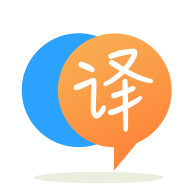
[英]error handler is getting called twice angular http_interceptor
[英]HTTP_INTERCEPTOR not called in production mode (heroku)
我在使用 HTTP_INTERCEPTORS 配置的 heroku 上部署的應用程序有問題。 應用程序后端位於 Spring 框架中並部署在另一個 heroku 存儲庫中,而前端是 Angular。
攔截器用於攔截請求並刷新header中的jwtTokens。
我寫了一個簡單的 console.log("httpRequest intercepted", req) 來檢查是否觸發了攔截方法。
對我來說奇怪的是,它在本地啟動時被正確調用,但在 heroku 上卻沒有。 因此 jwtToken 永遠不會刷新和過期。
其他功能(登錄、注冊、連接到數據庫等)正常工作。
你能看到我看不到的東西嗎?
令牌攔截器.ts
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent, HttpErrorResponse } from '@angular/common/http';
import { Observable, BehaviorSubject, throwError } from 'rxjs';
import { AuthService } from './auth/shared/auth.service';
import { catchError, switchMap, take, filter } from 'rxjs/operators';
import { LoginResponse } from './auth/login/login-response.payload';
@Injectable({
providedIn: 'root'
})
export class TokenInterceptor implements HttpInterceptor {
isTokenRefreshing = false;
refreshTokenSubject: BehaviorSubject<any> = new BehaviorSubject(null);
constructor(public authService: AuthService) { }
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
console.log("httpRequest intercepted", req)
if (req.url.indexOf('refresh') !== -1 || req.url.indexOf('login') !== -1) {
return next.handle(req);
}
const jwtToken = this.authService.getJwtToken();
if (jwtToken) {
return next.handle(this.addToken(req, jwtToken)).pipe(catchError(error => {
if (error instanceof HttpErrorResponse
&& error.status === 403) {
return this.handleAuthErrors(req, next);
} else {
return throwError(error);
}
}));
}
return next.handle(req);
}
private handleAuthErrors(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
if (!this.isTokenRefreshing) {
this.isTokenRefreshing = true;
this.refreshTokenSubject.next(null);
return this.authService.refreshToken().pipe(
switchMap((refreshTokenResponse: LoginResponse) => {
this.isTokenRefreshing = false;
this.refreshTokenSubject
.next(refreshTokenResponse.authenticationToken);
return next.handle(this.addToken(req,
refreshTokenResponse.authenticationToken));
})
)
} else {
return this.refreshTokenSubject.pipe(
filter(result => result !== null),
take(1),
switchMap((res) => {
return next.handle(this.addToken(req,
this.authService.getJwtToken()))
})
);
}
}
addToken(req: HttpRequest<any>, jwtToken: any) {
return req.clone({
headers: req.headers.set('Authorization',
'Bearer ' + jwtToken)
});
}
}
Header.component.ts
import { Component, OnInit } from '@angular/core';
import {AuthService} from '../auth/shared/auth.service';
import {Router} from '@angular/router';
@Component({
selector: 'app-header',
templateUrl: './header.component.html',
styleUrls: ['./header.component.css']
})
export class HeaderComponent implements OnInit {
isLoggedIn: boolean;
username: string;
constructor(private authService : AuthService, private router : Router){ }
ngOnInit(): void {
this.authService.loggedIn.subscribe((data: boolean) => this.isLoggedIn = data);
this.authService.username.subscribe((data: string) => this.username = data);
this.isLoggedIn = this.authService.isLoggedIn();
this.username = this.authService.getUserName();
console.log('header', this.isLoggedIn);
}
logout() {
this.authService.logout();
this.isLoggedIn = false;
this.router.navigateByUrl('');
}
}
auth.service.ts
import {EventEmitter, Injectable, Output} from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { SignupRequestPayload } from '../sign-up/signup-request.payload';
import {Observable, throwError} from 'rxjs';
import { LocalStorageService } from 'ngx-webstorage';
import { LoginRequestPayload } from '../login/login-request.payload';
import { LoginResponse } from '../login/login-response.payload';
import { map, tap } from 'rxjs/operators';
import {environment} from '../../../environments/environment';
@Injectable({
providedIn: 'root'
})
export class AuthService {
@Output() loggedIn: EventEmitter<boolean> = new EventEmitter();
