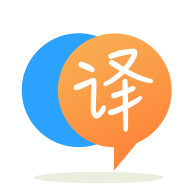
[英]How do I call a two-dimensional array created by a function outside of main?
[英]How do I properly call the function I created to the main?
所以我很討厭功能,需要調試它。 我很確定 function ToPigLating 在轉換方面做得很好。 但是,我只需要幫助在我的主要 function 內部調用 function ToPigLatin。 但是當我嘗試這樣做時,我只會得到一堆錯誤代碼。
#include <stdlib.h>
#include <string.h>
#define LEN 32
char* ToPigLatin(char* word[LEN]){
char word[LEN];
char translation [LEN];
char temp [LEN];
int i, j;
while ((scanf ("%s", word)) != '\0') {
strcpy (translation, word);
//just pretend I have all the work to convert it in here.
} // while
}
int main(){
printf("Enter 5 words: ");
scanf("%s", word);
ToPigLatin();
}```
粗略地說,變量只存在於聲明它們的 function 中。 ToPigLatin
中的word
只存在於ToPigLatin
中。 它在main
中不可用。 這讓我們編寫函數時不必擔心代碼的所有 rest。
您需要在main
中聲明一個不同的變量,它也可以稱為word
來存儲輸入,然后將其傳遞給ToPigLatin
。
讓我們用一些更簡單的東西來說明,一個 function,它的輸入加倍。
int times_two(int number) {
return number * 2;
}
我們需要給times_two
一個數字。
int main() {
// This is different from "number" in times_two.
int number = 42;
// We have to pass its value into time_two.
int doubled = times_two(number);
printf("%d doubled is %d\n", number, doubled);
}
您的情況有點復雜,因為您正在使用輸入和 memory 分配和 arrays。 我建議現在只關注 arrays 和 function 調用。 沒有scanf
。 沒有strcpy
。
例如,這里有一個 function 來打印一個單詞數組。
#include <stdio.h>
// Arrays in C don't store their size, the size must be given.
void printWords(const char *words[], size_t num_words) {
for( int i = 0; i < num_words; i++ ) {
printf("word[%d] is %s.\n", i, words[i]);
}
}
int main(){
// This "words" variable is distinct from the one in printWords.
const char *words[] = {"up", "down", "left", "right"};
// It must be passed into printWords along with its size.
printWords(words, 4);
}
ToPigLatingToPigLating function 期望有一個類似ToPigLating("MyParameter");
的參數
你好,icecolddash。
首先,缺少一些概念。 在主要部分:
scanf("%s", word);
您可能正在嘗試讀取字符串格式並存儲在 word 變量中。 在這種情況下,您應該在聲明 scope 中包含它。 經過一些調整后,它會是這樣的:
詮釋主要(){
字符字[LEN];
由於您定義的 LEN 最大為 32 個字節,因此您的程序將不允許讀取更大的字符串。 您還使用標准輸入和 output 函數作為 printf,因此您應該包含 stdio.h,這就是 header 已經聲明的“隱含聲明”的編譯警告。
下一個問題是你如何聲明你的翻譯 function,所以我們必須考慮一下:
char* ToPigLatin(char* word[LEN])
在這種情況下,您寫的是: ToPigLatin 是一個返回 char 指針的函數,這意味着您希望 function 可能返回一個字符串。 如果它對你有意義,那完全沒有問題。 雖然我們在參數 char* word[LEN] 方面遇到了一些實際問題。
像這樣聲明你的變量,假設你傳遞一個字符串數組作為參數。 如果我沒聽錯,你想閱讀正文中的所有五個單詞並翻譯每個單詞。 在這種情況下,我建議對主要的 function 進行一些更改,例如:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN 32
#define MAX_WORDS 5
char *globalname = "translated";
char* ToPigLatin(char* word){
char *translation = NULL;
//Translation work here.
if ( !strcmp(word, "icecolddash") ){
return NULL;
}
translation = globalname;
return translation;
}
int main(){
char word[LEN];
char *translatedword;
int i;
printf("Enter 5 words: \n");
for ( i=0; i < MAX_WORDS; i++ ){
fgets(word, sizeof(word), stdin);
strtok(word, "\n"); // Just in case you're using a keyboard as input.
translatedword = ToPigLatin(word);
if ( translatedword != NULL ){
//Do something with your translation
//I'll just print it out as an example
printf("%s\n", translatedword);
continue;
}
// Generic couldn't translate message
printf("Sorry, I know nothing about %s\n", word);
}
return 0;
}
上面的代碼將每個單詞翻譯成一個固定的單詞“translated”。 在讀取准確輸入“icecolddash”的情況下,程序將 output 出現一般錯誤消息,模擬翻譯過程中的一些問題。
我希望這對你的學習有所幫助。
我看到了幾件事。
#include <stdio.h>
#include <stdlib.h>
char* ToPigLatin(char* word){
printf(word);
return word;
}
int main(){
printf("Enter 5 words: ");
// declare the variable read into by scanf
char * word = NULL;
scanf("%s", word);
//Pass the variable into the function
ToPigLatin(word);
// Make sure you return an int from main()
return 0;
}
我在代碼中留下了一些帶有一些具體細節的注釋。
但是,我想強調的主要一點是您編寫代碼的方式。 始終嘗試編寫小的、可測試的塊並慢慢建立自己的方式。 嘗試讓您的代碼編譯。 總是。 如果你不能運行你的代碼,你就不能測試它來弄清楚你需要做什么。
至於你在劉易斯的帖子上留下的 char ** 評論,這里有一些你可能會發現對建立直覺有用的閱讀:
https://www.tutorialspoint.com/what-does-dereferencing-a-pointer-mean-in-c-cplusplus
快樂編碼!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.