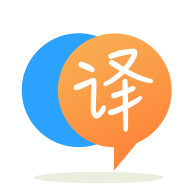
[英]How do I dynamically add a random amount of images to a div with javascript?
[英]How do I add images to cascading selects dynamically in JavaScript?
我正在做一個項目,我需要根據用戶的選擇進行動態變化的級聯選擇。 訪問這個小網站時,將提示用戶 select 兩個控制台之一,然后基於此,select 為所述控制台選擇顏色,然后選擇它附帶的游戲。 這是我當前的 JS 和 HTML:
HTML
<html>
<head>
<title>Cascading Selects</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<h2>Console Picker/Store</h2>
<div id="selectsDiv">
</div>
<script src="selectData.js"></script>
<script src="createSelects.js" ></script>
</body>
</html>
JS 創建選擇:
let selectsDiv = document.getElementById("selectsDiv");
let consoleSelect = document.createElement('select');
consoleSelect.setAttribute("id", "console");
consoleSelect.options.add(new Option('-- Select a Console --', 'console')); //new Option(text, value)
selectsDiv.appendChild(consoleSelect);
let colorSelect = document.createElement('select');
colorSelect.setAttribute("id", "color");
colorSelect.options.add(new Option('-- Select a Color --', 'color'));
selectsDiv.appendChild(colorSelect);
let gameSelect = document.createElement('select');
gameSelect.setAttribute("id", "game");
gameSelect.options.add(new Option('-- Select a Game --', 'game'));
selectsDiv.appendChild(gameSelect);
for (let console in selectData) { //for...in iterates over object properties
consoleSelect.options.add(new Option(console, console)); //new Option(text, value)
}
consoleSelect.onchange = function () {
// remove previous options from depending selects
colorSelect.length = 1;
gameSelect.length = 1;
// add options to the depending select based on the current select value
for (let color in selectData[this.value]) {
colorSelect.options.add(new Option(color, color));
}
};
colorSelect.onchange = function () {
// remove previous options from depending selects
gameSelect.length = 1;
for (let game in selectData[this.previousElementSibling.value][this.value]) {
gameSelect.options.add(new Option(game, game));
}
};
};
JS選擇中的數據:
const selectData = {//JS Object which properties are strings
"Nintendo Switch": {
"Blue-Red Version": {
"Pokemon Sword": 0,
"Pokemon Shield": 0
},
"Gray-Black Version": {
"Legend of Zelda: Breath of The Wild": 0,
"Super Smash Bros. Ultimate": 0,
}
},
"PlayStation 5": {
"Digital Version (White)": {
"Marvel's Spider-Man: Miles Morales (Digital Download)": 0
},
"Disc Version (White)": {
"Demon's Souls: Remake": 0
}
}
};
我的問題是,例如,當有人點擊“Nintendo Switch”時,如何在頁面上的 select 框下動態顯示 Switch 的圖像? 等等等等根據進一步的選擇動態地改變那個圖像?
您可以做的是創建一個consoles
object,其中包含控制台的名稱作為 object 鍵和路徑 url 的值。 然后一旦完成,您可以根據select dropdown
的值更改圖像元素的 src
如果您檢查 Playstation5 控制台,您會看到圖像沒有改變,但這只是更新consoles
object 的問題
const consoles = { "Nintendo Switch": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch", "Blue-Red Version": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch - Blue Red Version", "Pokemon Sword": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch - Blue Red Version - Pokemon Sword", }, "Pokemon Shield": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch - Blue Red Version - Pokemon Shield", } }, "Gray-Black Version": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch - Gray-Black Version", "Legend of Zelda: Breath of The Wild": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch - Gray-Black Version - Legend of Zelda: Breath of The Wild", }, "Super Smash Bros. Ultimate": { "picture": "https://dummyimage.com/200x200/63acf0/fff&text=Nintendo Switch", } } }, "PlayStation 5": { "picture": "https://dummyimage.com/100x100/63acf0/fff&text=Playstation 5", "Digital Version (White)": { "picture": "https://dummyimage.com/100x100/63acf0/fff&text=Playstation 5", "Marvel's Spider-Man: Miles Morales (Digital Download)": { "picture": "https://dummyimage.com/100x100/63acf0/fff&text=Playstation 5", } }, "Disc Version (White)": { "picture": "https://dummyimage.com/100x100/63acf0/fff&text=Playstation 5", "Demon's Souls: Remake": { "picture": "https://dummyimage.com/100x100/63acf0/fff&text=Playstation 5", } } }, } const selectData = {//JS Object which properties are strings "Nintendo Switch": { "Blue-Red Version": { "Pokemon Sword": 0, "Pokemon Shield": 0 }, "Gray-Black Version": { "Legend of Zelda: Breath of The Wild": 0, "Super Smash Bros. Ultimate": 0, } }, "PlayStation 5": { "Digital Version (White)": { "Marvel's Spider-Man: Miles Morales (Digital Download)": 0 }, "Disc Version (White)": { "Demon's Souls: Remake": 0 } } }; let selectedConsole, selectedColor, selectedGame; let consolePicture = document.getElementById("consolePicture"); let selectsDiv = document.getElementById("selectsDiv"); let consoleSelect = document.createElement('select'); consoleSelect.setAttribute("id", "console"); consoleSelect.options.add(new Option('-- Select a Console --', 'console')); //new Option(text, value) selectsDiv.appendChild(consoleSelect); let colorSelect = document.createElement('select'); colorSelect.setAttribute("id", "color"); colorSelect.options.add(new Option('-- Select a Color --', 'color')); selectsDiv.appendChild(colorSelect); let gameSelect = document.createElement('select'); gameSelect.setAttribute("id", "game"); gameSelect.options.add(new Option('-- Select a Game --', 'game')); selectsDiv.appendChild(gameSelect); for (let console in selectData) { //for...in iterates over object properties consoleSelect.options.add(new Option(console, console)); //new Option(text, value) } function setPicture(picture) { consolePicture.src = picture; } consoleSelect.onchange = function () { // remove previous options from depending selects colorSelect.length = 1; gameSelect.length = 1; // add options to the depending select based on the current select value for (let color in selectData[this.value]) { colorSelect.options.add(new Option(color, color)); } selectedConsole = consoles[this.value]; setPicture(selectedConsole.picture) }; colorSelect.onchange = function () { // remove previous options from depending selects gameSelect.length = 1; for (let game in selectData[this.previousElementSibling.value][this.value]) { gameSelect.options.add(new Option(game, game)); } selectedColor = selectedConsole[this.value]; setPicture(selectedColor.picture) } gameSelect.onchange = function() { selectedGame = selectedColor[this.value] setPicture(selectedGame.picture) }
<html> <head> <title>Cascading Selects</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <h2>Console Picker/Store</h2> <div id="selectsDiv"> </div> <img id="consolePicture" /> <script src="selectData.js"></script> <script src="createSelects.js" ></script> </body> </html>
您可以將參數傳遞給分配給onchange
事件的回調 function 的參數,該事件具有一個名為target
的屬性,該屬性是對 object 的引用,該事件被調度到該屬性上,這是一個具有值的選項
const selectData = { //JS Object which properties are strings "Nintendo Switch": { "Blue-Red Version": { "Pokemon Sword": 0, "Pokemon Shield": 0, }, "Gray-Black Version": { "Legend of Zelda: Breath of The Wild": 0, "Super Smash Bros. Ultimate": 0, }, }, "PlayStation 5": { "Digital Version (White)": { "Marvel's Spider-Man: Miles Morales (Digital Download)": 0, }, "Disc Version (White)": { "Demon's Souls: Remake": 0, }, }, }; let selectsDiv = document.getElementById("selectsDiv"); let consoleSelect = document.createElement("select"); consoleSelect.setAttribute("id", "console"); consoleSelect.options.add(new Option("-- Select a Console --", "console")); //new Option(text, value) selectsDiv.appendChild(consoleSelect); let colorSelect = document.createElement("select"); colorSelect.setAttribute("id", "color"); colorSelect.options.add(new Option("-- Select a Color --", "color")); selectsDiv.appendChild(colorSelect); let gameSelect = document.createElement("select"); gameSelect.setAttribute("id", "game"); gameSelect.options.add(new Option("-- Select a Game --", "game")); selectsDiv.appendChild(gameSelect); for (let console in selectData) { //for...in iterates over object properties consoleSelect.options.add(new Option(console, console)); //new Option(text, value) } consoleSelect.onchange = function (e) { // remove previous options from depending selects colorSelect.length = 1; gameSelect.length = 1; // add options to the depending select based on the current select value for (let color in selectData[this.value]) { colorSelect.options.add(new Option(color, color)); } if (e.target.value === 'Nintendo Switch') { console.log('Nintendo Switch selected'); } else if (e.target.value === 'PlayStation 5') { console.log('PlayStation 5 selected'); } }; colorSelect.onchange = function () { // remove previous options from depending selects gameSelect.length = 1; for (let game in selectData[this.previousElementSibling.value][this.value]) { gameSelect.options.add(new Option(game, game)); } };
<html> <head> <title>Cascading Selects</title> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> </head> <body> <h2>Console Picker/Store</h2> <div id="selectsDiv"></div> <script src="selectData.js"></script> <script src="createSelects.js"></script> </body> </html>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.