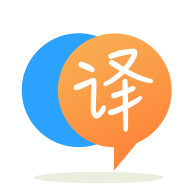
[英]Python: How to find a letter in a sentence's list of words and return those words in their original case (upper/lower)
[英]How to find all words with first letter as upper case using Python Regex
我需要在文件中找到所有以大寫字母開頭的單詞,我嘗試了下面的代碼,但它返回一個空字符串。
import os
import re
matches = []
filename = 'C://Users/Documents/romeo.txt'
with open(filename, 'r') as f:
for line in f:
regex = "^[A-Z]\w*$"
matches.append(re.findall(regex, line))
print(matches)
文件:
Hi, How are You?
Output:
[Hi,How,You]
您可以使用
import os, re
matches = []
filename = r'C:\Users\Documents\romeo.txt'
with open(filename, 'r') as f:
for line in f:
matches.extend([x for x in re.findall(r'\w+', line) if x[0].isupper()])
print(matches)
這個想法是使用簡單的\w+
正則表達式提取所有單詞,並僅將那些以大寫字母開頭的單詞添加到最終matches
列表中。
請參閱Python 演示。
注意:如果您只想匹配字母單詞,請使用r'\b[^\W\d_]+\b'
正則表達式。
這種方法對 Unicode 友好,即任何第一個大寫字母的 Unicode 字都會被找到。
你還問:
有沒有辦法將此限制為僅以大寫字母開頭的單詞而不是所有大寫單詞
您可以將前面的代碼擴展為
[x for x in re.findall(r'\w+', line) if x[0].isupper() and not x.isupper()]
請參閱此 Python 演示, "Hi, How ARE You?"
產生['Hi', 'How', 'You']
。
或者,為了避免在 output 中出現 CaMeL 字詞,請使用
matches.extend([x for x in re.findall(r'\w+', line) if x[0].isupper() and all(i.islower() for i in x[1:])])
請參閱此 Python 演示,其中all(i.islower() for i in x[1:])
確保第一個字母之后的所有字母都是小寫的。
完全正則表達式方法
您可以使用同時支持 Unicode 屬性和 POSIX 字符類\p{Lu}
/ \p{Ll}
和[:upper:]
/ [:lower:]
的 PyPi 正則表達式模塊。 所以,解決方案看起來像
import regex
text = "Hi, How ARE You?"
# Word starting with an uppercase letter:
print( regex.findall(r'\b\p{Lu}\p{L}*\b', text) )
## => ['Hi', 'How', 'ARE', 'You']
# Word starting with an uppercase letter but not ALLCAPS:
print( regex.findall(r'\b\p{Lu}\p{Ll}*\b', text) )
## => ['Hi', 'How', 'You']
\b
- 單詞邊界\p{Lu}
- 任何大寫字母\p{L}*
- 任何零個或多個字母\p{Ll}*
- 任何零個或多個小寫字母您可以使用單詞邊界代替錨^
和$
\b[A-Z]\w*
請注意,如果您使用matches.append
,則將項目添加到列表中並且 re.findall 返回列表,這將為您提供列表列表。
import re
matches = []
regex = r"\b[A-Z]\w*"
filename = r'C:\Users\Documents\romeo.txt'
with open(filename, 'r') as f:
for line in f:
matches += re.findall(regex, line)
print(matches)
Output
['Hi', 'How', 'You']
如果左邊應該有一個空白邊界,你也可以使用
(?<!\S)[A-Z]\w*
如果您不想使用\w
僅與大寫字符匹配單詞,則可以使用例如負前瞻來斷言不僅大寫字符直到單詞邊界
\b[A-Z](?![A-Z]*\b)\w*
\b
防止部分匹配的單詞邊界[AZ]
匹配大寫字符 AZ(?![AZ]*\b)
負前瞻,不僅斷言大寫字符后跟單詞邊界\w*
匹配可選的單詞字符要匹配以大寫字符開頭且不再包含任何大寫字符的單詞:
\b[A-Z][^\WA-Z]*\b
\b
一個詞的邊界[AZ]
匹配大寫字符 AZ[^\WA-Z]*
可選匹配一個不帶字符 AZ 的單詞 char\b
一個詞的邊界
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.