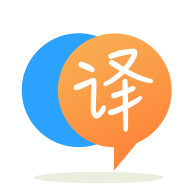
[英]How to resize ec2 instance type dynamically using AWS Lambda function with Boto3
[英]How to Stop and Start EC2 Instance using boto3 and lambda Function
我想使用 Lambda Function 啟動和停止 EC2 實例
我可以使用實例 ID 啟動和停止 EC2 實例,但是我如何才能對實例名稱執行相同操作,我正在嘗試這樣做,因為我的最終用戶不知道什么是實例 ID,他們只知道 Instance姓名
下面是我的代碼,它可以正常工作,例如 ID
import json
import boto3
region = 'us-east-1'
ec2 = boto3.client('ec2', region_name=region)
def lambda_handler(event, context):
instances = event["instances"].split(',')
action = event["action"]
if action == 'Start':
print("STARTing your instances: " + str(instances))
ec2.start_instances(InstanceIds=instances)
response = "Successfully started instances: " + str(instances)
elif action == 'Stop':
print("STOPping your instances: " + str(instances))
ec2.stop_instances(InstanceIds=instances)
response = "Successfully stopped instances: " + str(instances)
return {
'statusCode': 200,
'body': json.dumps(response)
}
我為停止而傳遞的事件
{
"instances": "i-0edb625f45fd4ae5e,i-0818263a2152a23bd,i-0cd2e17ba6f62f651",
"action": "Stop"
}
我為開始而傳遞的事件
{
"instances": "i-0edb625f45fd4ae5e,i-0818263a2152a23bd,i-0cd2e17ba6f62f651",
"action": "Start"
}
實例名稱基於名為Name
的標簽。 因此,要根據名稱獲取實例 ID,您必須按標簽過濾實例。 以下是一種可能的方法:
import json
import boto3
region = 'us-east-1'
ec2 = boto3.client('ec2', region_name=region)
def get_instance_ids(instance_names):
all_instances = ec2.describe_instances()
instance_ids = []
# find instance-id based on instance name
# many for loops but should work
for instance_name in instance_names:
for reservation in all_instances['Reservations']:
for instance in reservation['Instances']:
if 'Tags' in instance:
for tag in instance['Tags']:
if tag['Key'] == 'Name' \
and tag['Value'] == instance_name:
instance_ids.append(instance['InstanceId'])
return instance_ids
def lambda_handler(event, context):
instance_names = event["instances"].split(',')
action = event["action"]
instance_ids = get_instance_ids(instance_names)
print(instance_ids)
if action == 'Start':
print("STARTing your instances: " + str(instance_ids))
ec2.start_instances(InstanceIds=instance_ids)
response = "Successfully started instances: " + str(instance_ids)
elif action == 'Stop':
print("STOPping your instances: " + str(instance_ids))
ec2.stop_instances(InstanceIds=instance_ids)
response = "Successfully stopped instances: " + str(instance_ids)
return {
'statusCode': 200,
'body': json.dumps(response)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.