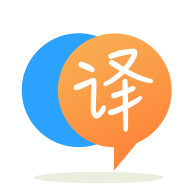
[英]Cannot read property 'navigate' of undefined - React Native Navigation
[英]React Native Cannot read property 'navigate' of undefined
我想在我的主屏幕上設置 2 個可觸摸的不透明度,一個用於導航到創建屏幕,一個用於導航到搜索屏幕。 我的選項卡導航中嵌套了堆棧導航,我的主頁選項卡顯示了主頁堆棧等。每當我觸摸我的搜索或創建按鈕時,它都會顯示一個屏幕,顯示“無法讀取未定義的屬性‘導航’”
import React from "react";
import {
View,
StyleSheet,
Text,
ImageBackground,
Image,
Button,
TouchableOpacity,
} from "react-native";
import { LinearGradient } from "expo-linear-gradient";
import colors from "../config/colors";
import { withNavigation } from "react-navigation";
export default function HomePageScreen({ navigation }) {
return (
<ImageBackground
style={styles.background}
source={require("../assets/background.jpg")}
>
<Image style={styles.logo} source={require("../assets/logo2.png")} />
{/* the create button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.createButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Create Project")}
>
<Text style={styles.buttonText}>Create</Text>
</TouchableOpacity>
</LinearGradient>
{/* the search button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.searchButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Search Projects")}
>
<Text style={styles.buttonText}>Search</Text>
</TouchableOpacity>
</LinearGradient>
</ImageBackground>
);
}
const styles = StyleSheet.create({
background: {
width: "100%",
height: "100%",
alignItems: "center",
justifyContent: "center",
},
logo: {
height: 275,
width: 275,
position: "absolute",
top: 60,
},
createButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 150,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
touch: {
height: 70,
width: 325,
borderRadius: 999,
textAlignVertical: "center",
justifyContent: "center",
},
searchButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 50,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
buttonText: {
color: "white",
fontSize: 28,
fontWeight: "bold",
alignSelf: "center",
},
});
我試圖在我的主屏幕上設置 2 個可觸摸的不透明度,一個用於導航到創建屏幕,一個用於導航到搜索屏幕。 我的標簽導航中嵌套了堆棧導航,我的主頁選項卡會調出主頁堆棧等。每當我觸摸我的搜索或創建按鈕時,它都會彈出一個屏幕,上面寫着“無法讀取未定義的屬性'導航'”
import React from "react";
import {
View,
StyleSheet,
Text,
ImageBackground,
Image,
Button,
TouchableOpacity,
} from "react-native";
import { LinearGradient } from "expo-linear-gradient";
import colors from "../config/colors";
import { withNavigation } from "react-navigation";
export default function HomePageScreen({ navigation }) {
return (
<ImageBackground
style={styles.background}
source={require("../assets/background.jpg")}
>
<Image style={styles.logo} source={require("../assets/logo2.png")} />
{/* the create button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.createButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Create Project")}
>
<Text style={styles.buttonText}>Create</Text>
</TouchableOpacity>
</LinearGradient>
{/* the search button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.searchButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Search Projects")}
>
<Text style={styles.buttonText}>Search</Text>
</TouchableOpacity>
</LinearGradient>
</ImageBackground>
);
}
const styles = StyleSheet.create({
background: {
width: "100%",
height: "100%",
alignItems: "center",
justifyContent: "center",
},
logo: {
height: 275,
width: 275,
position: "absolute",
top: 60,
},
createButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 150,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
touch: {
height: 70,
width: 325,
borderRadius: 999,
textAlignVertical: "center",
justifyContent: "center",
},
searchButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 50,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
buttonText: {
color: "white",
fontSize: 28,
fontWeight: "bold",
alignSelf: "center",
},
});
您應該使用 withNavigation 包裝您的組件以訪問導航
import React from "react";
import {
View,
StyleSheet,
Text,
ImageBackground,
Image,
Button,
TouchableOpacity,
} from "react-native";
import { LinearGradient } from "expo-linear-gradient";
import colors from "../config/colors";
import { withNavigation } from "react-navigation";
function HomePageScreen({ navigation }) {
return (
<ImageBackground
style={styles.background}
source={require("../assets/background.jpg")}
>
<Image style={styles.logo} source={require("../assets/logo2.png")} />
{/* the create button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.createButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Create Project")}
>
<Text style={styles.buttonText}>Create</Text>
</TouchableOpacity>
</LinearGradient>
{/* the search button */}
<LinearGradient
colors={[colors.primary, colors.secondary]}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 0 }}
style={styles.searchButton}
>
<TouchableOpacity
style={styles.touch}
onPress={() => navigation.navigate("Search Projects")}
>
<Text style={styles.buttonText}>Search</Text>
</TouchableOpacity>
</LinearGradient>
</ImageBackground>
);
}
const styles = StyleSheet.create({
background: {
width: "100%",
height: "100%",
alignItems: "center",
justifyContent: "center",
},
logo: {
height: 275,
width: 275,
position: "absolute",
top: 60,
},
createButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 150,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
touch: {
height: 70,
width: 325,
borderRadius: 999,
textAlignVertical: "center",
justifyContent: "center",
},
searchButton: {
height: 70,
width: 325,
position: "absolute",
bottom: 50,
borderRadius: 999,
alignItems: "center",
justifyContent: "center",
},
buttonText: {
color: "white",
fontSize: 28,
fontWeight: "bold",
alignSelf: "center",
},
});
export default withNavigation(HomePageScreen)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.