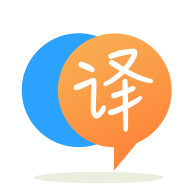
[英]Create React App changes behaviour of jest.fn() when mocking async function
[英]Mocking fetch with jest.fn() in React
我想知道為什么我需要在我的測試中放入 fetch 模擬邏輯以使其工作。
這是一個簡單的例子:
在 useEffect 中使用 fetch 進行測試的組件和 state 在響應后更新:
// Test.jsx
import React, {useEffect, useState} from 'react'
export const Test = () => {
const [description, setDescription] = useState<string | null>(null)
const fetchData = async () => {
const response = await fetch('https://dummyendpoint/');
const parsed = await response.json();
const description = parsed.value;
setDescription(description);
}
useEffect(() => {
fetchData();
}, [])
return (
<div data-testid="description">
{description}
</div>
)
};
export default Test;
測試邏輯:
// Test.test.js
import React from 'react';
import {render, screen} from '@testing-library/react';
import Test from "./Test";
global.fetch = jest.fn(() => Promise.resolve({
json: () => Promise.resolve({
value: "Testing something!"
})
}));
describe("Test", () => {
it('Should have proper description after data fetch', async () => {
// need to put mock logic here to make it work
render(<Test/>);
const description = await screen.findByTestId('description');
expect(description.textContent).toBe("Testing something!");
});
})
如果我將 global.fetch mock 保留在測試文件的頂部,我會不斷收到錯誤消息:
TypeError: Cannot read property 'json' of undefined
at const parsed = await response.json();
它不能按原樣工作,這真的很奇怪。
但是我能夠通過將設置移動到beforeEach
塊來修復它(我假設beforeAll
也可以工作)。
備份全局變量值,覆蓋它以進行測試並將其恢復是一種常見的模式。
import React from 'react';
import { render, screen } from '@testing-library/react';
import Test from "./Test";
describe("Test", () => {
let originalFetch;
beforeEach(() => {
originalFetch = global.fetch;
global.fetch = jest.fn(() => Promise.resolve({
json: () => Promise.resolve({
value: "Testing something!"
})
}));
});
afterEach(() => {
global.fetch = originalFetch;
});
it('Should have proper description after data fetch', async () => {
// need to put mock logic here to make it work
render(<Test />);
const description = await screen.findByTestId('description');
expect(description.textContent).toBe("Testing something!");
});
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.