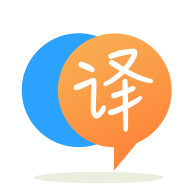
[英]I am getting read property map of undefined.I have tried everything but nothing worked
[英]React keeps saying .map is not a function and nothing I have tried is working?
我有一個組件,它使用 axios 從 API 獲取列表。 當主頁第一次加載時,所有列表都被檢索並加載正常,但是當我 go 說列表詳細信息頁面並返回主頁時,當我刷新頁面時,我得到一個“listings.map 不是函數”錯誤我沒有收到錯誤並且列表加載。
這是組件(嘗試使用加載 boolean 但什么也沒做):
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { getListings } from '../../actions/listings';
import { Link as RouteLink } from 'react-router-dom';
import PropTypes from 'prop-types';
//material ui
import {
Container,
Typography,
Button,
Card,
CardActions,
CardActionArea,
CardContent,
CardMedia,
Grid,
Link,
} from '@material-ui/core';
import { withStyles } from '@material-ui/core/styles';
const useStyles = {
listings: {
marginTop: '100px',
backgroundColor: 'white',
},
title: {
marginBottom: '15px',
},
listImg: {
width: '250px',
height: '250px',
},
card: {
width: '275px',
height: '300px',
},
media: {
height: 140,
},
};
class Listings extends Component {
static propTypes = {
listings: PropTypes.array.isRequired,
isLoading: PropTypes.bool,
};
componentDidMount() {
this.props.getListings();
}
render() {
const { classes, listings } = this.props;
return (
<>
<Container maxWidth='lg' className={classes.listings}>
<Typography align='center' variant='h1' className={classes.title}>
Recent Listings
</Typography>
<Grid container justify='center' spacing={8}>
{listings &&
listings.map((listing, index) => (
<Grid key={index} item>
<Link component={RouteLink} to={`/listing/${listing._id}`}>
<Card className={classes.card}>
<CardActionArea>
<CardMedia
className={classes.media}
title='Contemplative Reptile'
image={listing.imgUrls[0]}
/>
<CardContent>
<Typography gutterBottom variant='h6' component='h2'>
{listing.title}
</Typography>
<Typography
variant='body2'
color='textSecondary'
component='p'
>
{listing.price} /night
</Typography>
</CardContent>
</CardActionArea>
<CardActions>
<Button size='small' color='primary'>
BOOK
</Button>
</CardActions>
</Card>
</Link>
</Grid>
))}
</Grid>
</Container>
</>
);
}
}
//sets component props to the app state
const mapStateToProps = (state) => ({
listings: state.listings.listings,
isLoading: state.listings.isLoading,
});
//withStyles is used for material-ui styles (using class components)
export default connect(mapStateToProps, { getListings })(
withStyles(useStyles)(Listings)
);
這是 getListings 操作:
export const getListings = () => (dispatch) => {
axiosInstance
.get('/listings')
.then((res) => {
dispatch({
type: GET_LISTINGS,
payload: res.data,
});
})
.catch((err) => console.log(err));
};
和減速機:
const initialState = {
listings: [],
isLoading: true,
};
export default function (state = initialState, action) {
switch (action.type) {
case GET_LISTINGS:
return {
...state,
listings: action.payload,
isLoading: false,
};
case GET_LISTING:
return {
...state,
listings: action.payload,
isLoading: false,
};
case CREATE_LISTING:
return {
...state,
listings: [...state.listings, action.payload],
};
case CLEAR_LISTINGS:
return {
...state,
listings: [],
};
default:
return state;
}
}
只是不知道它是如何工作的,但有時不會。 列表在 initialState 中也被聲明為一個數組。
如果您的列表變量類型是
Object
那么你必須像這樣 map :
const object1 = {
a: 'somestring',
b: 42
};
for (const [key, value] of Object.entries(object1)) {
console.log(`${key}: ${value}`);
}
// expected output:
// "a: somestring"
// "b: 42"
// order is not guaranteed
另一方面,如果您的變量是
大批
然后:
const numbers = [2, 4, 6, 8];
const squares = numbers.map(number => number * numbers);
console.log(squares);
// output: Array [4, 16, 36, 64]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.