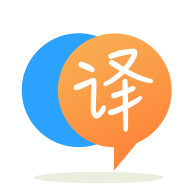
[英]AttributeError: 'str' object has no attribute 'tk', Python Tkinter
[英]Tkinter and python 'str' object has no attribute 'tk'
我想用 Python 和 Tkinter 創建一個小數字猜謎游戲,我遇到了錯誤,游戲只接受第一個按鈕按下,但如果你再次按下它會顯示錯誤。 我將告訴你代碼和回溯錯誤。 我試圖評論一切。
#import modules that are needed
from tkinter import *
import random
#define the random number used
randInt = random.randint(1,10)
#create basic tkinter window
r = Tk()
#define the window title
r.title(" Guess My Number")
#define window size
r.geometry("600x200")
#define the checkGuess function, which checks if the guess provided is the random number
def checkGuess():
#import variables
global randInt, guessEntry
#try to make the guess a integer
try:
guessEntry = Entry.get(guessEntry)
guessEntry = int(guessEntry)
#if that is not possible, prints that to the console and ends the function
except ValueError:
print("Input provided is not a whole number, please try another one")
return
#checks if the guess is the number
if randInt == guessEntry:
guessCorrectLabel = Label(r, text="Guess correct, new number created")
guessCorrectLabel.pack()
randInt = random.randint(1,10)
#checks if the guess is smaller than the number
elif randInt < guessEntry:
tooHighLabel = Label(r, text="Guess is too high")
tooHighLabel.pack()
print(randInt)
elif randInt > guessEntry:
tooLowLabel = Label(r, text="Guess is too low")
tooLowLabel.pack()
print(randInt)
#function to quit the app
def quitApp():
r.quit()
#make a title label
titleLabel = Label(r, text="Guess a random Number between 1 and 10")
#show the label on the screen
titleLabel.pack()
#make a Entry widget to enter the guess
guessEntry = Entry(r)
#show the entry widget on the screen
guessEntry.pack()
#make a button to check the entry given by the user
checkButton = Button(r, text="Check Guess", command=checkGuess)
#show the button on the screen
checkButton.pack()
#make a button to exit the application
exitButton = Button(r, text="QUIT", command=quitApp)
#show the button on the screen
exitButton.pack()
#show the window on the screen
if __name__ == "__main__":
r.mainloop()
這是第二次按鈕按下后的回溯錯誤:
Exception in Tkinter callback
Traceback (most recent call last):
File "D:\Python\Python392\lib\tkinter\__init__.py", line 1892, in __call__
return self.func(*args)
File "Z:\Coding\Projects\Pycharm\numGuesser1\main.py", line 23, in checkGuess
guessEntry = Entry.get(guessEntry)
File "D:\Python\Python392\lib\tkinter\__init__.py", line 3043, in get
return self.tk.call(self._w, 'get')
AttributeError: 'str' object has no attribute 'tk'
原因是當您按下按鈕時,您將變量guessEntry
從Entry 更改為int,這樣您將在第二次按下后得到錯誤。
試試下面的代碼:
#import modules that are needed
from tkinter import *
import random
#define the random number used
randInt = random.randint(1,10)
#create basic tkinter window
r = Tk()
#define the window title
r.title(" Guess My Number")
#define window size
r.geometry("600x200")
#define the checkGuess function, which checks if the guess provided is the random number
def checkGuess():
#import variables
global randInt, guessEntry
#try to make the guess a integer
try:
guessEntry1 = Entry.get(guessEntry)
guessEntry1 = int(guessEntry1)
#if that is not possible, prints that to the console and ends the function
except ValueError:
print("Input provided is not a whole number, please try another one")
return
#checks if the guess is the number
if randInt == guessEntry1:
guessCorrectLabel = Label(r, text="Guess correct, new number created")
guessCorrectLabel.pack()
randInt = random.randint(1,10)
#checks if the guess is smaller than the number
elif randInt < guessEntry1:
tooHighLabel = Label(r, text="Guess is too high")
tooHighLabel.pack()
print(randInt)
elif randInt > guessEntry1:
tooLowLabel = Label(r, text="Guess is too low")
tooLowLabel.pack()
print(randInt)
#function to quit the app
def quitApp():
r.quit()
#make a title label
titleLabel = Label(r, text="Guess a random Number between 1 and 10")
#show the label on the screen
titleLabel.pack()
#make a Entry widget to enter the guess
guessEntry = Entry(r)
#show the entry widget on the screen
guessEntry.pack()
#make a button to check the entry given by the user
checkButton = Button(r, text="Check Guess", command=checkGuess)
#show the button on the screen
checkButton.pack()
#make a button to exit the application
exitButton = Button(r, text="QUIT", command=quitApp)
#show the button on the screen
exitButton.pack()
#show the window on the screen
if __name__ == "__main__":
r.mainloop()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.