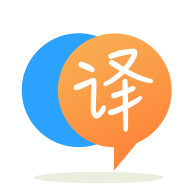
[英]In C using pthreads, how do I get a certain thread to run until a certain value has been reached?
[英]How do I notify a thread that new data is available using pthreads?
我有新數據出現在公共汽車上。 我希望我的主線程在新數據到達時“醒來”。 我的原始代碼版本是這樣的:
#include <time.h>
#include <stdio.h>
#include <pthread.h>
#include <time.h>
int data = 0;
void* thread_func(void* args)
{
while(1)
{
sleep(2);
data = random() % 5;
}
return NULL;
}
int main()
{
int tid;
pthread_create(&tid, NULL, &thread_func, NULL);
while(1)
{
// Check data.
printf("New data arrived: %d.\n", data);
sleep(2);
}
return 0;
}
但顯然主線程中的無限 while 循環是多余的。 所以我想這個怎么樣?
#include <time.h>
#include <stdio.h>
#include <pthread.h>
#include <time.h>
int data = 0;
pthread_mutex_t mtx;
void* thread_func(void* args)
{
while(1)
{
sleep(2);
// Data has appeared and can be read by main().
data = random() % 5;
pthread_mutex_unlock(&mtx);
}
return NULL;
}
int main()
{
int tid;
pthread_mutex_init(&mtx, NULL);
pthread_create(&tid, NULL, &thread_func, NULL);
while(1)
{
pthread_mutex_lock(&mtx);
printf("New data has arrived: %d.\n", data);
}
return 0;
}
這可行,但這是最好的方法嗎?
事實上,我不僅有一個主線程,還有幾個線程,我希望它們處於睡眠狀態,直到它們的新數據到達為止。 這將涉及為每個線程使用一個互斥鎖。 這是最好的做事方式嗎?
我希望這很清楚。 謝謝。
您可以使用pthread_cond_wait來等待您在線程之間共享的數據發生變化。 這個 function 會自動阻止你的互斥體,你必須在之后釋放它。 要通知您的線程數據已准備就緒,請使用pthread_cond_signal function。
但是要小心,您必須始終在每個線程中鎖定和解鎖互斥量,而不是像您的示例中那樣。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.