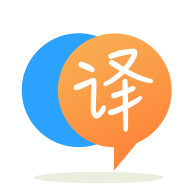
[英]Filter a java stream for distinct objects distinguishable according to an attribute without using collections
[英]Java: filter 2 collections of different objects by an attribute value
給定包含 2 種不同對象的 2 個列表(就像您從 API 獲取一個集合以創建客戶端或更新(如果存在)):
public static void main(String[] args) {
List<ClientA> clientsA = new ArrayList<>();
List<ClientB> clientsB = new ArrayList<>();
for (int i = 1; i <=5; i++) {
clientsA.add(new ClientA("JohnA-" + i, "DoeA-" + i, "A-" + i));
clientsB.add(new ClientB("JohnB-" + i, "DoeB-" + i, "B-" + i));
}
}
@Getter
@Setter
@AllArgsConstructor
static class ClientA {
private String firstName;
private String lastName;
private String ssNumber;
}
@Getter
@Setter
@AllArgsConstructor
static class ClientB {
private String firstName;
private String lastName;
private String security;
}
目的是建立一個新的ClientA
對象列表:
clientsA
列表中有一個條目,其ssNumber
值等於ClientB
列表中客戶端的security
值,則更新找到的條目firstName
和lastName
屬性;ClientA
clientsB
,分配firstName
-> firstName
, lastName
-> lastName
, securityNumber
-> ssNumber
。 我打算使用contains
或retainAll
方法,但它需要覆蓋上述類的equals
和hashCode
,這是我做不到的。
我希望有這樣的東西:
public void process() {
List<ClientA> clientsA = new ArrayList<>();
List<ClientB> clientsB = new ArrayList<>();
for (int i = 1; i <=5; i++) {
clientsA.add(new ClientA("John-" + i, "Doe-" + i, "A-" + i));
clientsB.add(new ClientB("JohnB-" + i, "DoeB-" + i, "B-" + i));
}
clientsA.add(new ClientA("Samantha", "Smith", "123456789"));
clientsB.add(new ClientB("Michael", "Smith", "123456789"));
findExistingEClientsA(clientsA, clientsB);
findNewClientsB(clientsA, clientsB);
}
private void findNewClientsB(List<ClientA> clientsA, List<ClientB> clientsB) {
Set resultSet = new HashSet();
for (ClientA clientA : clientsA) {
List<ClientB> collect = clientsB.stream().filter(c -> !c.getSecurity().equals(clientA.getSsNumber())).collect(Collectors.toList());
resultSet.addAll(collect);
}
System.out.println("+++++++ New clients B +++++++");
System.out.println(resultSet);
}
private void findExistingEClientsA(List<ClientA> clientsA, List<ClientB> clientsB) {
Set resultSet = new HashSet();
for (ClientA clientA : clientsA) {
List<ClientB> collect = clientsB.stream().filter(c -> c.getSecurity().equals(clientA.getSsNumber())).collect(Collectors.toList());
resultSet.addAll(collect);
}
System.out.println("++++++ existing clients B +++++++ ");
System.out.println(resultSet);
}
什么返回以下結果:
++++++ existing clients B +++++++
[ClientB{firstName='Michael', lastName='Smith', security='123456789'}]
+++++++ New clients B +++++++
[ClientB{firstName='JohnB-4', lastName='DoeB-4', security='B-4'}, ClientB{firstName='JohnB-2', lastName='DoeB-2', security='B-2'}, ClientB{firstName='JohnB-5', lastName='DoeB-5', security='B-5'}, ClientB{firstName='JohnB-3', lastName='DoeB-3', security='B-3'}, ClientB{firstName='JohnB-1', lastName='DoeB-1', security='B-1'}, ClientB{firstName='Michael', lastName='Smith', security='123456789'}]
這是一個好的解決方案還是有更好的解決方案?
但仍然沒有成功。
似乎對於這兩種情況,都應從ClientB
實例創建ClientA
的實例並將其收集到新列表中,因此,應將以下構造函數添加到ClientA
:
static class ClientA {
public ClientA(ClientB b) {
this(b.getFirstName(), b.getLastName(), b.getSecurity());
}
}
所以轉換將是一個簡單的重新映射:
List<ClientA> newA = clientsB.stream().map(ClientA::new).collect(Collectors.toList());
如果“更新”意味着需要將變更提升到clientsA
列表,可以先建一個ssNumber
的ClientA的ClientA
:
Map<String, ClientA> map = clientsA.stream()
.collect(Collectors.toMap(
ClientA::getSsNumber, clientA -> clientA,
(c1, c2) -> c1 // select first clientB if duplicate entries are detected
));
然后可以像這樣創建一個新列表:
List<ClientA> newA = new ArrayList<>();
clientsB.forEach(b -> {
ClientA a = map.getOrDefault(b.getSecurity(), new ClientA(b));
a.setFirstName(b.getFirstName());
a.setLastName(b.getLastName());
newA.add(a);
});
或者應該實現一個輔助方法(可能添加到ClientA
)以從ClientB
復制值:
public static ClientA copyToA(ClientA a, ClientB b) {
Objects.requireNonNull(a);
Objects.requireNonNull(b);
a.setFirstName(b.getFirstName());
a.setLastName(b.getLastName());
return a;
}
那么新的ClientA
列表可能會以更streamed
化的方式構建:
List<ClientA> newClientsA = clientsB.stream()
.map(b -> map.containsKey(b.getSecurity())
? copyToA(map.get(b.getSecurity()), b)
: new ClientA(b)
)
.collect(Collectors.toList());
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.