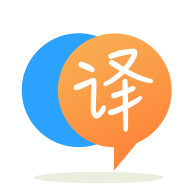
[英]How list of object return a Spring Page with Mongo repository?
[英]How to return list of specific fields in query Java Spring Mongo Repository?
我在我的項目中使用 Java spring 和 MongoDB 存儲庫。
這是DTO定義:
@Document(collection = "Info")
public class Info {
String id,
Integer count,
String type
…
}
我需要從查詢中返回一個 ID 列表,其中計數字段不為零並且類型字段具有“二進制”文本。
這里我如何嘗試實現它:
@Query(value="{ 'count' : 0, 'type' : 'binary' }", fields="{ 'id' : 1 }")
List<String> getInfo();
我從上面的查詢中得到這個結果:
0={"_id": {"$oid": "5eb97a8139d4c62be4d90e4c"}}
1={"_id": {"$oid": "3ec97a8127d4c60cb4d90e9e"}}
我期待這個結果:
{"5eb97a8139d4c62be4d90e4c", "3ec97a8127d4c60cb4d90e9e"}
如您所見,我希望從上面的查詢中獲得一個 id 字符串列表。
知道我應該在上面的查詢中更改什么以獲得預期的 ids 結果列表嗎?
不,這不可能是你的想法。
原因: MongoDB只能返回JSON 文件。 您可以包含您希望出現的字段。
您可以遵循以下建議:
DTO 定義:
@Document(collection = "Info")
public class Info {
@Id
private String id;
private Integer count;
private String type;
// other fields and getters and setters
}
樣本庫:
public interface InfoRepository extends MongoRepository<Info, String> {
@Query(value="{ 'count' : 0, 'type' : 'binary' }", fields="{ 'id' : 1 }")
List<Info> getInfo();
}
樣品服務 class:
@Service
public class InfoService {
@Autowired
private InfoRepository infoRepository;
public List<String> getIds() {
return infoRepository.getInfo()
.stream()
.map(Info::getId)
.collect(Collectors.toList());
}
}
返回的{"$oid": "5eb97a8139d4c62be4d90e4c"}
是 ObjectID 的MongoDB Extended JSON表示。
它沒有返回字符串,因為存儲在數據庫中的字段是ObjectID
類型,而不是String
類型。
如果你希望它返回一個字符串,你應該使用聚合和$toString運算符來轉換它。
你能得到的最好的結果是一個帶有數組字段的文檔,其中找到的 id 如下所示:
{
"ids" : [
"606018909fb6351e4c34f964",
"606018909fb6351e4c34f965"
]
}
這可以通過如下聚合查詢來實現:
db.Info.aggregate([
{
$match: {
count: 0,
type: "binary"
}
},
{
$project: { _id: { $toString: "$_id" } }
},
{
$group: {
_id: null,
ids: { $push: "$_id" }
}
},
{
$project: { _id: 0 }
}
])
我有 2 條建議。
1.可以使用JPA查詢代替命名查詢
public interface InfoRepository extends MongoRepository<Info, String> {
List<Info> findByCountAndType(final Integer count, final String type);
}
2. 在您的業務邏輯中使用 java stream api 從上述結果中收集所有 id 作為 List。
public class InfoServiceImpl {
@Autowired
private InfoRepository repository;
public String getIds(final String type, final Integer count) {
return repository.findByCountAndType(count, type)
.stream()
.map(Info::getId)
.collect(Collectors.toList());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.