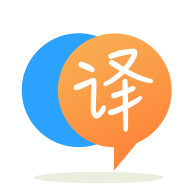
[英]Why am I getting java.lang.StringIndexOutOfBoundsException?
[英]Why am I getting a java.lang.StringIndexOutOfBoundsException error when I run?
我正在學習 Java,我們有一個項目來制作一個將文本轉換為 ASCII 並返回的程序。
到目前為止我的主要方法是
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
char decisionOne;
System.out.println("Are we making a new message or decrypting an old one?");
decisionOne = s.nextLine().charAt(0);
switch(decisionOne) {
case 'd':
decrypt();
System.exit(0);
break;
case 'D':
decrypt();
System.exit(0);
break;
case 'E':
encrypt();
System.exit(0);
break;
case 'e':
encrypt();
System.exit(0);
break;
}
while (!(decisionOne == 'd') && !(decisionOne == 'D') && !(decisionOne == 'e') && !(decisionOne == 'E')) {
System.out.println("Hey! Choose one of them.");
decisionOne = s.nextLine().charAt(0);
switch(decisionOne) {
case 'd':
decrypt();
System.exit(0);
break;
case 'D':
decrypt();
System.exit(0);
break;
case 'E':
encrypt();
System.exit(0);
break;
case 'e':
encrypt();
System.exit(0);
break;
}
}
}
我加密成ASCII的方法是
Scanner s = new Scanner(System.in);
System.out.println("What is your message?");
String message = s.nextLine();
char m;
int length = message.length();
int tracker = 0;
int ascii;
while (length >= 0 ) {
m = message.charAt(tracker);
length--;
ascii = (int)m;
System.out.print(ascii + " ");
tracker ++;
}
}
我環顧了其他問題,但似乎都沒有回答這里發生的事情。 當我運行時,我得到了正確的 output,所以如果我輸入
11
我會得到
49 49 線程“main”中的異常 java.lang.StringIndexOutOfBoundsException:字符串索引超出范圍:2
我能做些什么來解決這個問題?
你想要while (length > 0)
或while (tracker < length)
或者您可以只執行for (Character m: message.toCharArray())
並放棄長度、跟蹤器和 charAt 方法
我把它放在你的加密方法中,然后..
m = message.charAt(tracker);
System.out.print(length + " ");
length--;
ascii = (int)m;
System.out.print(ascii + " ");
tracker ++;
System.out.print(length + " "+tracker+" ");
output 我得到的是這個...
2 49 1 1 1 49 0 2
您在最后看到您的 tracker=2 超出了您的消息字符串的范圍,這就是您遇到異常的原因,您可以處理異常或改進您的代碼......個人經驗 - 如果您是初學者,請使用 for 循環它會讓你更清楚代碼是如何工作的
將加密方法中的代碼從while(length >= 0)
更改為while(length - 1 >= 0)
。 使用后還要關閉掃描儀 object,因為它會導致 memory 泄漏。
length() 方法返回字符串的長度,(eg) String message = "Hello"; length = message.length();
String message = "Hello"; length = message.length();
length 將等於 5。但是在 while 循環中使用它時,您必須使用 message.length() - 1 因為在 Java 中,索引從 0 而不是 1 開始。
我重構了你的 encypt 方法和 main 方法,而且我覺得沒有必要使用 switch 語句。 用 if else 語句替換它們以減少冗余代碼。
如果您發現此解決方案有用,請支持該解決方案,謝謝。
public static void encrypt() {
Scanner s = new Scanner(System.in);
System.out.println("What is your message?");
String message = s.nextLine();
char m;
int length = message.length();
int tracker = 0;
int ascii;
while (length - 1 >= 0 ) {
m = message.charAt(tracker);
length--;
ascii = (int)m;
System.out.print(ascii + " ");
tracker++;
}
s.close();
}
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
char decisionOne;
System.out.println("Are we making a new message [e (or) E] or decrypting an old one [d (or) D] ?");
decisionOne = s.nextLine().charAt(0);
if (decisionOne == 'd' || decisionOne == 'D') {
decrypt();
System.exit(0);
}
else if (decisionOne == 'e' || decisionOne == 'E') {
encrypt();
System.exit(0);
}
while (!(decisionOne == 'd') && !(decisionOne == 'D') && !(decisionOne == 'e') && !(decisionOne == 'E')) {
System.out.println("Hey! Choose one of them.");
decisionOne = s.nextLine().charAt(0);
if (decisionOne == 'd' || decisionOne == 'D') {
decrypt();
System.exit(0);
}
else if (decisionOne == 'e' || decisionOne == 'E') {
encrypt();
System.exit(0);
}
}
s.close();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.