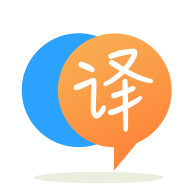
[英]How do I pass data from one component to another (New Browser Tab) in angular?
[英]Is there any method to pass live data from one Browser tab another Browser tab by using Javascript
我創建了一個遞增和遞減程序,但在其中,我想將數據從第一個瀏覽器傳遞到第二個瀏覽器(一個選項卡到另一個選項卡)。
在 Index.html 中有兩個按鈕用於遞增和遞減操作。 在第二頁上只有數據會顯示,但我的代碼只在同一個選項卡上工作。 以及如何在頁面刷新后存儲數據?
索引.html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="/index.js"></script>
<link href="/index.css" rel="stylesheet" type="text/css">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Live Users in Mall</title>
</head>
<body>
<div class="container">
<h1>Live Users in Mall</h1>
<div class = "card">
<button onclick = "decrement()">-</button>
<h2 id = "root">0</h2>
<button onclick = "increment()">+</button>
</div>
</div>
</body>
</html>
索引.css
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
background-color: rgb(255, 255, 255);
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
text-align: center;
}
.card{
display: flex;
justify-content: center;
align-items: center;
height: 100%;
padding: 100px;
}
h2{
margin: 0 50px;
font-size: 50px;
}
button{
background-color: #ff9100;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 25px;
}
button:hover{
background-color: rgb(206, 206, 206);
color: #ff9100;
border-color: #000 ;
}
index.js
var data = 0;
document.getElementById("root").innerText = data;
function decrement() {
data = data-1;
if (data <= 0)
{
data = 0;
}
document.getElementById("root").innerText = data;
localStorage.setItem("key", data);
}
function increment() {
data = data + 1;
document.getElementById("root").innerText = data;
localStorage.setItem("key", data);
}
第二頁名稱為 Showdata
Showdata.html
<!DOCTYPE html>
<html lang="en">
<head>
<link href="/index.css" rel="stylesheet" type="text/css">
<script src="/showdata.js"></script>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Show Live Data</title>
</head>
<body>
<h3>Users Present in Mall</h3>
<h2 id="root">0
</h2>
</body>
</html>
顯示數據.js
var data = localStorage.getItem("key");
document.write(data);
//output.innerHTML = data;
//document.getElementById("root").innerText=data;
誰能為我的問題提供解決方案?
您可以使用的一種(幾種)方法是使用BroadcastChannel
api。 來自MDN的宣傳語指出:
BroadcastChannel 接口表示給定來源的任何瀏覽上下文都可以訂閱的命名頻道。 它允許同一來源的不同文檔(在不同的 windows、標簽、框架或 iframe 中)之間進行通信。 消息通過在所有偵聽頻道的 BroadcastChannel 對象上觸發的消息事件進行廣播。
具有兩個按鈕的index
頁:
<!DOCTYPE html>
<html lang="en">
<head>
<style>
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
background-color: rgb(255, 255, 255);
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
text-align: center;
}
.card{
display: flex;
justify-content: center;
align-items: center;
height: 100%;
padding: 100px;
}
h2{
margin: 0 50px;
font-size: 50px;
}
button{
background-color: #ff9100;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 25px;
}
button:hover{
background-color: rgb(206, 206, 206);
color: #ff9100;
border-color: #000 ;
}
</style>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Live Users in Mall</title>
</head>
<body>
<div class="container">
<h1>Live Users in Mall</h1>
<div class="card">
<!--
Rather than using `inline` function calls it is
preferable to assign an external event listener.
You can easily use the same event handler to
perform both increment and decrement tasks.
To aid that the buttons were assign a dataset
attribute which is analysed in the click handler.
-->
<button data-action='decrement'>-</button>
<h2>0</h2>
<button data-action='increment'>+</button>
</div>
</div>
<script>
// Establish the communication channel - this can be (nearly) any name
// but the same name is needed for the listeners.
let oChan=new BroadcastChannel( 'tabs' );
let h2=document.querySelector('div.card h2');
// simple function to send a payload of your design
const sendmessage=function( data ){
let payload={ 'data':data };
oChan.postMessage( payload );
};
// fetch the data from localStorage or set as zero
let data=localStorage.getItem('key');
if( data == null )data=0;
else data=Number( data );
h2.innerHTML=data;
// assign an event listener for both buttons
document.querySelectorAll('button[data-action]').forEach( bttn=>{
bttn.addEventListener('click',function(e){
switch( this.dataset.action ){
case 'increment':data++;break;
case 'decrement':data--;break;
}
//update storage
localStorage.setItem('key',data);
// send the message to the "other" page
sendmessage( data );
// update local display
h2.innerHTML=data;
});
})
</script>
</body>
</html>
以及點擊按鈕時實時更新的listening
頁面。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Show Live Data</title>
</head>
<body>
<h3>Users Present in Mall</h3>
<h2>0</h2>
<script>
// establish listener on same channel
let oChan=new BroadcastChannel( 'tabs' );
oChan.addEventListener('message',(e)=>{
// process the messages as they arrive
document.querySelector('h2').innerHTML=e.data.data
});
</script>
</body>
</html>
index
頁面建立了一個監聽器也必須訂閱的頻道,並發送一個簡單的消息(數據類型非常靈活),該消息在第二個窗口/選項卡中處理
您可以使用 BrowserObject,並找到 window 或帶有名稱的選項卡,您可以附加到選項卡。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.