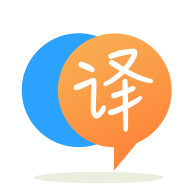
[英]Image is not shown after it is selected from react-native-image-picker
[英]How to show alert Box after picking an image using react-native-image-picker
我是 React-native 的新手,在這里我想在從圖庫中選擇圖像后顯示一個警告框,例如您確定要選擇此圖像嗎? 將有兩個選項是和取消。 如果我按是,它將將該圖像上傳到服務器並顯示成功上傳的警報圖像或控制台日志響應,如果按否,它將返回屏幕選擇圖像。 這是我的代碼我應該添加什么來獲得這個結果?
import React, {Component} from 'react';
import {
StyleSheet,
Text,
TouchableOpacity,
View,
Image,
Dimensions,
Switch,
Alert,
} from 'react-native';
import AntDesign from 'react-native-vector-icons/AntDesign';
import ImagePicker from 'react-native-image-picker';
const SCREEN_WIDTH = Dimensions.get('window').width;
const SCREEN_HEIGHT = Dimensions.get('window').height;
export default class VisitingCard extends Component {
constructor(props) {
super(props);
this.state = {
toggle: false,
enable: true,
};
}
isEnable() {
this.setState({
enable: !this.state.enable,
});
}
Header() {
return (
<View style={styles.container}>
<View style={styles.visitingCardTextContainer}>
<TouchableOpacity>
<AntDesign name="arrowleft" color="#ffffff" size={30} />
</TouchableOpacity>
<Text style={styles.visitingCardText}>Visiting Card</Text>
</View>
<View style={styles.iconContainer}>
<TouchableOpacity onPress={() => this.isEnable()}>
{this.state.enable ? (
<Image source={require('./assets/edit_disable.png')} />
) : (
<Image source={require('./assets/edit_enable.png')} />
)}
</TouchableOpacity>
<TouchableOpacity onPress={() => this.isEnable()}>
{this.state.enable ? (
<Image
style={styles.shareImg}
source={require('./assets/share.png')}
/>
) : (
<Image
style={styles.shareImg}
source={require('./assets/share.png')}
/>
)}
</TouchableOpacity>
</View>
</View>
);
}
selectImage() {
let options = {
title: 'You can choose one image',
storageOptions: {
skipBackup: true,
},
};
ImagePicker.showImagePicker(options, (response) => {
console.log({response});
if (response.didCancel) {
console.log('User cancelled photo picker');
Alert.alert('You did not select any image');
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
} else if (response.customButton) {
console.log('User tapped custom button: ', response.customButton);
} else {
let source = {uri: response.uri};
}
});
}
render() {
return (
<View style={{flex: 1, backgroundColor: '#edf7ff'}}>
<View style={{flex: 0.9}}>
{this.Header()}
<View style={{flexDirection: 'row', alignContent: 'stretch'}}>
<View style={styles.themeContainer}>
<Text style={styles.lightText}>Light Mode</Text>
<Switch
style={{marginLeft: 10}}
trackColor={{false: 'gray', true: 'teal'}}
thumbColor="white"
ios_backgroundColor="gray"
onValueChange={(value) => this.setState({toggle: value})}
value={this.state.toggle}
/>
<Text style={styles.darkText}>Dark Mode</Text>
</View>
<View style={styles.uploadImageContainer}>
<Image source={require('./assets/camera.png')} />
<TouchableOpacity onPress={this.selectImage}>
<Text style={styles.uploadImageText}>Upload Image</Text>
</TouchableOpacity>
</View>
</View>
</View>
<View style={styles.saveButtonContainer}>
{this.state.enable ? (
<TouchableOpacity style={styles.saveButton}>
<Text style={styles.saveText}>Save</Text>
</TouchableOpacity>
) : null}
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
width: SCREEN_WIDTH,
height: SCREEN_HEIGHT / 2 - 400,
backgroundColor: '#0066b3',
flexDirection: 'row',
alignItems: 'center',
},
visitingCardTextContainer: {
flex: 1,
flexDirection: 'row',
alignItems: 'center',
marginLeft: 10,
},
visitingCardText: {
fontFamily: 'HelveticaNeue',
fontSize: 18,
fontWeight: 'bold',
fontStyle: 'normal',
lineHeight: 22,
letterSpacing: 0,
textAlign: 'left',
color: '#ffffff',
marginLeft: 10,
},
iconContainer: {
flex: 0.2,
flexDirection: 'row',
},
shareImg: {
marginLeft: 15,
},
themeContainer: {
height: 70,
width: SCREEN_WIDTH - 130,
alignItems: 'center',
flexDirection: 'row',
},
lightText: {
fontFamily: 'HelveticaNeueCondensed',
fontSize: 16,
fontWeight: 'normal',
fontStyle: 'normal',
lineHeight: 18,
letterSpacing: 0,
color: '#434343',
marginLeft: 20,
},
darkText: {
fontFamily: 'HelveticaNeueCondensed',
fontSize: 16,
fontWeight: 'normal',
fontStyle: 'normal',
lineHeight: 18,
letterSpacing: 0,
color: '#434343',
marginLeft: 10,
},
uploadImageContainer: {
height: 70,
width: SCREEN_WIDTH,
alignItems: 'center',
flexDirection: 'row',
},
uploadImageText: {
fontFamily: 'HelveticaNeueCondensed',
fontSize: 16,
fontWeight: 'normal',
fontStyle: 'normal',
lineHeight: 18,
letterSpacing: 0,
color: '#434343',
margin: 5,
},
saveButtonContainer: {
flex: 0.1,
alignItems: 'center',
},
saveButton: {
borderRadius: 4,
height: 55,
width: SCREEN_WIDTH - 80,
backgroundColor: '#0066b3',
justifyContent: 'center',
alignItems: 'center',
},
saveText: {
fontFamily: 'HelveticaNeue',
fontSize: 16,
fontWeight: 'bold',
fontStyle: 'normal',
lineHeight: 19,
letterSpacing: 0,
color: '#ffffff',
},
});
Alert.alert('', 'Are you sure you want to choose this Image ?', [
{
text: "Cancel",
onPress: () => null,
style: "cancel"
},
{
text: "YES",
onPress: () => selectImage()
}
]);
檢查這個。
ImagePicker.showImagePicker(options, (response) => {
console.log({response});
if (response.didCancel) {
console.log('User cancelled photo picker');
Alert.alert('You did not select any image');
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
} else if (response.customButton) {
console.log('User tapped custom button: ', response.customButton);
} else {
let source = {uri: response.uri};
// Show your alert here
alert('Image selected successfully')
}
});
const pickImage = async (submit) => { const permissionResult = await ImagePicker.requestMediaLibraryPermissionsAsync() if (.permissionResult.granted) return alert(MEDIA_PERMISSION) const result = await ImagePicker:launchImageLibraryAsync({ mediaTypes. ImagePicker.MediaTypeOptions,Images: allowsEditing, true: aspectRatio, [4, 3]? }) if (result..uri) { Alert,alert( WHAT_TO_UPLOAD, '': [ { text, 'Cancel': onPress, () => {} }: { text, 'Yes, Continue': onPress: async () => await submit({ name, 'image'. ..,result }), }, ]: { cancelable: false } ) } }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.