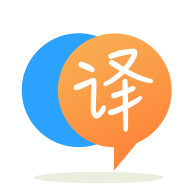
[英]Getting Java Play framework to cache Ebean entities using memcached
[英]Play Framework using AsyncCacheApi | Implement Cache for tests in Java
我目前正在嘗試在 Java 中實現 play.api.cache.AsyncCacheApi。 由於泛型參數,我在實現 get 和 getOrElseUpdate 時遇到了很大的麻煩。
我的 class:
package common;
import akka.Done;
import net.sf.ehcache.Element;
import play.api.cache.AsyncCacheApi;
import play.api.cache.SyncCacheApi;
import scala.Function0;
import scala.Option;
import scala.concurrent.Future;
import scala.concurrent.duration.Duration;
import scala.reflect.ClassTag;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import static scala.compat.java8.FutureConverters.toScala;
public class InMemoryCacheJava implements AsyncCacheApi {
final Map<String, Element> cache = new HashMap<>();
@Override
public SyncCacheApi sync() {
return null;
}
@Override
public Future<Done> set(String key, Object value, Duration expiration) {
return toScala(CompletableFuture.supplyAsync(
() -> {
final Element element = new Element(key, value);
if (expiration == Duration.Zero()) {
element.setEternal(true);
}
element.setTimeToLive(Math.toIntExact(expiration.toSeconds()));
cache.put(key, element);
return Done.getInstance();
}
));
}
@Override
public Future<Done> remove(String key) {
return toScala(CompletableFuture.supplyAsync(
() -> {
cache.remove(key);
return Done.getInstance();
}
));
}
@Override
public <T> Future<Option<T>> get(String key, ClassTag<T> evidence$2) {
return null;
}
@Override
public Future<Done> removeAll() {
return toScala(CompletableFuture.supplyAsync(
() -> {
cache.clear();
return Done.getInstance();
}
));
}
@Override
public <A> Future<A> getOrElseUpdate(String key, Duration expiration, Function0<Future<A>> orElse, ClassTag<A> evidence$1) {
return null;
}
}
這是正確的方法嗎? 我正在使用這個 API 因為在我的生產代碼中我正在使用實現 DefaultAsyncCacheApi 並且我想使用內部緩存(如地圖)測試我的代碼。 我錯過了什么?
我決定改用 Java API 並在 memory 緩存中實現了自己的。
我的工作代碼(它可能不是最好的實現),如果它可以幫助:
package common;
import akka.Done;
import play.cache.AsyncCacheApi;
import java.util.HashMap;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
public class InMemoryCache implements AsyncCacheApi {
private final HashMap<String, Object> cache = new HashMap();
@Override
public <T> CompletionStage<T> get(String key) {
return CompletableFuture.completedFuture((T) this.cache.get(key));
}
@Override
public <T> CompletionStage<T> getOrElseUpdate(String key, Callable<CompletionStage<T>> block, int expiration) {
return this.getOrElseUpdate(key, block);
}
@Override
public <T> CompletionStage<T> getOrElseUpdate(String key, Callable<CompletionStage<T>> block) {
final Object o = this.cache.get(key);
if (Objects.isNull(o)) {
try {
return block.call();
} catch (Exception e) {
e.printStackTrace();
}
}
return CompletableFuture.completedFuture((T) o);
}
@Override
public CompletionStage<Done> set(String key, Object value, int expiration) {
return this.set(key, value);
}
@Override
public CompletionStage<Done> set(String key, Object value) {
return CompletableFuture.supplyAsync(
() -> {
this.cache.put(key, value);
return Done.getInstance();
}
);
}
@Override
public CompletionStage<Done> remove(String key) {
return CompletableFuture.supplyAsync(
() -> {
this.cache.remove(key);
return Done.getInstance();
}
);
}
@Override
public CompletionStage<Done> removeAll() {
return CompletableFuture.supplyAsync(() -> {
this.cache.clear();
return Done.getInstance();
});
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.