@Output() username: EventEmitter<string> = new EventEmitter();
refreshTokenPayload = {
refreshToken: this.getRefreshToken(),
username: this.getUserName()
}
baseUrl = environment.baseUrl;
constructor(private httpClient: HttpClient,
private localStorage: LocalStorageService) {
}
signup(signupRequestPayload: SignupRequestPayload): Observable<any> {
return this.httpClient.post(this.baseUrl + 'api/auth/signup', signupRequestPayload, { responseType: 'text' });
}
login(loginRequestPayload: LoginRequestPayload): Observable<boolean> {
return this.httpClient.post<LoginResponse>(this.baseUrl + 'api/auth/login',
loginRequestPayload).pipe(map(data => {
this.localStorage.store('authenticationToken', data.authenticationToken);
this.localStorage.store('username', data.username);
this.localStorage.store('refreshToken', data.refreshToken);
this.localStorage.store('expiresAt', data.expiresAt);
this.loggedIn.emit(true);
this.username.emit(data.username);
return true;
}));
}
refreshToken() {
return this.httpClient.post<LoginResponse>(this.baseUrl + 'api/auth/refresh/token',
this.refreshTokenPayload)
.pipe(tap(response => {
this.localStorage.clear('authenticationToken');
this.localStorage.clear('expiresAt');
this.localStorage.store('authenticationToken', response.authenticationToken);
this.localStorage.store('expiresAt', response.expiresAt);
}));
}
logout() {
this.httpClient.post(this.baseUrl + 'api/auth/logout', this.refreshTokenPayload,
{ responseType: 'text' })
.subscribe(data => {
console.log(data);
}, error => {
throwError(error);
})
this.localStorage.clear('authenticationToken');
this.localStorage.clear('username');
this.localStorage.clear('refreshToken');
this.localStorage.clear('expiresAt');
}
getJwtToken() {
console.log('triggered)')
return this.localStorage.retrieve('authenticationToken');
}
getRefreshToken() {
return this.localStorage.retrieve('refreshToken');
}
getUserName() {
return this.localStorage.retrieve('username');
}
isLoggedIn(): boolean {
return this.getJwtToken() != null;
}
}
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { HeaderComponent } from './header/header.component';
import {HTTP_INTERCEPTORS, HttpClientModule} from '@angular/common/http';
import { MapComponent } from './map/map.component';
import { HomeComponent } from './home/home.component';
import {LoginComponent} from "./auth/login/login.component";
import {ReactiveFormsModule} from "@angular/forms";
import {BrowserAnimationsModule} from "@angular/platform-browser/animations";
import { ToastrModule } from 'ngx-toastr';
import {NgxWebstorageModule} from "ngx-webstorage";
import { CreateVehicleComponent } from './create-vehicle/create-vehicle.component';
import {TokenInterceptor} from './token-interceptor';
import { SignupComponent } from './auth/sign-up/sign-up.component';
import {NgbModule} from '@ng-bootstrap/ng-bootstrap';
@NgModule({
declarations: [
AppComponent,
HeaderComponent,
SignupComponent,
MapComponent,
HomeComponent,
LoginComponent,
CreateVehicleComponent
],
imports: [
BrowserModule,
AppRoutingModule,
ReactiveFormsModule,
HttpClientModule,
NgxWebstorageModule.forRoot(),
BrowserAnimationsModule,
ToastrModule.forRoot(),
NgbModule
],
providers: [
{
provide: HTTP_INTERCEPTORS,
useClass: TokenInterceptor,
multi: true
}
],
bootstrap: [AppComponent]
})
export class AppModule { }
Angular版
Angular CLI: 11.2.0
Node: 14.15.0
OS: win32 x64
Angular:
...
Ivy Workspace:
Package Version
------------------------------------------------------
@angular-devkit/architect 0.1102.0 (cli-only)
@angular-devkit/core 11.2.0 (cli-only)
@angular-devkit/schematics 11.2.0 (cli-only)
@schematics/angular 11.2.0 (cli-only)
@schematics/update 0.1102.0 (cli-only)
我的應用基於 Sai Upadhyayula 在本教程中的工作: https://programmingtechie.com/2020/05/14/building-a-reddit-clone-with-spring-boot-and-angular/
如果您需要更多詳細信息,請告訴我
您是否在本地機器上以生產模式測試了您的構建? 可能存在環境文件問題。
嘗試在本地運行 Heroku,這將有助於輕松調試/查找根本原因
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.