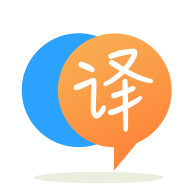
[英]how to Receive UDP packets, when it comes from server? for android java
[英]When we receive packets from an UDP server, why do we have to receive them in a seperate thread?
所以我的應用程序非常簡單。 如果您通過掃描儀鍵入某些內容,它會將其發送到服務器,然后服務器將其發送回客戶端。 但是,我不明白為什么我們必須將處理從服務器接收數據包的代碼放入線程中?
下面的代碼工作正常,但如果我不使用使用多線程,那么應用程序將無法工作。 我發送數據包的部分也停止工作。 你能解釋一下為什么會這樣嗎?
public class Client {
private static DatagramSocket socket = null;
public static void main(String[] args) {
System.out.println("Send to server:");
Scanner scanner = new Scanner(System.in);
while (true) {
try {
// port shoudn't be the same as in TCP but the port in the datagram packet must
// be the same!
socket = new DatagramSocket();
} catch (SocketException e1) {
System.out.println("[CLIENT] Unable to initiate DatagramSocket");
}
InetAddress ip = null;
try {
ip = InetAddress.getByName("127.0.0.1");
} catch (UnknownHostException e) {
System.out.println("[CLIENT] Unable to determine server IP");
}
// must be in a while loop so we can continuously send messages to server
String message = null;
Thread thread = new Thread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
receive();
}
});
thread.start();
while (scanner.hasNextLine()) {
message = scanner.nextLine();
byte[] buffer = message.getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, ip, 6066);
try {
socket.send(packet);
} catch (IOException e) {
System.out.println("[CLIENT] Unable to send packet to server");
}
}
}
}
private static void receive() {
// receiving from server
byte[] buffer2 = new byte[100];
DatagramPacket ps = new DatagramPacket(buffer2, buffer2.length);
while (true) {
try {
socket.receive(ps);
} catch (IOException e) {
System.out.println("[CLIENT] Unable to receive packets from server.");
}
System.out.println("[SERVER] " + new String(ps.getData()));
}
}
}
如果您通過掃描儀鍵入某些內容,它會將其發送到服務器,然后服務器將其發送回客戶端。
所以 main 方法在主線程上運行並完成一些工作。 你剛才提到的工作。
閱讀一些用戶輸入以及以下部分
while (scanner.hasNextLine()) {
message = scanner.nextLine();
byte[] buffer = message.getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, ip, 6066);
try {
socket.send(packet);
} catch (IOException e) {
System.out.println("[CLIENT] Unable to send packet to server");
}
}
標題:從 UDP 服務器接收數據包
您想接收數據包,但又不想阻止用戶輸入某些內容作為輸入並將其發送到服務器。
因此,您需要同時做 2 份工作。 又名多線程
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.