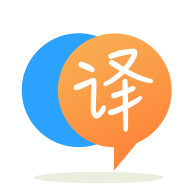
[英]How to format Highcharts columnRange to get json data for temperature Min and Max
[英]How to get the min, max, and sum of JSON data
我有 JSON 數據返回單個 int 值。 經過一些更改,這些值現在返回為整數的 arrays(以及原始格式)。
{
"value": 10,
"value": 70,
"value": 30,
"value": 200
}
- and -
{
"value": [64, 13, 55, 34, 52, 43, 59, 20, 20],
"value": [10, 90, 20, 80, 30, 70, 60, 40, 50]
}
我有一個公式可以返回舊版本 JSON 數據的最小值、最大值和總和。 現在它不起作用,我不知道重寫 function 以處理 arrays 的最佳方法是什么。 或者,如果它更好地制作第二個 function 來處理 arrays 並檢查它是 int 還是 array?
有沒有一種方法可以返回(從上面的數字):
// no value array, apply to all
[ 10, 200, 310 ] // min, max, sum
- and -
// for each of the value arrays
[ 23, 64, 360 ] // val 1 - min, max, sum
[ 10, 90, 450 ] // val 2 - min, max, sum
// input data const value = document.querySelectorAll( "div" ).forEach( el => { const contents = el.textContent, // get the text in the <div> json = JSON.parse( contents ), // parse the data jsonData = json.data; // get the data only // normalise the data // @from: https://stackoverflow.com/a/67294607/1086990 const normaliseData = arr => { const data = arr.map(({ value }) => value); return typeof arr[0].value === 'number'? [data]: data; }; // add into const const valueArray = normaliseData( jsonData ); // get the min / max / sum const minMaxSum = valueArray.forEach( e => { return [ Math.min(...e), Math.max(...e), [...e].reduce((v, w) => v + w) ]; }); // output console.log( minMaxSum ); });
<div> { "data": [ { "value": [64, 23, 45, 34, 52, 43, 59, 40] }, { "value": [10, 90, 20, 80, 30, 70, 60, 40, 50] } ] } </div> <div> { "data": [ { "value": 600 }, { "value": 70 }, { "value": 30 } ] } </div>
通過測試每個數組中第一個 object 的值的類型來規范化數據:
const valueInArray = [{ value: [64, 23] }, { value: [45, 34] }];
const valueAsSingle = [{ value: 600 }, { value: 70 }];
const normalizeData = arr => {
const data = arr.map(({ value }) => value);
return typeof arr[0].value === 'number'
? [data]
: data;
};
console.log(normalizeData(valueInArray));
//=> [ [ 64, 23 ], [ 45, 34 ] ]
console.log(normalizeData(valueAsSingle));
//=> [ [ 600, 70 ] ]
現在它們是相同的形狀,因此您可以平等對待它們。
您可以使用 Math.max 和 Math.min 找到數組的最大值和最小值,然后分配特定變量中的值。
目前,當您使用val.value
時,它由整個數組組成,因此您還需要遍歷數組以找到最大值、最小值或總和。
要找到總和,請在 val.value 數組上使用reduce
,然后將其添加到acc[2]
中。
// input data const valueInArray = document.getElementById("valueInArray").innerHTML, valueAsSingle = document.getElementById("valueAsSingle").innerHTML; // parse const jsonArray = JSON.parse( valueInArray ), jsonNumber = JSON.parse( valueAsSingle ), jsonArrayData = jsonArray.data, jsonNumberData = jsonNumber.data; // get numbers const minMaxSumArray = jsonArrayData.reduce( ( acc, val ) => { // smallest number acc[0] = ( ( acc[0] === undefined || Math.min(...val.value) < acc[0] )? Math.min(...val.value): acc[0] ) // largest number acc[1] = ( ( acc[1] === undefined || Math.max(...val.value) > acc[1] )? Math.max(...val.value): acc[1] ) // sum of numbers acc[2] = ( acc[2] === undefined? val.value.reduce((v, w) => v + w): val.value.reduce((v, w) => v + w) + acc[2] ) console.log('answer', acc) // return the array return acc; }, [] );
<div id="valueInArray"> { "data": [ { "value": [64, 23, 45, 34, 52, 43, 59, 40] }, { "value": [10, 90, 20, 80, 30, 70, 60, 40, 50] } ] } </div> <div id="valueAsSingle"> { "data": [ { "value": 10 }, { "value": 70 }, { "value": 30 } ] } </div>
我對此的看法:首先,通過連接它們並使用 Array.flat() 將其展平,創建一個包含所有值(arrays 或單個)的單個數組。 然后使用 reducer 確定總和並使用 Math.min/max 作為最小值和最大值。
// input data const valuesInArray = JSON.parse( document.querySelector("#valueInArray").textContent).data; const singleValues = JSON.parse( document.querySelector("#valueAsSingle").textContent).data; // get all values from the objects to a single Array of values // (so: convert all to single values) const allValues = valuesInArray.map( v => v.value ).concat(singleValues.reduce( (acc, val) => [...acc, +val.value], [] ) ).flat(); // let's see what we have console.log(`All values from both objects: ${JSON.stringify(allValues)}`); // create sum, min and max const [ sum, min, max, ] = [ allValues.reduce( (a, v) => a + +v, 0), Math.min(...allValues), Math.max(...allValues) ]; console.log(`From all values sum is ${sum}, min ${min} and max ${max}`);
div { display: none; }
<div id="valueInArray"> { "data": [ { "value": [64, 23, 45, 34, 52, 43, 59, 40] }, { "value": [10, 90, 20, 80, 30, 70, 60, 40, 50] } ] } </div> <div id="valueAsSingle"> { "data": [ { "value": 10 }, { "value": 70 }, { "value": 30 } ] } </div>
第二個片段聚合每個值的數據,其中單個值作為值數組添加到valuesInArray
。
// input data const valuesInArray = JSON.parse( document.querySelector("#valueInArray").textContent).data; const singleValues = JSON.parse( document.querySelector("#valueAsSingle").textContent).data; // create sum, min and max *per value*, in one go const aggregatesAdded = valuesInArray.concat({ value: singleValues.reduce( (acc, val) => [...acc, +val.value], [] ) } ).reduce( (acc, val) => [...acc, {...val, aggregatedValues: { sum: val.value.reduce( (a, v) => a + +v, 0 ), min: Math.min(...val.value), max: Math.max(...val.value) } } ], []) document.querySelector("pre").textContent = JSON.stringify({data: aggregatesAdded}, null, 2);
div { display: none; }
<div id="valueInArray"> { "data": [ { "value": [64, 23, 45, 34, 52, 43, 59, 40] }, { "value": [10, 90, 20, 80, 30, 70, 60, 40, 50] } ] } </div> <div id="valueAsSingle"> { "data": [ { "value": 10 }, { "value": 70 }, { "value": 30 } ] } </div> <pre id="result"></pre>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.