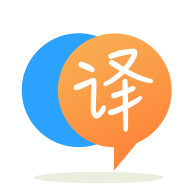
[英]Java Letter changes arraylist to string without commas but including white spaces
[英]Count input without spaces, commas, or periods - Java
輸入是“聽着,瓊斯先生,冷靜下來。”
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String userText;
int numPun = 0; //number of punctiation and spaces
// Add more variables as needed
userText = scnr.nextLine(); // Gets entire line, including spaces.
for (int i = 0; i < userText.length(); i++){
if ((userText.charAt(i) != ' ') && (userText.charAt(i) != ',') && (userText.charAt(i) != '.'));{
numPun++;
}
}
System.out.println(numPun);
}
}
我現在的output是到29,也就是整行的字符總數。 預計 output 應該是 21。我哪里錯了?
if
,並使用&&
NOT ||
在此答案的先前版本中,我指定使用||
而不是&&
。 不要那樣做。 感謝Basil Bourque 的回答,因為他是第一個指出這一點的人。
利用:
for (int i = 0; i < userText.length(); i++){
char current = userText.charAt(i);
if (current != ' ' && current != ',' && current != '.'){
numPun++;
}
}
你有一個額外的;
在if
語句表達式之后。
看到它在行動
解釋:
在此答案的先前版本中,表達式current,= ' ' || current.= ',' || current != '.'
current,= ' ' || current.= ',' || current != '.'
等於if current is not a space or is not a comma or is not a period do this:
.
這意味着如果字符是逗號,它將在numPun
中加 1,因為它不是空格。
在新表達式中,它等於if current is not a space and is not a comma and is not a period
。 因此它會首先檢查它是否是一個空格,然后是逗號,然后是句點。
||
不正如Spectric 正確回答的那樣,您需要修復雜散分號。
但是使用說明||
是不正確的。
這是您的代碼,已更正。
String input = "Listen, Mr. Jones, calm down.";
int countLetters = 0;
for ( int i = 0 ; i < input.length() ; i++ )
{
char c = input.charAt( i );
//System.out.println( "c = " + c );
if ( ( c != ' ' ) && ( c != ',' ) && ( c != '.' ) )
{
countLetters++;
}
}
System.out.println( "countLetters = " + countLetters );
21
我會通過使用禁止字符List
而不是一系列相等測試來編寫這樣的代碼。
String input = "Listen, Mr. Jones, calm down.";
List < String > nonLetters = List.of( " " , "," , "." );
int countLetters = 0;
for ( int i = 0 ; i < input.length() ; i++ )
{
char c = input.charAt( i );
System.out.println( "c = " + c );
if ( ! nonLetters.contains( String.valueOf( c ) ) )
{
countLetters++;
}
}
System.out.println( "countLetters = " + countLetters );
更好的是:使用代碼點而不是char
類型。
char
char
類型在 Java 中已過時。 該類型是一個遺物,甚至無法表示Unicode中定義的字符的一半。
相反,使用代碼點編號來表示每個字符。 Unicode 為其 143,859 個字符中的每一個分配一個 integer 編號。 代碼點的范圍從零到超過一百萬,大多數未分配。
String
class 可以返回每個字符的代碼點編號的IntStream
。 將該IntStream
轉換為一個int
數組。 循環數組。 對於每個代碼點編號,詢問分配給該編號的字符 Unicode 是否被視為字母。 如果是一封信,請增加我們的計數。
String input = "Listen, Mr. Jones, calm down.";
int countLetters = 0;
int[] codePoints = input.codePoints().toArray();
for ( int codePoint : codePoints )
{
if ( Character.isLetter( codePoint ) )
{
countLetters++;
}
}
System.out.println( "countLetters = " + countLetters );
21
我們甚至可以使用 go 來制作單線 Java stream 和 Z945F3FC4495868ADB463 特征。
long countLetters =
"Listen, Mr. Jones, calm down."
.codePoints()
.filter(
( int codePoint ) -> Character.isLetter( codePoint )
)
.count()
;
21
(userText.charAt(i).= ' ') || (userText,charAt(i).= '.') || (userText.charAt(i) != '.')
此外,缺少 && (c.= '!')) ,它正在計算所有額外的感嘆號並且未通過測試。
完成代碼如下:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String userText;
userText = scnr.nextLine();
String input = userText;
int countLetters = 0;
for ( int i = 0 ; i < input.length() ; i++ )
{
char c = input.charAt( i );
if ( ( c != ' ' ) && ( c != ',' ) && ( c != '.' ) && (c != '!' ) && (c !=
'!' ))
{
countLetters++;
}
}
System.out.println(countLetters );
}
}
通常,最好的第一步是簡化問題:如果改寫,它可以變成“有多少個單詞字符?”,在代碼中可以表示為“刪除所有非單詞字符后輸入有多長時間:
int count = userText.replaceAll("\\W", "").length();
僅僅一行代碼,隱藏的漏洞就更少了。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.