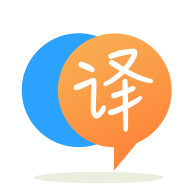
[英]Can't get controls to display on react leaflet mapContainer
[英]React Leaflet display legend using MapContainer
我正在使用 React leaflet 3.1.0,我想在 map 中顯示一個圖例。 我為圖例構建了一個組件,並傳遞了我從 MapContainer whenCreated({setMap}) 獲得的 map 實例。
Map 組件:
import { useState } from 'react';
import { MapContainer, TileLayer } from 'react-leaflet';
import './Map.css';
import Legend from './Legend/Legend';
const INITIAL_MAP_CONFIG = {center: [41.98311,2.82493], zoom: 14}
function Map() {
const [map, setMap] = useState(null);
return (
<MapContainer center={INITIAL_MAP_CONFIG.center} zoom={INITIAL_MAP_CONFIG.zoom} scrollWheelZoom={true} whenCreated={setMap}>
<TileLayer
attribution='© <a href="http://osm.org/copyright">OpenStreetMap</a> contributors'
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
/>
<Legend map={map} />
</MapContainer>
)
}
export default Map
圖例組件:
import { useEffect } from 'react';
import L from 'leaflet';
function Legend({ map }) {
useEffect(() => {
if (map){
const legend = L.control({ position: "bottomright" });
legend.onAdd = () => {
const div = L.DomUtil.create('div', 'info legend');
div.innerHTML =
'<h4>This is the legend</h4>' +
'<b>Lorem ipsum dolor sit amet consectetur adipiscing</b>';
return div;
}
legend.addTo(map);
}
},[]);
return null
}
export default Legend
Map CSS:
.leaflet-container {
width: 100vw;
height: 100vh;
}
圖例 CSS:
.info {
padding: 6px 8px;
font: 14px/16px Arial, Helvetica, sans-serif;
background: white;
border-radius: 5px;
}
.legend {
text-align: left;
line-height: 18px;
color: #555;
}
我沒有收到任何錯誤,但看不到圖例。
嘗試將 map 實例放在依賴數組中。 在第一個渲染中,map 是 null,因此無法將圖例添加到 map。
function Legend({ map }) {
console.log(map);
useEffect(() => {
if (map) {
const legend = L.control({ position: "bottomright" });
legend.onAdd = () => {
const div = L.DomUtil.create("div", "info legend");
div.innerHTML =
"<h4>This is the legend</h4>" +
"<b>Lorem ipsum dolor sit amet consectetur adipiscing</b>";
return div;
};
legend.addTo(map);
}
}, [map]); //here add map
return null;
}
您可以使用標記的圖標屬性並作為道具 Leaflet 圖標類型傳遞。
import React from "react";
import L from "leaflet";
import {
MapContainer,
TileLayer,
Marker,
Popup,
} from "react-leaflet";
const CENTER =
{
lat: 50.8210849,
lng: 19.1115524,
};
const customIcon = L.icon({
iconUrl: require("assets/images/icon.jpeg"),
iconSize: [35, 35],
});
export default function Maps() {
const mapRef = React.useRef(null);
return (
<MapContainer
center={CENTER}
zoom={5}
scrollWheelZoom={true}
style={{ width: "100%", height: 500 }}
ref={mapRef}
>
<TileLayer
attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
/>
<Marker position={CENTER} icon={customIcon}>
<Popup>
A pretty CSS3 popup. <br /> Easily customizable.
</Popup>
</Marker>
</MapContainer>
);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.