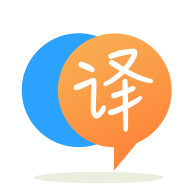
[英]Find a particular word in array of sentences and return the sentences that contain the word
[英]Find a particular word in a string array of sentences and return the frequency of a particular word throughout the array
輸入是字符串數組,如下所示,
{"1112323 400 錯誤","1112323 400 錯誤","9988778 400 錯誤"}
我需要打印時間戳,即句子開頭的數字及其在整個數組中的頻率
我到現在才到這一步。 只有在已知字符串的情況下才能找到該字符串。
int count = 0;
for(int i=str1.length-1;i>=0;i--)
{
String[] ElementOfArray = str1[i].split(" ");
for(int j=0;j<ElementOfArray.length-1;j++)
{
if(ElementOfArray[j].equals("Hi"))
{
count++;
}
}
}
System.out.println(count);
一種方法是跟蹤條目的數量並增加。
public static void main(String[] args)
{
String[] inp = {"1112323 400 error",
"1112323 400 error",
"9988778 400 error"};
Map<String,Integer> results = new HashMap<>();
for (String one : inp) {
String[] parts = one.split(" ");
String ts = parts[0];
int val = results.computeIfAbsent(ts, v-> 0);
results.put(ts, ++val);
}
System.out.println(results);
}
注意:還有其他方法可以處理 map 遞增。 這只是一個例子。
樣品 Output:
{1112323=2, 9988778=1}
現在,如果將來可能想要執行其他操作,那么使用對象可能會很有趣。
所以 class 可能是:
private static class Entry
{
private final String ts;
private final String code;
private final String desc;
public Entry(String ts, String code, String desc)
{
// NOTE: error handling is needed
this.ts = ts;
this.code = code;
this.desc = desc;
}
public String getTs()
{
return ts;
}
public static Entry fromLine(String line)
{
Objects.requireNonNull(line, "Null line input");
// NOTE: other checks would be good
String[] parts = line.split(" ");
// NOTE: should verify the basic parts
return new Entry(parts[0], parts[1], parts[2]);
}
// other getter methods
}
然后可以執行以下操作:
List<Entry> entries = new ArrayList<>();
for (String one : inp) {
entries.add(Entry.fromLine(one));
}
Map<String,Integer> res2 = entries.stream()
.collect(Collectors.groupingBy(x->x.getTs(),
Collectors.summingInt(x -> 1)));
System.out.println(res2);
(目前相同的樣品 output)。 但是,如果需要擴展以計算 400 個代碼的數量或其他什么,更改 stream 是微不足道的,因為 object 有數據。 當然,這種方法還有更多的擴展。
您可以使用HashMap
來解決計算時間戳的頻率。
import java.util.HashMap;
public class test {
public static void main(String[] args) {
// Create a HashMap object called timeFrequency
HashMap<String, Integer> timeFrequency = new HashMap<String, Integer>();
String []str1 = {"1112323 400 error","1112323 400 error","9988778 400 error"};
for(int i=0;i<str1.length;i++)
{
String[] ElementOfArray = str1[i].split(" ");
if(timeFrequency.containsKey(ElementOfArray[0])){
timeFrequency.put(ElementOfArray[0], timeFrequency.get(ElementOfArray[0]) + 1);
}else{
timeFrequency.put(ElementOfArray[0], 1);
}
}
System.out.println(timeFrequency);
}
}
Output:
{1112323=2, 9988778=1}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.