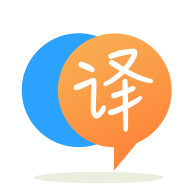
[英]how add custom validator for `javax.validation.constraints` annotation?
[英]json validator in Java - using javax.validation.constraints
我正在使用 javax.validation.constraints 並且已經檢查了 package 的用法,但仍然找不到我想做的事情。 https://javaee.github.io/javaee-spec/javadocs/javax/validation/constraints/package-summary.html
這是從請求正文發送的兩個變量
@NotNull
@PositiveOrZero
@Digits(integer = 9, fraction = 0)
private BigDecimal valueA;
@NotNull
@PositiveOrZero
@Digits(integer = 9, fraction = 0)
private BigDecimal valueB;
是否可以僅通過注釋將 valueB 限制為不超過 valueA 的 50%? (值B <= 值A/2)
您正在尋找的是使用跨參數約束。 一些基本指南可以在這里找到第 2.x 章https://www.baeldung.com/javax-validation-method-constraints
有兩種方法可以做到這一點:
@AssertTrue
public boolean isFiftyPercent(){
//your logic to compare value a and value b
}
為此,您需要有一個 class 級別的注釋。 字段級注釋僅訪問字段的值。
這是一個例子:
import static java.lang.annotation.ElementType.TYPE;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Documented;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import javax.validation.Constraint;
import javax.validation.Payload;
// Custom annotation
@Target({ TYPE })
@Retention(RUNTIME)
@Repeatable(NotGreaterThans.class)
@Documented
@Constraint(validatedBy = { NotGreaterThanValidator.class }) // Explicitly define validator
public @interface NotGreaterThan {
String source();
String target();
double percentage()
String message() default "Default message ..";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
@Target({ TYPE })
@Retention(RUNTIME)
@Documented
@interface NotGreaterThans {
NotGreaterThan[] value();
}
}
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
// Validator accesses both annotation and object value
public class NotGreaterThanValidator implements ConstraintValidator<NotGreaterThan, Object> {
private String source;
private String target;
private double percentage;
@Override
public void initialize(NotGreaterThan notGreaterThan) {
this.source = notGreaterThan.source();
this.target = notGreaterThan.target();
this.percentage = notGreaterThan.percentage();
}
@Override
public boolean isValid(Object value, ConstraintValidatorContext context) {
BigDecimal sourceValue = customMethodToGetFieldValue(source, value);
BigDecimal targetValue = customMethodToGetFieldValue(target, value);
return source.compareTo(target.multiply(percentage)) <= 0;
}
private BigDecimal customMethodToGetFieldValue(String fieldName, Object object) {
return ....
}
}
// Define your annotation on type
@NotGreaterThan(source ="a", target="b", percentage =50.0)
public class MyCustomBodyClass {
private BigDecimal a;
private BigDecimal b;
}
我尚未對此進行測試,但應該讓您先行一步。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.