
}
body {
color: var(--text-color);
background: var(--background-color);
}
var bodyStyles = document.body.style;
bodyStyles.setProperty('--text-color', 'white');
bodyStyles.setProperty('--background-color', 'black');
但它並不止於此,您可能會找到替代方法來實現相同的結果。
參考資料和例子:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.